python整理json数据
时间: 2023-04-05 11:02:57 浏览: 76
好的,我可以回答这个问题。Python可以使用json模块来整理json数据。可以使用json.loads()函数将json字符串转换为Python对象,使用json.dumps()函数将Python对象转换为json字符串。还可以使用json.load()函数从文件中读取json数据,使用json.dump()函数将json数据写入文件。
相关问题
python数据清洗json文件
回答: 清洗JSON文件是指对JSON格式的数据进行处理和统计的过程。在Python中,可以使用相关的工具包来实现这个目标。首先,通过导入json、os、shutil和csv等工具包,可以方便地处理JSON格式的数据、进行文件路径操作、复制文件以及整理数据并进行可视化操作。\[1\]接下来,可以使用getDirList函数来获取指定路径下的所有文件名,并将其存储在一个列表中。\[2\]最后,根据实际需要,可以根据JSON文件的具体形式,使用相应的代码对数据进行提取、处理和统计。需要注意的是,实际的JSON数据可能具有不同的形式,因此可能需要根据具体情况进行适应性修改。如果在处理过程中遇到问题,可以随时联系相关人员进行咨询和帮助。\[3\]
#### 引用[.reference_title]
- *1* *2* [Python Code :不同Json文件的数据挖掘、清洗、反写](https://blog.csdn.net/Errors_In_Life/article/details/71968489)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [【Python】实现json数据清洗、json数据去重、json数据统计](https://blog.csdn.net/qq_35902025/article/details/130428789)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python json excel
使用Python将JSON数据整理到Excel可以有多种方法。引用提供了一种方法,使用`json`和`tablib`库来处理JSON数据并将其转换为Excel文件。首先,你需要导入`json`和`tablib`库。然后,使用`open`函数打开JSON文件,并使用`json.load`函数将数据加载到变量`rows`中。接下来,将JSON中的键作为表头,创建一个元组`header`。然后,创建一个空列表`data`,并循环遍历每个字典中的值,将其添加到`body`列表中。将`body`转换为元组,并将其添加到`data`列表中。最后,使用`tablib.Dataset`函数创建一个数据集`data`,并使用`open`函数将数据集保存为Excel文件。这种方法适用于嵌套的JSON数据。
引用提供了另一种简单的方法,使用`pandas`库将单层JSON列表直接转换为数据框,并输出为Excel文件。首先,导入`pandas`库,并使用`pd.DataFrame`函数将JSON数据转换为数据框`df`。然后,使用`to_excel`函数将数据框保存为Excel文件。这种方法适用于单层的JSON数据。
另外,引用介绍了如何通过Python将JSON数据整理到Excel。具体步骤如下:
1. 首先,解除JSON的嵌套关系。你可以使用递归或循环的方式来解除嵌套关系,确保将所有层级的数据都提取出来,以便后续处理。
2. 接下来,将解除嵌套关系后的JSON数据转换为数据框,可以使用`pandas`库中的`pd.DataFrame`函数。
3. 最后,使用`to_excel`函数将数据框保存为Excel文件。
这些方法可以根据你的具体需求选择使用。无论你选择哪种方法,都可以使用Python轻松地将JSON数据整理到Excel文件中。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [python中 将json数据转化为excel表格](https://blog.csdn.net/weixin_44774255/article/details/119146211)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [Python3用简单的方法,将一组具有相同格式的json数据(将多层json展开到一层),整理到excel中](https://blog.csdn.net/watfe/article/details/96313024)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
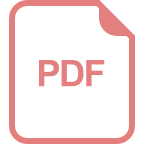
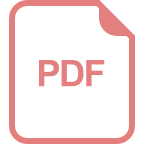












