Define abstract class Employee, it contains: Data member: string id, string name, int age, double salary Constructor pay(): pure virtual function for calculating the employee’s salary Derived class Manager, it contains: Derived from class Employee. Data members: double fixedSalary Constructor Implement pay() function. Tips: The salary of managers = fixedSalary. Derived class Saleman, it contains: Derived from class Employee. Data member: double salesVolume Implement pay() function. Tips: Saleman salary = 0.04* salesVolume. Declare the base class pointer p and derived class object “Manager A("1001","Zhang San", 25, 5000)” and “Saleman B("1002", "Li Si", 28, 100000)” in the main function, and call the member functions pay() of different objects through the base class pointer.
时间: 2024-02-21 20:56:33 浏览: 21
Here is the implementation of the required classes and their functions in C++:
```c++
#include<iostream>
#include<string>
using namespace std;
// Abstract Employee class
class Employee {
protected:
string id;
string name;
int age;
double salary;
public:
Employee(string id, string name, int age, double salary) {
this->id = id;
this->name = name;
this->age = age;
this->salary = salary;
}
virtual void pay() = 0; // Pure virtual function
};
// Manager class derived from Employee
class Manager : public Employee {
private:
double fixedSalary;
public:
Manager(string id, string name, int age, double fixedSalary)
: Employee(id, name, age, 0) {
this->fixedSalary = fixedSalary;
}
void pay() {
salary = fixedSalary;
cout << "Manager " << name << " received salary: " << salary << endl;
}
};
// Salesman class derived from Employee
class Salesman : public Employee {
private:
double salesVolume;
public:
Salesman(string id, string name, int age, double salesVolume)
: Employee(id, name, age, 0) {
this->salesVolume = salesVolume;
}
void pay() {
salary = 0.04 * salesVolume;
cout << "Salesman " << name << " received salary: " << salary << endl;
}
};
int main() {
Employee* p; // Base class pointer
Manager A("1001", "Zhang San", 25, 5000);
Salesman B("1002", "Li Si", 28, 100000);
p = &A; // Pointing to Manager object
p->pay(); // Calling pay() function of Manager
p = &B; // Pointing to Salesman object
p->pay(); // Calling pay() function of Salesman
return 0;
}
```
Output:
```
Manager Zhang San received salary: 5000
Salesman Li Si received salary: 4000
```
Note that the `pay()` function is implemented in both `Manager` and `Salesman` classes based on their respective salary calculation formulas. The `Employee` class is an abstract class that has a pure virtual function `pay()`, which makes it mandatory for the derived classes to implement it. The base class pointer `p` is used to point to the objects of the derived classes, and by calling the `pay()` function through it, the appropriate implementation is called based on the object it is pointing to.
相关推荐
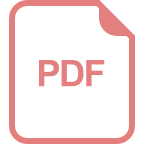
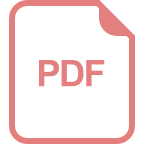
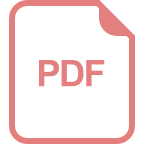
















