#!/usr/bin/env python3 # -*- coding: utf-8 -*- import rospy import math import numpy as np from grasp_demo.srv import * def tf_transform(req): tool_h_cam = np.array([[1.0, 0.0, 0, -0.010], [0.0, -1.0, 0.0, -0.018], [0.0, 0.0, -1.0, 0.1], [0.0, 0.0, 0.0, 1.0] ]) cam_h_obj = np.array([[req.marker_x], [req.marker_y], [req.marker_z], [1]]) r_x = np.array([[1, 0, 0], [0, math.cos(req.robot_roll), -math.sin(req.robot_roll)], [0, math.sin(req.robot_roll), math.cos(req.robot_roll)] ]) r_y = np.array([[math.cos(req.robot_pitch), 0, math.sin(req.robot_pitch)], [0, 1, 0], [-math.sin(req.robot_pitch), 0, math.cos(req.robot_pitch)] ]) r_z = np.array([[math.cos(req.robot_yaw), -math.sin(req.robot_yaw), 0], [math.sin(req.robot_yaw), math.cos(req.robot_yaw), 0], [0, 0, 1] ]) r = np.dot(r_z, np.dot(r_y, r_x)) base_h_tool = np.array([[r[0, 0], r[0, 1], r[0, 2], req.robot_x], [r[1, 0], r[1, 1], r[1, 2], req.robot_y], [r[2, 0], r[2, 1], r[2, 2], req.robot_z], [0, 0, 0, 1] ]) print(base_h_tool) kk = np.dot(base_h_tool, tool_h_cam) #print (kk) base_h_obj = np.dot(np.dot(base_h_tool, tool_h_cam), cam_h_obj) return EyeinHandResponse(base_h_obj[0, 0], base_h_obj[1, 0], base_h_obj[2, 0]) def obj_to_base_server(): rospy.init_node('eyeinhandServer') s = rospy.Service('eyeinhand', EyeinHand, tf_transform) rospy.spin() if __name__ == "__main__": obj_to_base_server()
时间: 2024-02-29 16:57:10 浏览: 20
这是一个 Python 节点,使用了 ROS 框架,提供了一个名为 'eyeinhand' 的服务。该服务的请求消息类型为 EyeinHandRequest,响应消息类型为 EyeinHandResponse。在这个节点中,定义了一个名为 tf_transform 的函数,用于处理服务的请求。这个函数接收一个请求,其中包含机器人的位姿信息和相机标定信息,并计算出相机到目标物体的位姿信息。最后,函数返回一个响应,其中包含了目标物体在机器人坐标系下的位姿信息。这个节点的主函数是 obj_to_base_server(),它初始化了 ROS 节点,并将 'eyeinhand' 服务注册到 ROS 系统中。最后,节点进入 spin() 循环,等待服务请求。
相关问题
#!/usr/bin/env python # -*- coding: utf-8 -*- import cv2 import numpy as np import rospy from sensor_msgs.msg import Image from cv_bridge import CvBridge, CvBridgeError # 定义要识别的颜色范围 lower_color = np.array([19, 78, 44]) upper_color = np.array([74, 202, 129]) # 初始化cv_bridge bridge = CvBridge() # 定义回调函数,处理订阅到的图像 def image_callback(msg): # 将ROS图像格式转换为OpenCV图像格式 try: cv_image = bridge.imgmsg_to_cv2(msg, 'bgr8') except CvBridgeError as e: print(e) return # 转换颜色空间 hsv = cv2.cvtColor(cv_image, cv2.COLOR_BGR2HSV) # 根据颜色范围进行二值化 mask = cv2.inRange(hsv, lower_color, upper_color) # 寻找轮廓 _, contours, _ = cv2.findContours(mask, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE) # 遍历轮廓 for contour in contours: # 计算轮廓面积 area = cv2.contourArea(contour) # 忽略面积较小的轮廓 if area < 100: continue # 计算轮廓的外接矩形 x, y, w, h = cv2.boundingRect(contour) # 在原图上绘制外接矩形 cv2.rectangle(cv_image, (x, y), (x + w, y + h), (0, 255, 0), 2) # 显示图像 cv2.imshow('frame', cv_image) cv2.waitKey(1) # 初始化节点 rospy.init_node('color_detection') # 订阅摄像头图像 image_sub = rospy.Subscriber('/usb_cam/image_raw', Image, image_callback) # 进入循环 rospy.spin() # 关闭窗口 cv2.destroyAllWindows() 帮我改成检测多种色值的
