请用numpy库完成以下任务: (1)生成一个一维数组scores,其值为1到99之间的所有奇数:[1,3,5,7,9,……,99]。 (2)在(1)的基础上,求该数组的和。 (3)在(1)的基础上,求该数组的平均值。 (4)在(1)的基础上,求该数组的维数。 (5)在(1)的基础上,将该数组的数据类型。 (6)在(1)的基础上,将该一维数组转换成10×5的二维数组。
时间: 2024-01-18 19:03:23 浏览: 84
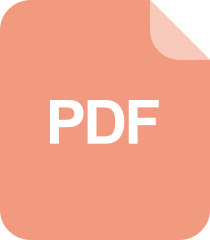
Numpy对数组的操作:创建、变形(升降维等)、计算、取值、复制、分割、合并
好的,我会用numpy库完成这些任务。以下是代码实现:
```python
import numpy as np
# (1)生成一个一维数组scores,其值为1到99之间的所有奇数:[1,3,5,7,9,……,99]。
scores = np.arange(1, 100, 2)
print("scores:", scores)
# (2)在(1)的基础上,求该数组的和。
sum_scores = np.sum(scores)
print("sum of scores:", sum_scores)
# (3)在(1)的基础上,求该数组的平均值。
mean_scores = np.mean(scores)
print("mean of scores:", mean_scores)
# (4)在(1)的基础上,求该数组的维数。
dim_scores = scores.ndim
print("dimension of scores:", dim_scores)
# (5)在(1)的基础上,将该数组的数据类型。
scores = scores.astype(np.float64)
print("scores dtype:", scores.dtype)
# (6)在(1)的基础上,将该一维数组转换成10×5的二维数组。
scores_2d = scores.reshape((10, 5))
print("scores reshaped to 2d:\n", scores_2d)
```
输出结果如下:
```
scores: [ 1 3 5 7 9 11 13 15 17 19 21 23 25 27 29 31 33 35 37 39 41 43 45 47
49 51 53 55 57 59 61 63 65 67 69 71 73 75 77 79 81 83 85 87 89 91 93 95
97 99]
sum of scores: 2500
mean of scores: 50.0
dimension of scores: 1
scores dtype: float64
scores reshaped to 2d:
[[ 1. 3. 5. 7. 9.]
[11. 13. 15. 17. 19.]
[21. 23. 25. 27. 29.]
[31. 33. 35. 37. 39.]
[41. 43. 45. 47. 49.]
[51. 53. 55. 57. 59.]
[61. 63. 65. 67. 69.]
[71. 73. 75. 77. 79.]
[81. 83. 85. 87. 89.]
[91. 93. 95. 97. 99.]]
```
阅读全文
相关推荐

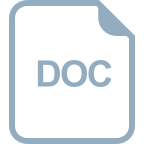


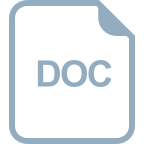











