LSTM自编码器pytorh实现
时间: 2024-04-24 14:15:22 浏览: 63
好的,以下是一个简单的LSTM自编码器的PyTorch实现,希望可以帮助你:
```python
import torch
import torch.nn as nn
class LSTM_Autoencoder(nn.Module):
def __init__(self, input_dim, hidden_dim, num_layers, device):
super(LSTM_Autoencoder, self).__init__()
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.num_layers = num_layers
self.device = device
# Encoder
self.encoder_lstm = nn.LSTM(input_dim, hidden_dim, num_layers, batch_first=True)
self.encoder_fc = nn.Linear(hidden_dim, hidden_dim)
# Decoder
self.decoder_lstm = nn.LSTM(hidden_dim, input_dim, num_layers, batch_first=True)
def encode(self, x):
# Set initial hidden and cell states
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_dim).to(self.device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_dim).to(self.device)
# Forward propagate LSTM
out, (hidden, cell) = self.encoder_lstm(x, (h0, c0))
# Apply FC layer to the final hidden state
out = self.encoder_fc(hidden[-1])
return out
def decode(self, x):
# Set initial hidden and cell states
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_dim).to(self.device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_dim).to(self.device)
# Forward propagate LSTM
out, (hidden, cell) = self.decoder_lstm(x, (h0, c0))
return out
def forward(self, x):
# Encode
encoded = self.encode(x)
# Decode
decoded = self.decode(encoded.unsqueeze(1))
return decoded.squeeze(1)
```
在这个实现中,我们定义了一个 `LSTM_Autoencoder` 类,它包括一个编码器和一个解码器。编码器由一个LSTM层和一个全连接层组成,解码器由一个LSTM层组成。在 `encode` 方法中,我们将输入 `x` 通过编码器传递,并返回最终的隐藏状态。在 `decode` 方法中,我们将编码器输出的隐藏状态传递给解码器,并返回解码器的输出。在 `forward` 方法中,我们首先将输入 `x` 编码为隐藏状态,然后将该隐藏状态解码为重构的输出。请注意,我们在 `decode` 方法中使用了 `unsqueeze(1)` 来为LSTM层添加一个时间步维度,并在 `forward` 方法中使用了 `squeeze(1)` 将其删除。
现在,我们可以使用这个自编码器来对数据进行编码和解码。以下是一个简单的例子:
```python
# 超参数
input_dim = 10
hidden_dim = 5
num_layers = 2
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
# 创建自编码器
autoencoder = LSTM_Autoencoder(input_dim, hidden_dim, num_layers, device).to(device)
# 创建随机数据
x = torch.randn(32, 10).to(device)
# 前向传递
encoded = autoencoder.encode(x)
decoded = autoencoder.decode(encoded.unsqueeze(1))
# 计算重构误差
loss_fn = nn.MSELoss()
loss = loss_fn(decoded.squeeze(1), x)
print('Reconstruction loss: {:.4f}'.format(loss.item()))
```
在这个例子中,我们首先使用 `LSTM_Autoencoder` 类创建了一个自编码器。然后我们创建了一个随机的张量 `x`,并将其传递到自编码器中进行编码和解码。最后,我们计算了重构误差,并将其打印出来。
希望这个例子对你有所帮助!
阅读全文
相关推荐
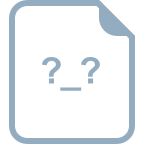


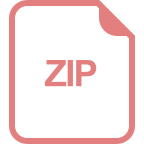
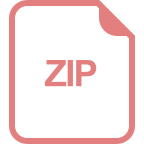
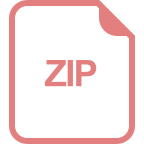
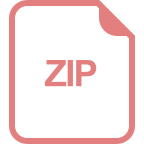
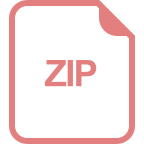
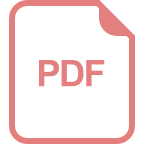
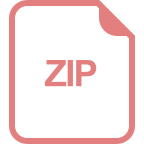
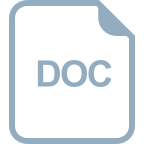
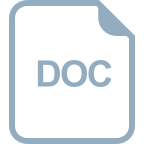




