c语言统计代码中关键字个数
时间: 2024-06-12 22:08:26 浏览: 115
关键字是在编程语言中具有特殊含义和功能的预定义单词。在C语言中,关键字是被保留的,不能作为标识符使用。下面是一个示例代码,可以统计C语言代码中关键字的数量:
```c
#include <stdio.h>
#include <string.h>
int isKeyword(char* word) {
char* keywords[] = {
"auto", "break", "case", "char", "const", "continue", "default", "do",
"double", "else", "enum", "extern", "float", "for", "goto", "if",
"int", "long", "register", "return", "short", "signed", "sizeof",
"static", "struct", "switch", "typedef", "union", "unsigned",
"void", "volatile", "while"
};
int numKeywords = sizeof(keywords) / sizeof(keywords[0]);
for (int i = 0; i < numKeywords; i++) {
if (strcmp(word, keywords[i]) == 0) {
return 1;
}
}
return 0;
}
int countKeywords(char* code) {
int count = 0;
char* word = strtok(code, " \t\n\r");
while (word != NULL) {
if (isKeyword(word)) {
count++;
}
word = strtok(NULL, " \t\n\r");
}
return count;
}
int main() {
char code[] = "int main() {\n\tint a = 10;\n\treturn 0;\n}";
int numKeywords = countKeywords(code);
printf("Number of keywords: %d\n", numKeywords);
return 0;
}
```
该示例代码中,`isKeyword` 函数用于判断一个单词是否是关键字,`countKeywords` 函数用于统计代码中关键字的个数。在 `main` 函数中,我们给出了一个示例代码,其中包含了一些C语言关键字。运行该代码,将会输出关键字的数量。
阅读全文
相关推荐




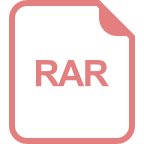
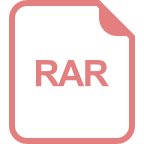












