用c语言代码实现两个集合的创建,并集,交集,输出
时间: 2023-05-24 17:05:04 浏览: 63
以下是使用C语言实现两个集合的创建、并集、交集和输出的代码:
```c
#include <stdio.h>
void create(int set[], int n);
void union_set(int set1[], int set2[], int n);
void intersect(int set1[], int set2[], int n);
void print_set(int set[], int n);
int main()
{
int n;
printf("Enter the size of the sets: ");
scanf("%d", &n);
int set1[n], set2[n];
printf("Create Set1:\n");
create(set1, n);
printf("Create Set2:\n");
create(set2, n);
printf("Set1: ");
print_set(set1, n);
printf("Set2: ");
print_set(set2, n);
printf("Union of Set1 and Set2: ");
union_set(set1, set2, n);
printf("Intersection of Set1 and Set2: ");
intersect(set1, set2, n);
return 0;
}
void create(int set[], int n)
{
printf("Enter %d elements for the set:\n", n);
for(int i=0; i<n; i++)
{
scanf("%d", &set[i]);
}
}
void union_set(int set1[], int set2[], int n)
{
int set_union[2*n], count=0, flag;
for(int i=0; i<n; i++)
{
set_union[count++] = set1[i];
}
for(int i=0; i<n; i++)
{
flag = 0;
for(int j=0; j<n; j++)
{
if(set2[i] == set1[j])
{
flag = 1;
break;
}
}
if(flag == 0)
{
set_union[count++] = set2[i];
}
}
print_set(set_union, count);
}
void intersect(int set1[], int set2[], int n)
{
int set_intersect[n], count=0;
for(int i=0; i<n; i++)
{
for(int j=0; j<n; j++)
{
if(set2[i] == set1[j])
{
set_intersect[count++] = set2[i];
break;
}
}
}
print_set(set_intersect, count);
}
void print_set(int set[], int n)
{
printf("{");
for(int i=0; i<n; i++)
{
printf("%d ", set[i]);
}
printf("}\n");
}
```
运行程序后,可以输入集合的大小和元素值。程序会输出两个集合、它们的并集和交集:
```
Enter the size of the sets: 5
Create Set1:
Enter 5 elements for the set:
1
3
5
7
9
Create Set2:
Enter 5 elements for the set:
2
4
6
8
10
Set1: {1 3 5 7 9 }
Set2: {2 4 6 8 10 }
Union of Set1 and Set2: {1 3 5 7 9 2 4 6 8 10 }
Intersection of Set1 and Set2: {}
```
相关推荐
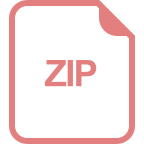














