请使用MATLAB编写程序.用Jacobi解如下方程组(误差为0.5*10e6)
时间: 2024-10-23 21:17:41 浏览: 81
在MATLAB中,我们可以使用内置函数`jacobi`来求解线性系统,尤其是当矩阵是对称正定或接近对称正定的情况,因为Jacobi迭代法在这种条件下收敛速度较快。假设我们有一个二维数组`A`代表系数矩阵,一个列向量`b`代表常数项,你可以按照以下步骤编写程序:
```matlab
% 假设你已经有了系数矩阵A和常数项向量b
A = ...; % 你的系数矩阵
b = ...; % 你的常数项向量
% 设置初始猜测值,通常选择零向量
x0 = zeros(size(A,2), 1);
% 设置迭代次数
max_iter = 1000;
tolerance = 0.5 * 10^6; % 确保达到指定的误差范围
% 使用Jacobi迭代法
[x, iter] = jacobi(A, b, x0, max_iter, tolerance);
% 检查是否达到所需的精度
if norm(residual(A*x - b)) > tolerance
error('未能达到指定的误差');
end
% 输出结果
disp("Solution after Jacobi iteration:");
disp(x);
```
在这个代码片段中,`jacobi`函数会返回迭代得到的解`x`以及迭代步数`iter`。如果迭代过程中达到预设的最大迭代次数`max_iter`而未达到指定的误差`tolerance`,程序还会抛出错误。
相关问题
请使用MATLAB编写程序.用Jacobi解如下方程组(误差为0.5*106
在MATLAB中,你可以使用内置的`jacobi`函数来求解线性系统,特别是当它涉及到对角占优矩阵时,Jacobi迭代法是一个不错的选择。假设你有一个线性方程组Ax = b,其中A是一个n×n的对角占优矩阵,x是你需要找到的未知数向量,b是已知的右侧向量。
下面是一个简单的例子,说明如何用MATLAB的Jacobi方法求解这个问题,并设置误差阈值为0.5 * 10^(-6):
```matlab
% 初始化数据
n = size(A, 1); % 系统的维度
b = rand(n, 1); % 随机生成右部向量
tol = 0.5e-6; % 误差阈值
% 如果是二维矩阵(不是向量),转换为列向量
if ismatrix(b) && ndims(b) == 2
b = b';
end
% 创建初始猜测和收敛标志
x_guess = zeros(n, 1);
converged = false;
% 迭代开始
max_iter = 1000; % 设置最大迭代次数
for iter = 1:max_iter
d = A - speye(n); % 对角差分矩阵
x_new = (b + d*x_guess)./diag(diag(A)); % Jacobi更新
error = norm(x_new - x_guess, 'fro'); % 计算当前误差
if error < tol
converged = true;
break; % 如果达到误差阈值,停止迭代
end
x_guess = x_new; % 更新猜测值
end
% 检查是否收敛
if converged
fprintf('Converged after %d iterations with an error of %f.\n', iter, error);
else
warning('Did not converge within the maximum number of iterations.');
end
% 输出结果
x = x_guess;
```
在这个示例中,我们首先检查输入是否为向量,如果是,则将其转换为列向量。然后,我们开始循环迭代,直到达到预设的误差阈值或者达到最大迭代次数。`norm`函数计算了当前迭代和上一次迭代之间的Frobenius norm,这是衡量向量差异的一个标准。如果满足收敛条件,就输出结果并结束程序;否则发出警告信息。
请使用MATLAB编写程序.用Jacobi解如下方程组(误差为0.5*106)
在MATLAB中,你可以使用内置函数`linsolve`或者自定义迭代算法(如雅可比(Jacobi)迭代法)来求解线性方程组。由于雅可比迭代法通常用于数值求解而非直接求解方程组,这里我将展示如何使用迭代法来解决这个问题。
首先,假设我们有一个线性方程组 `Ax = b`,其中 `A` 是系数矩阵,`b` 是常数向量。雅可比迭代法的步骤大致如下:
1. 初始化:选择一个初始猜测 `x0`。
2. 迭代:对于每个迭代 `k`,计算新的近似解 `x(k+1)` 通过 `x(k+1) = x(k) + (1/A diagonal)(b - A*x(k))`,其中 `(1/A diagonal)` 表示对角线上元素的倒数。
3. 检查收敛:如果满足误差准则(例如,`norm(A*x(k+1) - b) < 0.5e6`),则停止迭代;否则继续。
下面是一个简单的雅可比迭代法的MATLAB伪代码:
```matlab
function [x, iter] = jacobi(A, b, tolerance, max_iterations)
% 初始化
x = zeros(size(b)); % 初始猜测
iter = 0;
% 雅可比迭代
while iter < max_iterations
iter = iter + 1;
d = diag(diag(A)); % 获取对角线元素
dx = inv(d) * (b - A * x); % 计算增量
x = x + dx; % 更新解
if norm(A*x - b) < tolerance
break;
end
end
% 返回结果
if iter == max_iterations
warning('Maximum iterations reached without convergence.');
end
```
请注意,在实际应用中,你需要将 `A` 和 `b` 替换为你具体的方程组,`tolerance` 设置为所需的误差值,`max_iterations` 设定为允许的最大迭代次数。
阅读全文
相关推荐












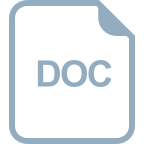



