用python输入一个二叉树判断是否对称
时间: 2023-05-20 15:01:40 浏览: 119
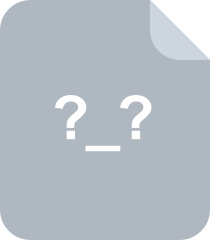
判断二叉树是否相似的问题
可以使用递归的方式判断二叉树是否对称。具体实现如下:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def isSymmetric(root: TreeNode) -> bool:
def helper(left: TreeNode, right: TreeNode) -> bool:
if not left and not right:
return True
if not left or not right:
return False
if left.val != right.val:
return False
return helper(left.left, right.right) and helper(left.right, right.left)
if not root:
return True
return helper(root.left, root.right)
# 示例
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(2)
root.left.left = TreeNode(3)
root.left.right = TreeNode(4)
root.right.left = TreeNode(4)
root.right.right = TreeNode(3)
print(isSymmetric(root)) # 输出 True
```
这段代码中,`isSymmetric` 函数接收一个二叉树的根节点作为参数,返回一个布尔值,表示该二叉树是否对称。`helper` 函数是一个递归函数,用于判断左右子树是否对称。如果左右子树都为空,则返回 True;如果左右子树其中一个为空,则返回 False;如果左右子树的值不相等,则返回 False;否则递归判断左子树的左子树和右子树的右子树是否对称,以及左子树的右子树和右子树的左子树是否对称。最后,如果根节点为空,则返回 True,否则调用 `helper` 函数判断左右子树是否对称。
阅读全文
相关推荐
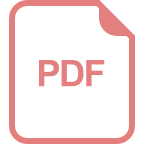


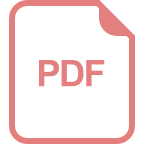
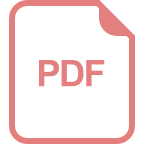

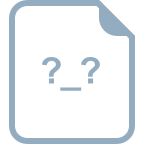
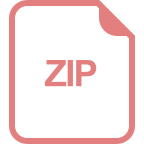
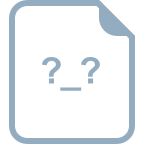
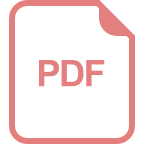
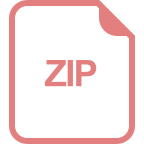
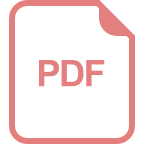


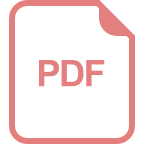