[MarshalAs(UnmanagedType.ByValArray, SizeConst = 1)] 这行代码是什么意思?
时间: 2024-04-18 12:26:29 浏览: 129
这行代码是用于指定参数在托管和非托管代码之间进行互操作时的数据传递方式。
`MarshalAs(UnmanagedType.ByValArray, SizeConst = 1)` 表示将参数作为按值传递的数组传递,并指定数组的大小为1。
具体解释如下:
- `MarshalAs(UnmanagedType.ByValArray)` 指定参数以按值传递的方式进行传递。这意味着参数的值会被复制到非托管代码中,而不是通过引用传递。
- `SizeConst = 1` 指定数组的大小为1。这表示参数被当作一个仅包含一个元素的数组,即使在实际使用时该参数可能是一个单个的元素而不是数组。
使用 `MarshalAs` 属性可以在托管和非托管代码之间传递不同类型的数据,例如结构体、字符串、数组等。通过指定适当的 `MarshalAs` 参数,可以确保数据在传递过程中被正确地转换和处理。
需要注意的是,该代码片段可能是在与非托管代码进行交互或者进行平台调用时使用的,因此更多的上下文信息可能有助于准确理解其含义。
希望对你有所帮助。如果还有其他问题,请随时提问。
相关问题
生成USBCAN的代码
USBCAN 是一种 USB 接口的 CAN 总线适配器,可以用于与汽车电子控制模块(ECU)进行通信。在 C# 中生成 USBCAN 的代码可以通过以下步骤进行:
1. 引用 USBCAN 动态链接库:在 C# 项目中添加对 USBCAN 动态链接库的引用,可以使用 DLLImport 来加载动态链接库,并定义相应的函数和结构体。
```csharp
using System;
using System.Runtime.InteropServices;
namespace USBCANExample
{
class Program
{
// 加载动态链接库
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_OpenDevice(UInt32 DeviceType, UInt32 DeviceIndex, UInt32 Reserved);
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_CloseDevice(UInt32 DeviceType, UInt32 DeviceIndex);
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_InitCAN(UInt32 DeviceType, UInt32 DeviceIndex, UInt32 CANIndex, ref VCI_INIT_CONFIG pInitConfig);
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_StartCAN(UInt32 DeviceType, UInt32 DeviceIndex, UInt32 CANIndex);
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_Transmit(UInt32 DeviceType, UInt32 DeviceIndex, UInt32 CANIndex, ref VCI_CAN_OBJ pSend, UInt32 Length);
[DllImport("ControlCAN.dll")]
static extern UInt32 VCI_Receive(UInt32 DeviceType, UInt32 DeviceIndex, UInt32 CANIndex, [Out] VCI_CAN_OBJ[] pReceive, UInt32 Length, UInt32 WaitTime);
}
// CAN 初始化参数结构体
[StructLayout(LayoutKind.Sequential)]
public struct VCI_INIT_CONFIG
{
public UInt32 AccCode;
public UInt32 AccMask;
public UInt32 Reserved;
public Byte Filter;
public Byte Timing0;
public Byte Timing1;
public Byte Mode;
}
// CAN 数据帧结构体
[StructLayout(LayoutKind.Sequential)]
public struct VCI_CAN_OBJ
{
public UInt32 ID;
public UInt32 TimeStamp;
public Byte TimeFlag;
public Byte SendType;
public Byte RemoteFlag;
public Byte ExternFlag;
public Byte DataLen;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 8)]
public Byte[] Data;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 3)]
public Byte[] Reserved;
}
}
```
2. 初始化 USBCAN 适配器:在 C# 代码中调用 VCI_OpenDevice 和 VCI_InitCAN 函数来打开设备和初始化 CAN 总线参数。
```csharp
// 初始化 USBCAN 适配器
UInt32 DeviceType = 4; // USBCAN2
UInt32 DeviceIndex = 0;
UInt32 CANIndex = 0;
VCI_INIT_CONFIG InitConfig = new VCI_INIT_CONFIG();
InitConfig.AccCode = 0x00000000;
InitConfig.AccMask = 0xFFFFFFFF;
InitConfig.Filter = 0;
InitConfig.Mode = 0;
InitConfig.Timing0 = 0x00;
InitConfig.Timing1 = 0x1C;
UInt32 status = VCI_OpenDevice(DeviceType, DeviceIndex, 0);
status = VCI_InitCAN(DeviceType, DeviceIndex, CANIndex, ref InitConfig);
status = VCI_StartCAN(DeviceType, DeviceIndex, CANIndex);
```
3. 发送和接收 CAN 数据帧:在 C# 代码中调用 VCI_Transmit 和 VCI_Receive 函数来发送和接收 CAN 数据帧。
```csharp
// 发送 CAN 数据帧
VCI_CAN_OBJ send = new VCI_CAN_OBJ();
send.ID = 0x123;
send.DataLen = 8;
send.Data = new byte[] { 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08 };
status = VCI_Transmit(DeviceType, DeviceIndex, CANIndex, ref send, 1);
