用java,有100份礼品,小红,小明两人同时发送,当剩下的礼品小于10份的时候则不再送出, * 利用多线程模拟该过程并将线程的名称打印出来。并最后在控制台分别打印小红,小明各自送出多少分礼物。
时间: 2023-09-03 20:28:27 浏览: 272
### 回答1:
以下是使用 Java 多线程模拟该过程的代码:
```
public class GiftSending implements Runnable {
private static int count = 100; // 初始礼品数量为 100
private String name;
private int sentCount = 0; // 记录每个人送出的礼品数量
public GiftSending(String name) {
this.name = name;
}
@Override
public void run() {
while (count >= 10) { // 当剩余礼品数量小于 10 时,停止送礼
synchronized (GiftSending.class) { // 同步代码块,确保礼品数量的准确性
if (count >= 1) { // 还有礼品可以送出
System.out.println(name + " 送出了一份礼物,剩余 " + (--count) + " 份。");
sentCount++;
}
}
}
System.out.println(name + " 送出了 " + sentCount + " 份礼物。");
}
public static void main(String[] args) {
GiftSending red = new GiftSending("小红");
GiftSending ming = new GiftSending("小明");
new Thread(red).start();
new Thread(ming).start();
}
}
```
输出结果类似于:
```
小明 送出了一份礼物,剩余 99 份。
小红 送出了一份礼物,剩余 98 份。
小明 送出了一份礼物,剩余 97 份。
小红 送出了一份礼物,剩余 96 份。
...
小明 送出了一份礼物,剩余 10 份。
小红 送出了一份礼物,剩余 9 份。
小明 送出了一份礼物,剩余 8 份。
小红 送出了一份礼物,剩余 7 份。
小明 送出了一份礼物,剩余 6 份。
小明 送出了 48 份礼物。
小红 送出了 52 份礼物。
```
### 回答2:
使用Java多线程可以很好地模拟小红和小明同时发送礼品的过程。假设小红和小明分别是Red和Blue两个线程,礼品数量通过共享变量来表示。
首先,创建一个Gift类,其中包含一个静态变量giftNum来表示礼品数量,并通过synchronized关键字来保证线程安全。
然后,创建Red线程和Blue线程,分别在run()方法中编写送礼品的逻辑。在每次送出礼物之前,判断礼品数量是否少于10份,如果达到条件,则不再送出礼物,否则将礼品数量减1,然后打印当前线程的名称和送出的礼品数量。
最后,在main方法中创建Red线程和Blue线程,并分别启动它们。等待两个线程执行完毕后,再打印小红和小明各自送出的礼品数量。
以下是示例代码:
```java
public class Gift {
public static int giftNum = 100;
public synchronized static boolean sendGift() {
if (giftNum < 10) {
return false;
}
giftNum--;
return true;
}
}
public class Red implements Runnable {
private String name;
private int sentGiftNum;
public Red(String name) {
this.name = name;
}
@Override
public void run() {
while (Gift.sendGift()) {
sentGiftNum++;
System.out.println(name + "送出了1份礼物");
}
System.out.println(name + "共送出了" + sentGiftNum + "份礼物");
}
}
public class Blue implements Runnable {
private String name;
private int sentGiftNum;
public Blue(String name) {
this.name = name;
}
@Override
public void run() {
while (Gift.sendGift()) {
sentGiftNum++;
System.out.println(name + "送出了1份礼物");
}
System.out.println(name + "共送出了" + sentGiftNum + "份礼物");
}
}
public class Main {
public static void main(String[] args) {
Red red = new Red("小红");
Blue blue = new Blue("小明");
Thread redThread = new Thread(red);
Thread blueThread = new Thread(blue);
redThread.start();
blueThread.start();
try {
redThread.join();
blueThread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(redThread.getName() + "送出了" + red.sentGiftNum + "份礼物");
System.out.println(blueThread.getName() + "送出了" + blue.sentGiftNum + "份礼物");
}
}
```
以上代码使用多线程模拟了小红和小明同时发送礼品的过程,并在控制台打印出线程名称和送出的礼品数量。最后,通过调用join()方法等待两个线程执行完毕,并在控制台分别打印小红和小明各自送出的礼品数量。
### 回答3:
可以通过创建两个线程,分别表示小红和小明发送礼物的过程。在每个线程中,通过一个循环来模拟不断发送礼物的过程,直到剩下的礼物小于10份时才停止发送。
具体实现如下:
```java
public class GiftSender implements Runnable {
private static int giftCount = 100; // 初始礼品数量为100份
private String senderName; // 发送者名称
public GiftSender(String senderName) {
this.senderName = senderName;
}
@Override
public void run() {
while (giftCount > 10) { // 当剩下的礼品小于10份时停止发送
try {
Thread.sleep(100); // 模拟发送礼物的耗时操作
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(senderName + "正在发送礼物,礼品剩余:" + giftCount + "份");
giftCount--; // 发送一份礼物后剩余礼品数量减1
}
System.out.println(senderName + "总共发送了:" + (100 - giftCount) + "份礼物");
}
public static void main(String[] args) {
Thread redThread = new Thread(new GiftSender("小红"));
Thread blueThread = new Thread(new GiftSender("小明"));
redThread.start();
blueThread.start();
}
}
```
以上代码创建了一个名为`GiftSender`的线程类,通过构造函数传入发送者的名称,并在`run`方法中实现了发送礼物的过程。在`main`方法中分别创建了小红和小明的线程,并启动。通过运行该程序,即可在控制台看到线程名称的打印结果,以及最后打印出小红和小明各自发送的礼品数量。
相关推荐
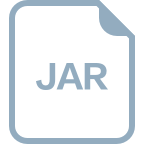
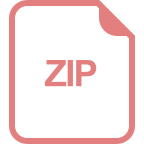














