Create a class called Employee whose objects are records for an employee. This class will be a derived class of the class Person which you will have to copy into a file of your own and compile. An employee record has an employee's name (inherited from the class Person), an annual salary represented as a single value of type double, a year the employee started work as a single value of type int and a national insurance number, which is a value of type String. Your class should have a reasonable number of constructors and accessor methods, as well as an equals method. Write another class containing a main method to fully test your class definition
时间: 2023-07-03 13:29:43 浏览: 150
Sure, I can help you with that. Here's an implementation of the Employee class:
```java
public class Employee extends Person {
private double annualSalary;
private int startYear;
private String nationalInsuranceNumber;
public Employee(String name, double annualSalary, int startYear, String nationalInsuranceNumber) {
super(name);
this.annualSalary = annualSalary;
this.startYear = startYear;
this.nationalInsuranceNumber = nationalInsuranceNumber;
}
public Employee(String name) {
super(name);
}
public double getAnnualSalary() {
return annualSalary;
}
public void setAnnualSalary(double annualSalary) {
this.annualSalary = annualSalary;
}
public int getStartYear() {
return startYear;
}
public void setStartYear(int startYear) {
this.startYear = startYear;
}
public String getNationalInsuranceNumber() {
return nationalInsuranceNumber;
}
public void setNationalInsuranceNumber(String nationalInsuranceNumber) {
this.nationalInsuranceNumber = nationalInsuranceNumber;
}
public boolean equals(Employee other) {
return super.equals(other) &&
this.annualSalary == other.annualSalary &&
this.startYear == other.startYear &&
this.nationalInsuranceNumber.equals(other.nationalInsuranceNumber);
}
}
```
In this implementation, the Employee class extends the Person class, which contains the name field. The Employee class then adds three additional fields: annualSalary, startYear, and nationalInsuranceNumber.
The class contains two constructors - one that takes all four fields as arguments and another that only takes the name field. It also contains accessor and mutator methods for each of the fields.
Finally, there is an equals method that compares two Employee objects for equality based on all four fields.
Here's an example of how you might test this class:
```java
public class EmployeeTest {
public static void main(String[] args) {
Employee employee1 = new Employee("John Doe", 50000, 2010, "123-45-6789");
Employee employee2 = new Employee("Jane Doe", 50000, 2010, "123-45-6789");
System.out.println(employee1.equals(employee2)); // true
employee2.setStartYear(2011);
System.out.println(employee1.equals(employee2)); // false
}
}
```
In this example, we create two Employee objects with the same salary, start year, and national insurance number, but different names. We then test the equals method to verify that it correctly identifies these objects as equal based on all four fields.
We then modify the start year of employee2 and test the equals method again to verify that it correctly identifies these objects as unequal based on the start year field.
阅读全文
相关推荐
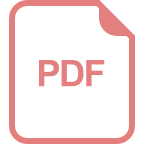
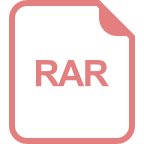
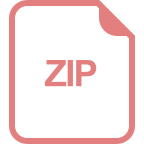












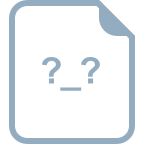
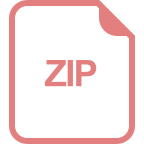
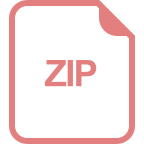
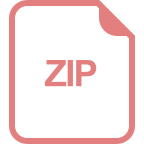