// 接收 CAN 数据帧
VCI_CAN_OBJ[] receive = new VCI_CAN_OBJ[1024];
status = VCI_Receive(DeviceType, DeviceIndex, CANIndex, receive, 1024, 1000);
if (status > 0)
{
for (int i = 0; i < status; i++)
{
Console.WriteLine("ID: 0x{0:X}, Data: {1}", receive[i].ID, BitConverter.ToString(receive[i].Data));
}
}
```
需要注意的是,USBCAN 的使用和配置需要根据具体的硬件和软件环境进行。以上代码示例仅供参考,具体实现需要根据实际需求进行修改和完善。
C#代码如何实现海康相机数字IO输出
以下是C#代码示例,用于实现海康相机数字IO输出:
```c#
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Runtime.InteropServices;
namespace DigitalIOControlDemo
{
class Program
{
[DllImport("HCNetSDK.dll")]
public static extern bool NET_DVR_Init();
[DllImport("HCNetSDK.dll")]
public static extern bool NET_DVR_Cleanup();
[DllImport("HCNetSDK.dll")]
public static extern int NET_DVR_Login_V30(string sDVRIP, ushort wDVRPort, string sUserName, string sPassword, ref NET_DVR_DEVICEINFO_V30 lpDeviceInfo);
[DllImport("HCNetSDK.dll")]
public static extern bool NET_DVR_Logout(int lUserID);
[DllImport("HCNetSDK.dll")]
public static extern bool NET_DVR_SetDVRConfig(int lUserID, uint dwCommand, int lChannel, ref NET_DVR_DIGITAL_IO_CONTROL lpInBuffer, uint dwInBufferSize);
[StructLayout(LayoutKind.Sequential)]
public struct NET_DVR_DEVICEINFO_V30
{
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 48)]
public string sSerialNumber;
public byte byAlarmInPortNum;
public byte byAlarmOutPortNum;
public byte byDiskNum;
public byte byDVRType;
public byte byChanNum;
public byte byStartChan;
public byte byAudioChanNum;
public byte byIPChanNum;
public byte byZeroChanNum;
public byte byMainProto;
public byte bySubProto;
public byte bySupport;
public byte bySupport1;
public byte bySupport2;
public ushort wDevType;
public byte bySupport3;
public byte byMultiStreamProto;
public byte byStartDChan;
public byte byStartDTalkChan;
public byte byHighDChanNum;
public byte bySupport4;
public byte byLanguageType;
public byte byVoiceInChanNum;
public byte byRes3;
public byte byMirrorChanNum;
public ushort wStartMirrorChanNo;
public byte byRes2;
public byte bySupport5;
public int lStartUserRight; // the begin user right
public int lEndUserRight; // the end user right
public byte byMirrorChanNum2;
public byte byStartListenChan;
public byte byReserve3;
public byte bySupport6;
public int lSerialNum; //Serial number
public byte bySupport7;
public byte byDevTypeName;
public byte byRes4;
public byte bySupport8;
public byte bySupport9;
public byte bySupport10;
public byte bySupport11;
public byte bySupport12;
public byte bySupport13;
public byte bySupport14;
public byte bySupport15;
public byte bySupport16;
public byte bySupport17;
public byte bySupport18;
public byte bySupport19;
public byte bySupport20;
public byte bySupport21;
public byte bySupport22;
public byte bySupport23;
public byte bySupport24;
public byte bySupport25;
public byte bySupport26;
public byte bySupport27;
public byte bySupport28;
public byte bySupport29;
public byte bySupport30;
public byte bySupport31;
public byte bySupport32;
public byte bySupport33;
public byte bySupport34;
public byte bySupport35;
public byte bySupport36;
public byte bySupport37;
public byte bySupport38;
public byte bySupport39;
public byte bySupport40;
public byte bySupport41;
public byte bySupport42;
public byte bySupport43;
