C# Vector3d转Vector3
时间: 2023-07-12 20:10:02 浏览: 74
C#中的Vector3d和Unity中的Vector3有些差别。如果你想将C#中的Vector3d转换为Unity中的Vector3,可以使用Vector3的构造函数来实现。你可以按照以下步骤进行转换:
1. 创建一个新的Vector3类型的变量,作为转换后的结果。
2. 使用Vector3的构造函数,将Vector3d类型的变量的x、y和z属性分别赋值给Vector3类型的变量的x、y和z属性。
3. 将新创建的Vector3类型的变量作为转换后的结果返回。
具体实现代码如下:
```csharp
Vector3d vector3d = new Vector3d(1.0, 2.0, 3.0);
Vector3 vector3 = new Vector3((float)vector3d.x, (float)vector3d.y, (float)vector3d.z);
```
在这个示例中,我们创建了一个Vector3d类型的变量vector3d,并将它的x、y和z属性分别设置为1.0、2.0和3.0。然后,我们使用Vector3的构造函数,将vector3d的x、y和z属性分别赋值给一个新创建的Vector3类型的变量vector3的x、y和z属性,最终得到一个转换后的Vector3类型的变量vector3。
相关问题
SatelliteController 中 Vector3d C#详细代码
以下是一个C#版本的 SatelliteController 类,其中包含一个 Vector3d 类,用于处理三维向量计算:
```
using System;
public class Vector3d {
public double x, y, z;
public Vector3d(double x = 0, double y = 0, double z = 0) {
this.x = x;
this.y = y;
this.z = z;
}
// 重载一些运算符,如 +, -, *, /, 等
public static Vector3d operator +(Vector3d v1, Vector3d v2) {
return new Vector3d(v1.x + v2.x, v1.y + v2.y, v1.z + v2.z);
}
public static Vector3d operator -(Vector3d v1, Vector3d v2) {
return new Vector3d(v1.x - v2.x, v1.y - v2.y, v1.z - v2.z);
}
public static Vector3d operator *(Vector3d v, double k) {
return new Vector3d(v.x * k, v.y * k, v.z * k);
}
public static Vector3d operator /(Vector3d v, double k) {
return new Vector3d(v.x / k, v.y / k, v.z / k);
}
public Vector3d Add(Vector3d v) {
x += v.x;
y += v.y;
z += v.z;
return this;
}
public Vector3d Subtract(Vector3d v) {
x -= v.x;
y -= v.y;
z -= v.z;
return this;
}
public Vector3d Multiply(double k) {
x *= k;
y *= k;
z *= k;
return this;
}
public Vector3d Divide(double k) {
x /= k;
y /= k;
z /= k;
return this;
}
// 计算向量长度
public double Length() {
return Math.Sqrt(x * x + y * y + z * z);
}
// 单位化向量
public void Normalize() {
double len = Length();
if (len > 0) {
x /= len;
y /= len;
z /= len;
}
}
}
public class SatelliteController {
// 卫星当前位置和速度
private Vector3d position;
private Vector3d velocity;
// 卫星质量和引力常数
private double mass;
private double G;
public SatelliteController(double mass = 1000, double G = 6.674e-11) {
this.mass = mass;
this.G = G;
}
// 获取卫星当前位置
public Vector3d GetPosition() {
return position;
}
// 获取卫星当前速度
public Vector3d GetVelocity() {
return velocity;
}
// 设置卫星当前位置和速度
public void SetState(Vector3d position, Vector3d velocity) {
this.position = position;
this.velocity = velocity;
}
// 计算卫星所受的重力加速度
public Vector3d CalcGravityAcc(Vector3d planetPosition, double planetMass) {
Vector3d delta = planetPosition.Subtract(position);
double dist = delta.Length();
double force = G * mass * planetMass / (dist * dist);
Vector3d acc = delta.Multiply(force / dist / mass);
return acc;
}
// 计算卫星的轨道,简单起见,不考虑行星的运动,假设行星在 (0, 0, 0)
public void CalcOrbit(double dt, int steps) {
const double planetMass = 5.97e24; // 地球质量
Vector3d planetPosition = new Vector3d(0, 0, 0);
Vector3d acc = new Vector3d(0, 0, 0);
for (int i = 0; i < steps; i++) {
acc = CalcGravityAcc(planetPosition, planetMass);
velocity = velocity.Add(acc.Multiply(dt));
position = position.Add(velocity.Multiply(dt));
}
}
}
```
在上述代码中,Vector3d 类用于表示三维向量,它包含了向量加减、数乘、数除、长度计算、单位化等基本操作,同时 SatelliteController 类中也用到了这些操作,计算卫星的轨道。
vector3和vector3d的区别
Vector3和Vector3D都是表示三维向量的类,它们之间的区别在于精度和使用场景。
Vector3是Unity中的一个类,使用float类型表示向量的三个分量,适用于游戏中的大多数场景,例如物体的移动、旋转等。由于使用float类型,所以Vector3的精度相对较低,适合处理相对简单的运算。
Vector3D是C#中的一个类,使用double类型表示向量的三个分量,适用于需要高精度计算的场景,例如科学计算、CAD等领域。由于使用double类型,所以Vector3D的精度相对更高,但同时也会占用更多的内存和计算资源。
总之,如果你需要进行精度要求不高的三维向量计算,可以使用Vector3;如果需要高精度计算或需要处理复杂的三维向量运算,可以使用Vector3D。
相关推荐
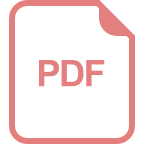












