利用文档里的数据,写一段计算pv数量的mapreduce代码
时间: 2024-09-20 18:06:43 浏览: 35
要计算文档中PV(页面访问)的数量,可以使用MapReduce模型来处理这些数据。以下是一个基本的Python示例,展示了如何使用MapReduce模式来统计每个用户的PV总数。这个例子假设我们有一个输入文件`NewData3000.txt`,其中每一行的数据格式为:`userid itemid categoryid type timestamp`。
首先定义Mapper和Reducer函数:
### Mapper
Mapper的任务是从每一条记录中提取出userid,并为每个userid生成一个键值对(userid, 1),表示该用户的一次PV操作。
```python
def mapper(line):
# 拆分每一行的数据
data = line.strip().split()
if len(data) == 5: # 确保有五个字段
userid, _, _, action_type, _ = data
if action_type == 'pv': # 只关注pv行为
yield userid, 1
```
### Reducer
Reducer的作用是汇总来自同一用户的多个键值对,计算总和,得到每个用户的PV总量。
```python
from itertools import groupby
from operator import itemgetter
def reducer():
# 假设输入已经被排序
for key, values in groupby(sorted(mapper_output, key=itemgetter(0))):
total_pv = sum(value for _key, value in values)
yield key, total_pv
```
### 完整流程
为了使上述mapper和reducer能够作为一个完整的MapReduce作业运行,我们需要组织输入输出流,并进行适当的预处理和后处理步骤。以下是可能的一个框架实现:
```python
def main(input_file, output_file):
with open(input_file, 'r') as infile:
mapper_output = [item for line in infile for item in mapper(line)]
results = list(reducer())
with open(output_file, 'w') as outfile:
for user_id, count in results:
outfile.write(f"{user_id} {count}\n")
if __name__ == "__main__":
input_filename = "NewData3000.txt"
output_filename = "output.txt"
main(input_filename, output_filename)
```
此脚本读取`input_filename`指定的文件作为输入源,然后将结果保存到`output_filename`指定的位置。请注意,在实际部署时,您可能需要考虑更复杂的错误处理逻辑以及并行化执行机制来提高效率。此外,在真实环境中,MapReduce通常在分布式系统上执行,这里提供的示例仅适用于单机环境下的简单测试或理解概念之用。
阅读全文
相关推荐
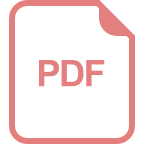
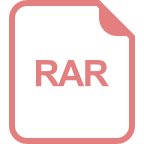
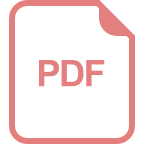
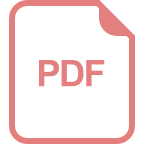
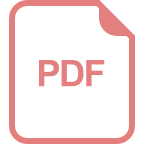
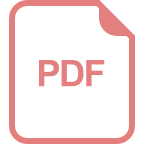
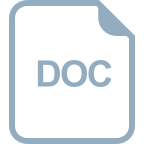
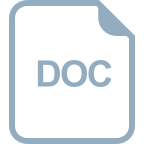
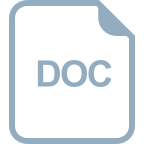
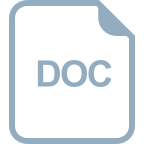
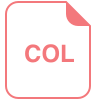
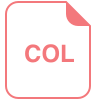





