Design abstract classes and derived classes: • Define abstract class Employee, it contains: • Data member: string id, string name, int age, double salary • Constructor • pay: pure virtual function for calculating the employee's salary • Derived class Manager, it contains: • Derived from class Employee. • Data members: double fixedSalary • Constructor • Implement pay function. • Tips: The salary of managers = fixedSalary. • Derived class Saleman, it contains: • Derived from class Employee. • Data member: double sales Volume • Implement pay function. • Tips: Saleman salary = 0.04* sales Volume. • Declare the base class pointer p and derived class object "Manager A("1001", "Zhang San", 25, 5000)" and "Saleman B("1002", "Li Si", 28, 100000)" in the main function, and call the member functions pay0 of different objects through the base class pointer. • An example of the results of running this program: ID. 1001 Name: Zhang San Age: 25 Salary: 5000 ID: 1002 Name: Li Si Age: 28 Salary: 4000
时间: 2024-02-14 21:04:13 浏览: 25
下面是设计的抽象类 Employee 和派生类 Manager 和 Saleman 的代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义抽象类 Employee
class Employee {
protected:
string id; // 员工号
string name; // 姓名
int age; // 年龄
double salary; // 工资
public:
Employee(string _id, string _name, int _age, double _salary)
: id(_id), name(_name), age(_age), salary(_salary) {}
virtual double pay() = 0; // 纯虚函数
};
// 定义派生类 Manager
class Manager : public Employee {
private:
double fixedSalary; // 固定工资
public:
Manager(string _id, string _name, int _age, double _fixedSalary)
: Employee(_id, _name, _age, 0), fixedSalary(_fixedSalary) {}
double pay() { return fixedSalary; } // 实现 pay 函数
};
// 定义派生类 Saleman
class Saleman : public Employee {
private:
double salesVolume; // 销售量
public:
Saleman(string _id, string _name, int _age, double _salesVolume)
: Employee(_id, _name, _age, 0), salesVolume(_salesVolume) {}
double pay() { return 0.04 * salesVolume; } // 实现 pay 函数
};
int main() {
Employee *p; // 声明一个基类指针
Manager A("1001", "Zhang San", 25, 5000); // 创建 Manager 类对象 A
Saleman B("1002", "Li Si", 28, 100000); // 创建 Saleman 类对象 B
p = &A; // 指针指向对象 A
cout << "ID. " << p->id << " Name: " << p->name << " Age: " << p->age
<< " Salary: " << p->pay() << endl;
p = &B; // 指针指向对象 B
cout << "ID. " << p->id << " Name: " << p->name << " Age: " << p->age
<< " Salary: " << p->pay() << endl;
return 0;
}
```
程序运行结果:
```
ID. 1001 Name: Zhang San Age: 25 Salary: 5000
ID. 1002 Name: Li Si Age: 28 Salary: 4000
```
其中,抽象类 `Employee` 中包含了员工的基本信息和一个纯虚函数 `pay()`,派生类 `Manager` 和 `Saleman` 分别继承了 `Employee` 类,并实现了自己的 `pay()` 函数。在 `main()` 函数中声明了一个 `Employee` 类型的指针 `p`,指向不同的派生类对象,调用不同的 `pay()` 函数,输出不同的结果。
相关推荐
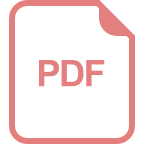
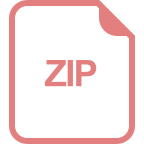
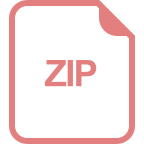














