使用python对2维图像采用最邻近插值和双线性插值算法实现旋转 ,不使用OpenCv和pillow库
时间: 2023-06-13 18:02:46 浏览: 201
旋转2维图像可以使用最邻近插值和双线性插值算法。这里给出基于 NumPy 和 Matplotlib 的实现代码,具体如下:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义最邻近插值函数
def nearest_interp(image, x, y):
height, width = image.shape
x = np.round(x).astype(int)
y = np.round(y).astype(int)
x = np.minimum(np.maximum(x, 0), width - 1)
y = np.minimum(np.maximum(y, 0), height - 1)
return image[y, x]
# 定义双线性插值函数
def bilinear_interp(image, x, y):
height, width = image.shape
x0 = np.floor(x).astype(int)
y0 = np.floor(y).astype(int)
x1 = np.minimum(x0 + 1, width - 1)
y1 = np.minimum(y0 + 1, height - 1)
f00 = image[y0, x0]
f01 = image[y0, x1]
f10 = image[y1, x0]
f11 = image[y1, x1]
wx = x - x0
wy = y - y0
fx0 = f00 * (1 - wx) + f01 * wx
fx1 = f10 * (1 - wx) + f11 * wx
result = fx0 * (1 - wy) + fx1 * wy
return result
# 定义旋转函数
def rotate(image, angle, interp_func):
height, width = image.shape
center_x = width / 2
center_y = height / 2
angle = np.deg2rad(angle)
cos_a = np.cos(angle)
sin_a = np.sin(angle)
x, y = np.meshgrid(np.arange(width), np.arange(height))
x = x - center_x
y = y - center_y
new_x = x * cos_a + y * sin_a
new_y = -x * sin_a + y * cos_a
new_x = new_x + center_x
new_y = new_y + center_y
result = interp_func(image, new_x, new_y)
return result
# 读取图像
image = plt.imread('test.png')
# 最邻近插值旋转
result_nearest = rotate(image, 45, nearest_interp)
# 双线性插值旋转
result_bilinear = rotate(image, 45, bilinear_interp)
# 显示结果
plt.subplot(1, 3, 1)
plt.imshow(image)
plt.title('Original')
plt.axis('off')
plt.subplot(1, 3, 2)
plt.imshow(result_nearest)
plt.title('Nearest')
plt.axis('off')
plt.subplot(1, 3, 3)
plt.imshow(result_bilinear)
plt.title('Bilinear')
plt.axis('off')
plt.show()
```
上述代码中,`nearest_interp` 和 `bilinear_interp` 分别实现了最邻近插值和双线性插值算法。`rotate` 函数实现了对图像的旋转,其中 `interp_func` 参数可以传入不同的插值函数。最后通过 Matplotlib 的 `imshow` 函数展示了原始图像、最邻近插值旋转和双线性插值旋转的结果。
阅读全文
相关推荐
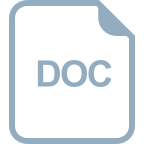
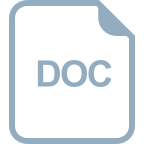
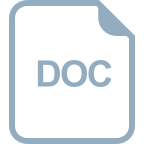
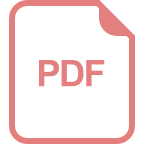
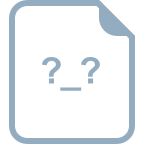
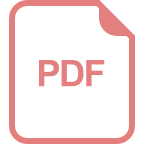
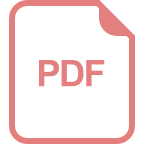










