C++邻接表存储有向图求两点间是否存在路径
时间: 2023-08-10 09:02:12 浏览: 212
可以使用深度优先搜索(DFS)或广度优先搜索(BFS)来判断两点之间是否存在路径。以下是使用邻接表存储有向图,使用DFS判断两点之间是否存在路径的示例代码:
```c++
#include <iostream>
#include <vector>
using namespace std;
const int MAXN = 100;
vector<int> g[MAXN]; // 邻接表存储图
bool vis[MAXN]; // 标记节点是否被访问过
void dfs(int u, int v, bool& found) {
if (u == v) { // 找到了一条路径
found = true;
return;
}
vis[u] = true;
for (int i = 0; i < g[u].size(); i++) {
int nxt = g[u][i];
if (!vis[nxt]) { // 没有访问过的节点才需要继续遍历
dfs(nxt, v, found);
if (found) return; // 如果找到路径了,直接返回
}
}
}
int main() {
int n, m;
cin >> n >> m;
for (int i = 0; i < m; i++) {
int u, v;
cin >> u >> v;
g[u].push_back(v);
}
int s, t;
cin >> s >> t;
bool found = false;
dfs(s, t, found);
if (found) cout << "Exists a path from " << s << " to " << t << endl;
else cout << "No path exists from " << s << " to " << t << endl;
return 0;
}
```
在上述代码中,我们使用了一个`vis`数组来标记节点是否被访问过。在DFS函数中,我们首先判断是否已经找到了一条路径,如果是,则直接返回。然后,我们将起始节点`u`标记为已访问,并遍历其所有未被访问过的邻居节点。对于每个邻居节点,如果它没有被访问过,则继续递归搜索。如果找到了一条路径,则通过`found`变量来标记,并直接返回。如果搜索完所有邻居节点都没有找到路径,则返回上一层搜索。
你也可以使用BFS来实现,思路类似,只是用一个队列来存储待访问的节点。
阅读全文
相关推荐
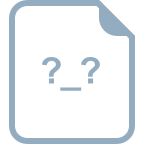


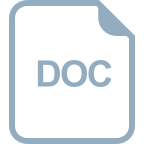
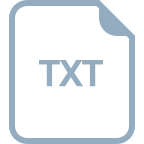
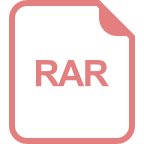
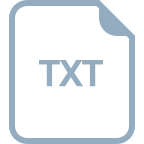
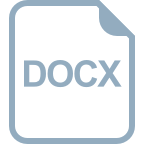
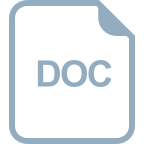
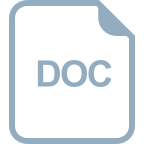
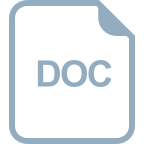
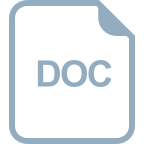
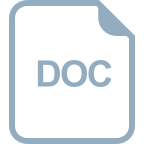




