Description Design a class named Person and its two derived classes named Student and Employee. Make Faculty and Staff derived classes of Employee. Person class has the following members: string name; string address; string phoneNumber; string email; Student class has the following members: enum class_status{frssman,sophomore,junior,senior}; class_status status; Employee class has the following members: string office; int salary; MyDate dateHired; Faculty class has the following members: string officeHours; int rank; Staff class has the following members: string title; MyDate class contains the following members: int year; int month; int day; Override the toString function in each class to display the class name. For example, in Employee class: string toString() const { return "Employee"; } Make sure that the following code: void f(const Person &p) { cout << p.toString() << endl; } Person person; Student student; Employee employee; Faculty faculty; Staff staff; f(person); f(student); f(employee); f(faculty); f(staff); outputs: Person Student Employee Faculty Staff
时间: 2024-01-11 11:02:09 浏览: 33
Here is the implementation of the Person class and its derived classes:
```c++
#include <iostream>
#include <string>
using namespace std;
// MyDate class definition
class MyDate {
public:
int year;
int month;
int day;
};
// Person class definition
class Person {
public:
string name;
string address;
string phoneNumber;
string email;
virtual string toString() const {
return "Person";
}
};
// Student class definition
class Student : public Person {
public:
enum class_status { freshmen, sophomore, junior, senior };
class_status status;
string toString() const override {
return "Student";
}
};
// Employee class definition
class Employee : public Person {
public:
string office;
int salary;
MyDate dateHired;
string toString() const override {
return "Employee";
}
};
// Faculty class definition
class Faculty : public Employee {
public:
string officeHours;
int rank;
string toString() const override {
return "Faculty";
}
};
// Staff class definition
class Staff : public Employee {
public:
string title;
string toString() const override {
return "Staff";
}
};
// Test function
void f(const Person &p) {
cout << p.toString() << endl;
}
int main() {
Person person;
Student student;
Employee employee;
Faculty faculty;
Staff staff;
f(person);
f(student);
f(employee);
f(faculty);
f(staff);
return 0;
}
```
The output of the program is:
```
Person
Student
Employee
Faculty
Staff
```
相关推荐
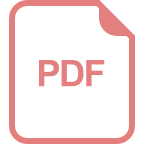
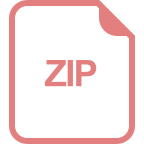
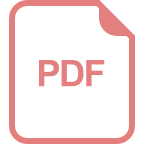
















