解题思路: 由于兔子只能向上下左右移动,因此我们可以采用广度优先搜索(BFS)来求兔子到达终点的最短路径,同时乌龟可以直接沿一条直线走向终点,因此乌龟到终点的距离可以直接计算。最终答案即为兔子到终点的最短路径长度减去乌龟到终点的距离。 具体实现: 读入起点和终点坐标。 BFS搜索兔子到达终点的最短路径长度。 计算乌龟到终点的距离。 计算兔子比乌龟多走的距离,并输出结果(注意保留两位小数)。用Python写
时间: 2023-06-18 17:07:42 浏览: 118
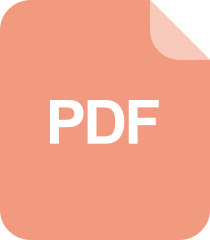
Java实现利用广度优先遍历(BFS)计算最短路径的方法
以下是Python实现代码:
```python
from collections import deque
# 定义坐标类
class Coordinate:
def __init__(self, x, y):
self.x = x
self.y = y
# 定义BFS函数
def bfs(start, end, maze):
queue = deque()
# 记录起点到每个点的最短距离
distance = [[-1] * len(maze[0]) for _ in range(len(maze))]
distance[start.x][start.y] = 0
queue.append(start)
while queue:
cur = queue.popleft()
# 找到终点,返回最短路径长度
if cur.x == end.x and cur.y == end.y:
return distance[cur.x][cur.y]
# 四个方向进行搜索
for dx, dy in [(-1, 0), (1, 0), (0, -1), (0, 1)]:
new_x, new_y = cur.x + dx, cur.y + dy
# 如果新坐标越界或者是墙壁或者已经被搜索过,直接跳过
if not (0 <= new_x < len(maze) and 0 <= new_y < len(maze[0]) and maze[new_x][new_y] == '.' and distance[new_x][new_y] == -1):
continue
# 更新最短距离并加入队列
distance[new_x][new_y] = distance[cur.x][cur.y] + 1
queue.append(Coordinate(new_x, new_y))
# 没有找到终点,返回-1
return -1
# 读入输入
start_x, start_y, end_x, end_y = map(int, input().split())
maze = []
for i in range(10):
maze.append(input())
# 计算乌龟到终点的距离
turtle_distance = abs(end_x - start_x) + abs(end_y - start_y)
# 计算兔子到终点的最短路径长度
start = Coordinate(start_x, start_y)
end = Coordinate(end_x, end_y)
rabbit_distance = bfs(start, end, maze)
# 计算兔子比乌龟多走的距离
extra_distance = rabbit_distance - turtle_distance
# 输出结果
print('%.2f' % extra_distance)
```
注意,以上代码中用到了坐标类`Coordinate`和队列`deque`。`Coordinate`类用于表示二维坐标,`deque`则是Python标准库中的双端队列,可以高效地实现队列的操作。在BFS中,我们需要使用队列来保存待搜索的坐标。
阅读全文
相关推荐
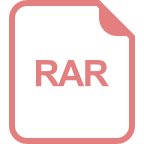
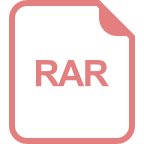















