c语言单链表原地逆置
时间: 2023-11-07 17:18:44 浏览: 81
你可以使用迭代或递归的方式来实现单链表的原地逆置。下面是使用迭代方式的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 原地逆置单链表
struct Node* reverseList(struct Node* head) {
struct Node *prev = NULL;
struct Node *curr = head;
struct Node *next = NULL;
while (curr != NULL) {
next = curr->next; // 保存当前节点的下一个节点
curr->next = prev; // 当前节点指向前一个节点
prev = curr; // 更新前一个节点为当前节点
curr = next; // 更新当前节点为下一个节点
}
return prev; // 返回逆置后的头节点
}
// 打印链表
void printList(struct Node* head) {
struct Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
// 创建链表
struct Node* head = (struct Node*)malloc(sizeof(struct Node));
struct Node* second = (struct Node*)malloc(sizeof(struct Node));
struct Node* third = (struct Node*)malloc(sizeof(struct Node));
struct Node* fourth = (struct Node*)malloc(sizeof(struct Node));
head->data = 1;
head->next = second;
second->data = 2;
second->next = third;
third->data = 3;
third->next = fourth;
fourth->data = 4;
fourth->next = NULL;
printf("原链表:");
printList(head);
// 逆置链表
head = reverseList(head);
printf("逆置后的链表:");
printList(head);
return 0;
}
```
这段代码首先定义了一个单链表节点结构体 `Node`,包含一个整型数据 `data` 和指向下一个节点的指针 `next`。然后,定义了 `reverseList` 函数,通过迭代的方式实现了单链表的原地逆置。最后,在 `main` 函数中创建了一个简单的链表,并调用 `reverseList` 函数进行逆置,打印出逆置后的结果。
希望能帮到你!如果有任何疑问,请随时提问。
相关推荐
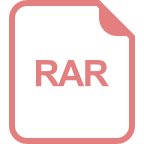
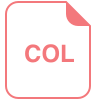













