如何在C语言中实现一个基于链表的单位员工通讯录管理系统?请详细说明链表节点的创建、信息的查询、修改、插入和删除过程。
时间: 2024-12-03 12:23:15 浏览: 22
在C语言中实现单位员工通讯录管理系统,涉及到线性表的多种操作。首先,我们需要定义一个链表节点的结构体,包含员工信息如编号、姓名、手机号码和办公室电话等,以及指向下一个节点的指针。链表的创建过程涉及初始化头节点并逐个添加新节点。信息查询需要遍历链表,比较每个节点的数据并返回匹配的记录。信息修改则是找到特定节点后更新其数据。信息插入涉及创建新节点并调整指针以将新节点加入到链表的适当位置。信息删除需要找到要删除的节点,并更新前一个节点的指针以跳过被删除节点,最后释放该节点的内存。整个过程需要良好的动态内存管理、指针操作能力以及对链表基本操作的熟悉。这个系统的设计不仅巩固了数据结构的基础知识,还提升了C语言的编程能力。
参考资源链接:[单位员工通讯录管理系统 - 数据结构线性表应用](https://wenku.csdn.net/doc/72ydmaof2b?spm=1055.2569.3001.10343)
相关问题
在C语言中,如何设计并实现一个基于单链表的员工通讯录管理系统?请详细说明如何添加员工信息、查询、修改和删除通讯录数据。
为了实现一个基于单链表的员工通讯录管理系统,你需要深入理解单链表的特性以及在C语言中的操作方法。首先,定义链表节点的数据结构,通常包括员工的基本信息和指向下一节点的指针。然后,实现链表的基本操作函数,如创建链表、添加节点、删除节点、查找节点、修改节点信息以及遍历链表等。以下是具体操作的示例代码:
参考资源链接:[单位员工通讯录管理系统 - 数据结构线性表应用](https://wenku.csdn.net/doc/7dhotf8fuo?spm=1055.2569.3001.10343)
首先,定义员工信息的结构体和链表节点结构体:
```c
typedef struct Employee {
int id;
char name[50];
char phone_number[15];
char office_phone[15];
struct Employee *next; // 指向下一个节点的指针
} EmployeeNode, *EmployeeLinkedList;
```
创建链表(初始化为NULL):
```c
EmployeeLinkedList createList() {
EmployeeLinkedList head = NULL;
return head;
}
```
插入节点到链表末尾:
```c
void insertNode(EmployeeLinkedList *head, EmployeeNode new_node) {
if (*head == NULL) {
*head = new_node;
} else {
EmployeeNode current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = new_node;
}
}
```
删除指定节点:
```c
void deleteNode(EmployeeLinkedList *head, int id) {
EmployeeNode current = *head;
EmployeeNode previous = NULL;
if (current != NULL && current->id == id) {
*head = current->next;
free(current);
return;
}
while (current != NULL && current->id != id) {
previous = current;
current = current->next;
}
if (current == NULL) {
return; // 没有找到
}
previous->next = current->next;
free(current);
}
```
查找节点(根据id):
```c
EmployeeNode *findNode(EmployeeLinkedList head, int id) {
EmployeeNode current = head;
while (current != NULL) {
if (current->id == id) {
return current;
}
current = current->next;
}
return NULL; // 没有找到
}
```
修改节点信息:
```c
void updateNode(EmployeeNode *node, const char *new_name, const char *new_phone, const char *new_office_phone) {
if (node != NULL) {
strcpy(node->name, new_name);
strcpy(node->phone_number, new_phone);
strcpy(node->office_phone, new_office_phone);
}
}
```
遍历链表并打印所有员工信息:
```c
void traverseList(EmployeeLinkedList head) {
EmployeeNode *current = head;
while (current != NULL) {
printf(
参考资源链接:[单位员工通讯录管理系统 - 数据结构线性表应用](https://wenku.csdn.net/doc/7dhotf8fuo?spm=1055.2569.3001.10343)
如何在C语言中使用单链表实现一个高效的员工通讯录管理系统?请提供创建链表、插入节点、删除节点和遍历链表的示例代码。
在C语言中,使用单链表来实现一个员工通讯录管理系统是数据结构应用的一个经典案例。为了帮助你构建这样的系统,下面将提供创建链表、插入节点、删除节点和遍历链表的示例代码。
参考资源链接:[单位员工通讯录管理系统 - 数据结构线性表应用](https://wenku.csdn.net/doc/7dhotf8fuo?spm=1055.2569.3001.10343)
首先,定义员工信息的节点结构体和链表的头指针:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义员工信息的结构体
typedef struct Employee {
int id; // 员工编号
char name[50]; // 员工姓名
char phone[20]; // 手机号码
char officePhone[20]; // 办公室电话
struct Employee *next; // 指向下一个员工信息的指针
} Employee;
// 创建链表头指针
Employee *createList() {
return NULL;
}
```
接下来,实现插入节点的功能,将新的员工信息添加到链表中:
```c
Employee *insert(Employee *head, int id, const char *name, const char *phone, const char *officePhone) {
// 创建新节点
Employee *newNode = (Employee *)malloc(sizeof(Employee));
if (!newNode) {
printf(
参考资源链接:[单位员工通讯录管理系统 - 数据结构线性表应用](https://wenku.csdn.net/doc/7dhotf8fuo?spm=1055.2569.3001.10343)
阅读全文
相关推荐
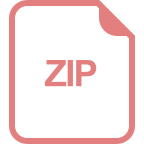
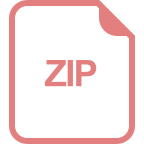
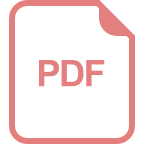



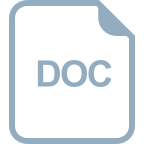
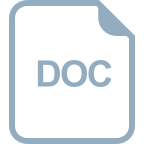







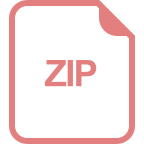
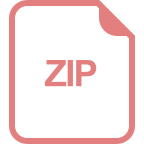