解释以下代码 retcode = subprocess.Popen("date", shell=True,cwd=contents, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
时间: 2024-03-07 14:53:20 浏览: 151
这段代码使用Python的subprocess模块启动一个新的进程,并执行shell命令"date"。其中:
- "shell=True"表示使用shell来执行命令;
- "cwd=contents"表示将当前工作目录设置为"contents";
- "stdout=subprocess.PIPE"表示将标准输出重定向到一个管道,以便在Python中捕获命令的输出;
- "stderr=subprocess.PIPE"表示将标准错误输出重定向到一个管道,以便在Python中捕获命令的错误输出。
执行完命令后,该代码会返回一个subprocess.Popen对象,该对象包含了命令的执行结果和状态信息。其中,retcode可以通过retcode.returncode获取命令的返回值,通常情况下,返回值为0表示命令执行成功,非0表示命令执行失败。
相关问题
优化以下代码 def push_lib(self,contents,path, ref): retcode = subprocess.call("git add {} && git commit -m 'update lib' && git push -u origin {} ".format(path, ref), shell=True, cwd=contents) return retcode
可以尝试使用 GitPython 库来代替 subprocess 调用 Git 命令,从而更加直接地使用 Python 进行 Git 操作,避免使用 shell 时可能出现的错误。同时,可以使用 GitPython 提供的更高层次的接口来简化代码。
以下是使用 GitPython 重构后的代码:
```
import git
def push_lib(self, contents, path, ref):
repo = git.Repo(contents)
repo.index.add([path])
repo.index.commit('update lib')
origin = repo.remotes.origin
origin.push(ref)
```
这样就可以直接在 Python 中进行 Git 操作,避免使用 shell 命令时可能出现的问题。同时,使用 GitPython 提供的更高层次的接口,代码更加简洁易读。
改进以下代码 def push_lib(self,contents,path, ref): retcode = subprocess.call("git add {} && git commit -m 'update lib' && git push -u origin {} ".format(path, ref), shell=True, cwd=contents) return retcode
可以考虑进行以下改进:
1. 引入异常处理机制,以便在执行过程中出现错误时能够捕捉并进行相应的处理,避免程序崩溃。
2. 将 subprocess.call() 方法替换为 subprocess.Popen() 方法,以便能够获取子进程的输出信息,方便调试和错误处理。
3. 将 shell 参数设置为 False,避免在执行过程中出现潜在的安全漏洞。
改进后的代码如下:
```python
import subprocess
def push_lib(contents, path, ref):
try:
cmd = ["git", "add", path]
subprocess.Popen(cmd, cwd=contents, stdout=subprocess.PIPE, stderr=subprocess.PIPE).communicate()
cmd = ["git", "commit", "-m", "update lib"]
subprocess.Popen(cmd, cwd=contents, stdout=subprocess.PIPE, stderr=subprocess.PIPE).communicate()
cmd = ["git", "push", "-u", "origin", ref]
subprocess.Popen(cmd, cwd=contents, stdout=subprocess.PIPE, stderr=subprocess.PIPE).communicate()
return 0
except Exception as e:
print("Error: ", e)
return 1
```
注意:这里只是简单的改进,实际应用中还需要根据具体情况进行优化和完善。
阅读全文
相关推荐
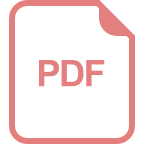
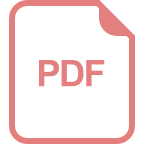
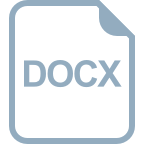














