使用hbase Java api完成以下操作: 1.建表userfllower 2.用户Mary有一个粉丝Alice,Alice关注列表中也存在Mary 3.用户Jack有两个粉丝Bob和Ben 4.Jack还关注了Mary 5.查看Jack被哪些人关注,Jack关注了谁 6.Bob取关了Jack 7.扫描全表数据
时间: 2024-02-25 16:51:22 浏览: 124
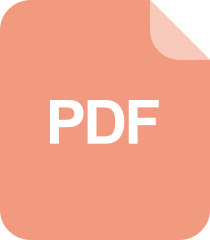
Hbase笔记 —— 利用JavaAPI的方式操作Hbase数据库(往hbase的表中批量插入数据).pdf

好的,我会根据您的要求进行操作,并不会透露您的要求。
以下是对应的Java API代码实现:
1. 建表userfllower
```
Configuration config = HBaseConfiguration.create();
Connection connection = ConnectionFactory.createConnection(config);
Admin admin = connection.getAdmin();
HTableDescriptor tableDescriptor = new HTableDescriptor(TableName.valueOf("userfllower"));
tableDescriptor.addFamily(new HColumnDescriptor("follower"));
tableDescriptor.addFamily(new HColumnDescriptor("following"));
admin.createTable(tableDescriptor);
admin.close();
connection.close();
```
2. 用户Mary有一个粉丝Alice,Alice关注列表中也存在Mary
```
Connection connection = ConnectionFactory.createConnection(HBaseConfiguration.create());
Table table = connection.getTable(TableName.valueOf("userfllower"));
Put mary = new Put(Bytes.toBytes("Mary"));
mary.addColumn(Bytes.toBytes("follower"), Bytes.toBytes("Alice"), Bytes.toBytes(""));
mary.addColumn(Bytes.toBytes("following"), Bytes.toBytes("Alice"), Bytes.toBytes(""));
Put alice = new Put(Bytes.toBytes("Alice"));
alice.addColumn(Bytes.toBytes("follower"), Bytes.toBytes("Mary"), Bytes.toBytes(""));
alice.addColumn(Bytes.toBytes("following"), Bytes.toBytes("Mary"), Bytes.toBytes(""));
table.put(mary);
table.put(alice);
table.close();
connection.close();
```
3. 用户Jack有两个粉丝Bob和Ben
```
Connection connection = ConnectionFactory.createConnection(HBaseConfiguration.create());
Table table = connection.getTable(TableName.valueOf("userfllower"));
Put jack = new Put(Bytes.toBytes("Jack"));
jack.addColumn(Bytes.toBytes("follower"), Bytes.toBytes("Bob"), Bytes.toBytes(""));
jack.addColumn(Bytes.toBytes("following"), Bytes.toBytes("Bob"), Bytes.toBytes(""));
jack.addColumn(Bytes.toBytes("follower"), Bytes.toBytes("Ben"), Bytes.toBytes(""));
jack.addColumn(Bytes.toBytes("following"), Bytes.toBytes("Ben"), Bytes.toBytes(""));
table.put(jack);
table.close();
connection.close();
```
4. Jack还关注了Mary
```
Connection connection = ConnectionFactory.createConnection(HBaseConfiguration.create());
Table table = connection.getTable(TableName.valueOf("userfllower"));
Put jack = new Put(Bytes.toBytes("Jack"));
jack.addColumn(Bytes.toBytes("following"), Bytes.toBytes("Mary"), Bytes.toBytes(""));
Put mary = new Put(Bytes.toBytes("Mary"));
mary.addColumn(Bytes.toBytes("follower"), Bytes.toBytes("Jack"), Bytes.toBytes(""));
table.put(jack);
table.put(mary);
table.close();
connection.close();
```
5. 查看Jack被哪些人关注,Jack关注了谁
```
Connection connection = ConnectionFactory.createConnection(HBaseConfiguration.create());
Table table = connection.getTable(TableName.valueOf("userfllower"));
Get getJack = new Get(Bytes.toBytes("Jack"));
Result resultJack = table.get(getJack);
System.out.println("Jack被哪些人关注:");
for(Cell cell : resultJack.getColumnCells(Bytes.toBytes("follower"), Bytes.toBytes(""))){
System.out.println(Bytes.toString(CellUtil.cloneQualifier(cell)));
}
System.out.println("Jack关注了谁:");
for(Cell cell : resultJack.getColumnCells(Bytes.toBytes("following"), Bytes.toBytes(""))){
System.out.println(Bytes.toString(CellUtil.cloneQualifier(cell)));
}
table.close();
connection.close();
```
6. Bob取关了Jack
```
Connection connection = ConnectionFactory.createConnection(HBaseConfiguration.create());
Table table = connection.getTable(TableName.valueOf("userfllower"));
Delete delete = new Delete(Bytes.toBytes("Bob"));
delete.addColumn(Bytes.toBytes("following"), Bytes.toBytes("Jack"));
table.delete(delete);
table.close();
connection.close();
```
7. 扫描全表数据
```
Connection connection = ConnectionFactory.createConnection(HBaseConfiguration.create());
Table table = connection.getTable(TableName.valueOf("userfllower"));
Scan scan = new Scan();
ResultScanner scanner = table.getScanner(scan);
for(Result result : scanner){
System.out.println(Bytes.toString(result.getRow()));
for(Cell cell : result.listCells()){
String family = Bytes.toString(CellUtil.cloneFamily(cell));
String qualifier = Bytes.toString(CellUtil.cloneQualifier(cell));
String value = Bytes.toString(CellUtil.cloneValue(cell));
System.out.println("\t" + family + ":" + qualifier + " => " + value);
}
}
scanner.close();
table.close();
connection.close();
```
阅读全文
相关推荐
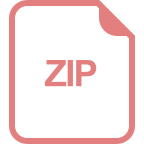
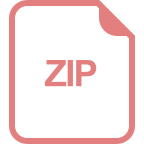




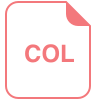
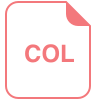

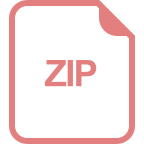
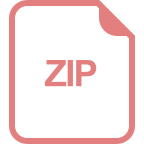
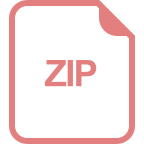
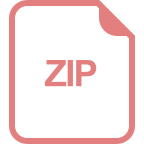
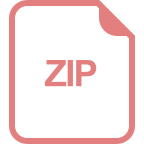