翻译代码@app.route('/comment',methods=['GET']) def comment(): limit = int(request.args['limit']) page = int(request.args['page']) page = (page-1)limit conn = pymysql.connect(host='127.0.0.1', user='abc', password='123456', port=3306, db='abc', charset='utf8mb4') cursor = conn.cursor() if(len(request.args)==2): cursor.execute("select count() from comment"); count = cursor.fetchall() cursor = conn.cursor(cursor=pymysql.cursors.DictCursor) cursor.execute("select * from comment limit " + str(page) + "," + str(limit)); data_dict = [] result = cursor.fetchall() for field in result: data_dict.append(field) else: type = str(request.args['type']) cursor.execute("select count(*) from comment where type= '"+type+"'"); count = cursor.fetchall() cursor = conn.cursor(cursor=pymysql.cursors.DictCursor) cursor.execute("select * from comment where type= '"+type+"' limit " + str(page) + "," + str(limit)); data_dict = [] result = cursor.fetchall() for field in result: data_dict.append(field) table_result = {"code": 0, "msg": None, "count": count[0], "data": data_dict} cursor.close() conn.close() return jsonify(table_result)
时间: 2024-02-26 22:51:37 浏览: 87
这是一个使用 Flask 框架开发的接口,该接口用于获取评论数据。接口使用了 GET 请求方法,并接受两个参数:limit 和 page,用于指定返回数据的数量和页数。代码中使用了 pymysql 库连接 MySQL 数据库,并执行了 SQL 查询语句获取数据。如果请求参数中未包含 type 参数,则查询所有评论数据;否则,根据 type 参数查询指定类型的评论。最终将查询结果封装成一个 JSON 格式的数据返回。
相关问题
上面的前端代码加上下面的后端代码,访问报错,帮我检查错误from flask import Flask, make_response from flask import render_template from flask import request import os app = Flask(__name__) @app.route('/') def index(): return render_template('1.html') # @app.route('/index/') # def root(): # uid = str(request.args['uid']) # with open("code_save.csv", "a+", encoding='utf-8')as f: # f.write(uid) # # os.system("python test.py") # response = make_response("被返回的信息") # return response @app.route('/post/') def post(): json = request.json print(json+'-*****************************************') return json if __name__ == '__main__': app.run(debug=True, host='0.0.0.0', port=112)
你的代码中有一些问题,可能导致运行出错。错误可能来自以下几个方面:
1. 没有在 HTML 文件中设置正确的表单提交方式。
在 `1.html` 文件中,你需要将表单的提交方式设置为 POST 方法,如下所示:
```html
<form action="/post/" method="POST">
<!-- 表单内容 -->
</form>
```
2. 没有导入 Flask 库中的 request 和 make_response 方法。
在你的代码中已经导入了这两个方法,但是你需要将它们从 Flask 库中导入,如下所示:
```python
from flask import Flask, render_template, request, make_response
```
3. 没有正确处理 POST 请求的数据。
在 `post()` 函数中,你需要使用 `request.get_json()` 方法获取 POST 请求中的数据,而不是使用 `request.json` 属性。修改后的代码如下:
```python
@app.route('/post/', methods=['POST'])
def post():
json = request.get_json()
print(json+'-*****************************************')
return json
```
4. 没有正确设置路由。
在你的代码中,`root()` 函数被注释掉了,并且没有设置路由。如果你想要使用 `/index/` 路由,你需要取消注释 `root()` 函数,并将其设置为该路由的处理函数,如下所示:
```python
@app.route('/index/')
def root():
uid = str(request.args['uid'])
with open("code_save.csv", "a+", encoding='utf-8') as f:
f.write(uid)
# os.system("python test.py")
response = make_response("被返回的信息")
return response
```
如果你不需要该路由,可以将其删除。
from flask import request, render_template,session,redirect, url_for from models import * import models from sqlalchemy import or_,and_ @app.route('/', methods=['GET', 'POST']) @app.route('/index', methods=['GET', 'POST']) def index(): uuid = session.get('uuid') if not User.query.get(uuid): return redirect(url_for('login')) if request.method == 'GET': search = request.args.get('search', '') if search: datas = KeCheng.query.filter(and_(KeCheng.name.like("%{}%".format(search)))).all() else: datas = KeCheng.query.all() return render_template('app/table.html',**locals())注释这段代码
这段代码是一个 Python Flask 应用中的路由定义,用于处理首页的 GET 和 POST 请求。该路由定义了两个 URL,一个是根目录 '/',另一个是 '/index',两者的处理逻辑相同。在处理 GET 请求时,代码首先获取 session 中保存的 uuid,如果 uuid 不存在或者对应的用户不存在,就重定向到登录页面。如果存在请求参数 search,代码就根据搜索关键字查询课程数据表,否则查询全部数据。最后,将查询结果传给模板,渲染出表格页面。在处理 POST 请求时,该路由暂时没有任何逻辑。这段代码同样注释清晰,易于理解。
阅读全文
相关推荐
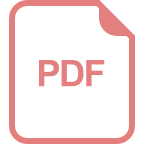
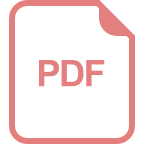
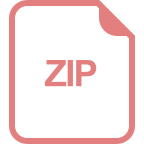