public byte bySupport44;
public byte bySupport45;
public byte bySupport46;
public byte bySupport47;
public byte bySupport48;
public byte bySupport49;
public byte bySupport50;
public byte bySupport51;
public byte bySupport52;
public byte bySupport53;
public byte bySupport54;
public byte bySupport55;
public byte bySupport56;
public byte bySupport57;
public byte bySupport58;
public byte bySupport59;
public byte bySupport60;
public byte bySupport61;
public byte bySupport62;
public byte bySupport63;
public byte bySupport64;
}
[StructLayout(LayoutKind.Sequential)]
public struct NET_DVR_DIGITAL_IO_CONTROL
{
public uint dwSize;
public uint dwChannel;
public uint dwControlType;
public NET_DVR_DIGITAL_IO struDigitalIO;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 76, ArraySubType = UnmanagedType.I1)]
public byte[] byRes;
}
[StructLayout(LayoutKind.Sequential)]
public struct NET_DVR_DIGITAL_IO
{
public uint dwChannel;
public uint dwWorkType;
public uint dwTrigType;
public byte byDelayTime;
public byte byRes1;
public ushort wRes1;
public uint dwAlarmOutPut;
public uint dwOutputDuration;
public byte byMode;
public byte byPolarity;
public byte byRes2;
public byte bySignalLatch;
public byte byRes3;
public byte bySignalType;
public byte byRes4;
public byte byRes5;
public byte byRes6;
public byte byRes7;
public uint dwSignalInPut;
public uint dwInputLevel;
public byte byRes8;
public byte byRes9;
public ushort wRes2;
public uint dwTrigChannel;
public byte byTrigDelayTime;
public byte byTrigLatch;
public byte byTrigSignalType;
public byte byRes10;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 4, ArraySubType = UnmanagedType.I1)]
public byte[] byRes11;
}
static void Main(string[] args)
{
// 初始化 SDK
NET_DVR_Init();
// 登录相机
NET_DVR_DEVICEINFO_V30 deviceInfo = new NET_DVR_DEVICEINFO_V30();
int lUserID = NET_DVR_Login_V30("192.168.1.64", 8000, "admin", "12345", ref deviceInfo);
if (lUserID < 0)
{
Console.WriteLine("登录相机失败,错误码:" + NET_DVR_GetLastError().ToString());
return;
}
// 设置数字 IO 参数
NET_DVR_DIGITAL_IO_CONTROL struIOControl = new NET_DVR_DIGITAL_IO_CONTROL();
struIOControl.dwSize = (uint)Marshal.SizeOf(struIOControl);
struIOControl.dwChannel = 1; // IO 通道号
struIOControl.dwControlType = 0; // 输出模式
NET_DVR_SetDVRConfig(lUserID, NET_DVR_SET_IO_CONTROL_CFG, 0, ref struIOControl, (uint)Marshal.SizeOf(struIOControl));
// 启用输出信号
struIOControl.struDigitalIO.dwChannel = 1; // IO 通道号
struIOControl.struDigitalIO.dwWorkType = 1; // 手动模式
struIOControl.struDigitalIO.byMode = 1; // 输出高电平
NET_DVR_SetDVRConfig(lUserID, NET_DVR_SET_IO_CONTROL_CFG, 0, ref struIOControl, (uint)Marshal.SizeOf(struIOControl));
// 关闭输出信号
struIOControl.struDigitalIO.byMode = 0; // 输出低电平
NET_DVR_SetDVRConfig(lUserID, NET_DVR_SET_IO_CONTROL_CFG, 0, ref struIOControl, (uint)Marshal.SizeOf(struIOControl));
// 释放资源
NET_DVR_Logout(lUserID);
NET_DVR_Cleanup();
}
}
}
```
注意:以上代码仅供参考,实际使用时需要根据相机型号和需求进行调整。
阅读全文
相关推荐
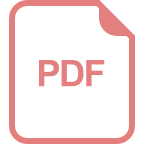
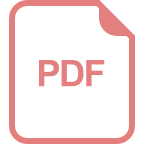
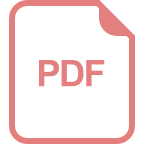
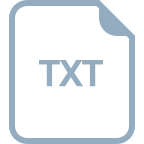
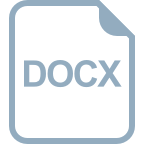
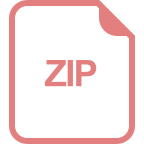
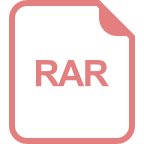
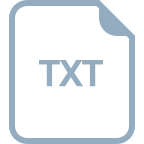