好的,以下是修改后的代码:
```python
#!/usr/bin/env python
# -*- coding: utf-8 -*-
import cv2
import numpy as np
import rospy
from sensor_msgs.msg import Image
from cv_bridge import CvBridge, CvBridgeError
# 定义要识别的颜色范围
colors = {
'red': ([0, 50, 50], [10, 255, 255]),
'green': ([36, 25, 25], [86, 255, 255]),
'blue': ([100, 50, 50], [130, 255, 255])
}
# 初始化cv_bridge
bridge = CvBridge()
# 定义回调函数,处理订阅到的图像
def image_callback(msg):
# 将ROS图像格式转换为OpenCV图像格式
try:
cv_image = bridge.imgmsg_to_cv2(msg, 'bgr8')
except CvBridgeError as e:
print(e)
return
# 转换颜色空间
hsv = cv2.cvtColor(cv_image, cv2.COLOR_BGR2HSV)
# 遍历所有颜色范围,进行二值化和轮廓检测
for color_name, color_range in colors.items():
lower_color = np.array(color_range[0])
upper_color = np.array(color_range[1])
mask = cv2.inRange(hsv, lower_color, upper_color)
_, contours, _ = cv2.findContours(mask, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 遍历轮廓,绘制外接矩形
for contour in contours:
area = cv2.contourArea(contour)
if area < 100:
continue
x, y, w, h = cv2.boundingRect(contour)
cv2.rectangle(cv_image, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 显示图像
cv2.imshow('frame', cv_image)
cv2.waitKey(1)
# 初始化节点
rospy.init_node('color_detection')
# 订阅摄像头图像
image_sub = rospy.Subscriber('/usb_cam/image_raw', Image, image_callback)
# 进入循环
rospy.spin()
# 关闭窗口
cv2.destroyAllWindows()
```
在上述代码中,我们定义了一个字典 `colors`,其中包含要识别的多种颜色的范围。然后我们遍历所有颜色范围,进行二值化和轮廓检测,并在原图上绘制外接矩形。
# !/usr/bin/env python # -*- coding:utf-8 -*- # author: haotian time:2019/9/14 import numpy as np f = open("./data/CD_Flight190914A.csv", "rb") excel = open("./data/time_flight.csv", "w+") # position_exl = open("./data/position_exl.csv", "w+") schedule = np.loadtxt(f, dtype=str, delimiter=",", skiprows=1, usecols=(4,)) # 分隔符 空格 Array = np.zeros(209) count = 1 i = 0 n = 0 while i < (len(schedule)-1): if schedule[i] == schedule[i + 1] : # 如果航班时间重复 创建一个不重复的时间表记录重复次数 count = count + 1 else: Array[n] = count #Array存的重复次数 count = 0 n = n + 1 i = i + 1 new_schedule,a = np.unique(schedule,return_index=True) #去掉相同时间的数据 # for i in range(len(position)): # position_exl.write(str(position[i])+',\n') # position_exl.close() # position_exl = open(("./data/position_exl.csv", "w+")) # position = np.loadtxt(position_exl, dtype=float, delimiter=",", skiprows=0, usecols=(0,)) # new_schedule = [len(position)*''] # n = 0 # numbers = [ int(x) for x in position ] # for i in range(numbers): # new_schedule[n] = schedule[i] # n = n + 1 excel.write("Schedule,PlaneNum"+'\n') for i in range(len(new_schedule)-1): excel.write(str(new_schedule[i])+","+str(Array[i])+",\n") excel.close() ''' 此时的数据time_flight.csv由于排序的原因导致时间的序列不一致, 最终数据用excel降序排列并保存到schedule_PlaneNum.csv中 '''帮我完善下
这段代码主要是读取一个csv数据文件,统计相同时间出现的次数,并将结果保存到一个新的csv文件中。但是代码中还有一些问题需要完善。我看到你有一些注释,我将根据你的注释进行修改。
```python
#!/usr/bin/env python
# -*- coding: utf-8 -*-
# author: haotian
# time: 2019/9/14
import numpy as np
# 打开数据文件
f = open("./data/CD_Flight190914A.csv", "rb")
# 创建新的csv文件来保存时间和航班次数
excel = open("./data/time_flight.csv", "w+")
# 读取航班时间数据
schedule = np.loadtxt(f, dtype=str, delimiter=",", skiprows=1, usecols=(4,))
# 创建一个数组来存储每个时间重复出现的次数
Array = np.zeros(209)
# 初始化计数器和数组下标
count = 1
n = 0
# 遍历航班时间数据,统计每个时间出现的次数
for i in range(len(schedule)-1):
if schedule[i] == schedule[i + 1]:
count = count + 1
else:
Array[n] = count
count = 1
n = n + 1
# 去除相同时间的数据,只保留第一次出现的时间
new_schedule,a = np.unique(schedule,return_index=True)
# 将时间和对应的航班次数保存到新的csv文件中
excel.write("Schedule,PlaneNum\n")
for i in range(len(new_schedule)):
excel.write(str(new_schedule[i])+","+str(Array[i])+"\n")
# 关闭文件
excel.close()
```
这样就完成了统计时间重复出现次数的代码部分。接下来是对时间进行排序,并保存到新的csv文件中的代码部分。在完成排序前,我们需要先读取之前生成的csv文件中的数据,并将其存储到数组中。修改后的代码如下:
```python
#!/usr/bin/env python
# -*- coding: utf-8 -*-
# author: haotian
# time: 2019/9/14
import numpy as np
import csv
# 打开数据文件
f = open("./data/time_flight.csv", "r")
# 创建新的csv文件来保存排序后的数据
excel = open("./data/schedule_PlaneNum.csv", "w+")
# 读取航班时间和对应的航班次数数据
reader = csv.reader(f)
data = list(reader)
# 将数据保存到数组中
new_schedule = []
Array = []
for i in range(1, len(data)):
new_schedule.append(data[i][0])
Array.append(int(data[i][1]))
# 对时间进行排序
sorted_idx = np.argsort(new_schedule)
new_schedule = np.array(new_schedule)[sorted_idx]
Array = np.array(Array)[sorted_idx]
# 将排序后的时间和对应的航班次数保存到新的csv文件中
excel.write("Schedule,PlaneNum\n")
for i in range(len(new_schedule)):
excel.write(str(new_schedule[i])+","+str(Array[i])+"\n")
# 关闭文件
f.close()
excel.close()
```
这样就完成了对时间进行排序的代码部分。需要注意的是,排序前需要先将读取的时间和航班次数数据保存到数组中,排序后再将数据写入到csv文件中。
希望这些修改能够帮助你完成你的代码。
相关推荐
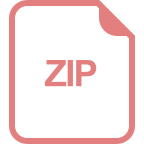
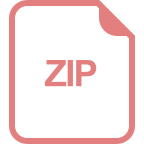
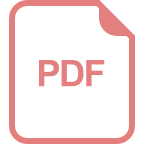










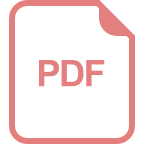
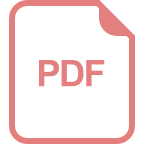
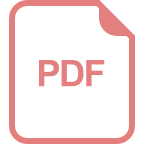