用c++写一个用差分进化算法拟合分段连续线性函数的示例
时间: 2024-05-03 08:18:41 浏览: 178
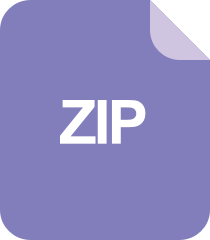
差分进化 算法的c++实现

好的,以下是一个简单的用差分进化算法拟合分段连续线性函数的示例(仅供参考):
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <cmath>
#include <random>
using namespace std;
// 定义函数类型为分段连续线性函数
typedef double (*piecewise_linear_function)(double, const vector<double>&);
double piecewise_linear(double x, const vector<double>& params) {
double result = 0.0;
int n = params.size();
for (int i = 0; i < n; i += 3) {
if (x <= params[i]) {
result += params[i+1];
break;
}
else if (i+3 == n) {
result += params[i+2];
break;
}
else {
double slope = (params[i+2] - params[i+1]) / (params[i+3] - params[i]);
result += slope * (x - params[i]) + params[i+1];
}
}
return result;
}
// 定义差分进化算法的参数结构体
struct DE_params {
int pop_size; // 种群大小
int max_gen; // 最大迭代次数
double F; // 缩放因子
double CR; // 交叉概率
};
// 差分进化算法
vector<double> differential_evolution(piecewise_linear_function f, const vector<double>& x_lower, const vector<double>& x_upper, const DE_params& params) {
int n = x_lower.size();
vector<vector<double>> population(params.pop_size, vector<double>(n));
vector<double> fitness(params.pop_size);
vector<double> best_individual(n);
double best_fitness = numeric_limits<double>::max();
mt19937 rng(random_device{}());
uniform_real_distribution<double> uni(0, 1);
// 初始化种群
for (int i = 0; i < params.pop_size; ++i) {
for (int j = 0; j < n; ++j) {
population[i][j] = x_lower[j] + uni(rng) * (x_upper[j] - x_lower[j]);
}
fitness[i] = f(population[i][0], population[i]);
if (fitness[i] < best_fitness) {
best_individual = population[i];
best_fitness = fitness[i];
}
}
// 迭代优化
for (int gen = 0; gen < params.max_gen; ++gen) {
for (int i = 0; i < params.pop_size; ++i) {
// 选择三个不同的个体
int r1, r2, r3;
do {
r1 = uni(rng) * params.pop_size;
} while (r1 == i);
do {
r2 = uni(rng) * params.pop_size;
} while (r2 == i || r2 == r1);
do {
r3 = uni(rng) * params.pop_size;
} while (r3 == i || r3 == r1 || r3 == r2);
// 变异操作
vector<double> mutant(n);
for (int j = 0; j < n; ++j) {
mutant[j] = population[r1][j] + params.F * (population[r2][j] - population[r3][j]);
mutant[j] = max(x_lower[j], min(x_upper[j], mutant[j])); // 判断是否越界
}
// 交叉操作
vector<double> trial(n);
int j_rand = uni(rng) * n;
for (int j = 0; j < n; ++j) {
if (uni(rng) < params.CR || j == j_rand) {
trial[j] = mutant[j];
}
else {
trial[j] = population[i][j];
}
}
// 评估个体适应度
double trial_fitness = f(trial[0], trial);
if (trial_fitness < fitness[i]) {
population[i] = trial;
fitness[i] = trial_fitness;
if (trial_fitness < best_fitness) {
best_individual = trial;
best_fitness = trial_fitness;
}
}
}
}
return best_individual;
}
int main() {
// 示例使用的分段连续线性函数为 f(x) = 2x + 1 (x <= 0) 和 f(x) = -3x + 2 (x > 0)
piecewise_linear_function f = [](double x, const vector<double>& params) -> double {
if (x <= 0) {
return params[0] * x + params[1];
}
else {
return params[2] * x + params[3];
}
};
// 定义差分进化算法的参数
DE_params params;
params.pop_size = 50;
params.max_gen = 100;
params.F = 0.5;
params.CR = 0.9;
// 定义变量的取值范围
vector<double> x_lower = {-1.0, -1.0, -1.0, -1.0};
vector<double> x_upper = {1.0, 1.0, 1.0, 1.0};
// 用差分进化算法拟合分段连续线性函数
vector<double> best_params = differential_evolution(f, x_lower, x_upper, params);
// 输出结果
cout << "f(x) = ";
if (best_params[0] < 0) {
cout << best_params[0] << "x";
}
else {
cout << "+" << best_params[0] << "x";
}
if (best_params[1] < 0) {
cout << best_params[1];
}
else {
cout << "+" << best_params[1];
}
cout << " (x <= 0)" << endl;
cout << "f(x) = ";
if (best_params[2] < 0) {
cout << best_params[2] << "x";
}
else {
cout << "+" << best_params[2] << "x";
}
if (best_params[3] < 0) {
cout << best_params[3];
}
else {
cout << "+" << best_params[3];
}
cout << " (x > 0)" << endl;
return 0;
}
```
这个示例程序中,我们定义了一个 piecewise_linear_function 类型,以便于传递分段连续线性函数;并且定义了一个差分进化算法的参数结构体 DE_params,用于传递算法参数。在 differential_evolution 函数中,我们使用了 vector<vector<double>> 类型来存储种群,vector<double> 类型来存储个体,其中个体的第一个元素表示分段点,其他元素表示线性函数的参数。在主函数中,我们使用了一个 lambda 表达式来定义分段连续线性函数,并且指定了变量的取值范围。最后,我们用 differential_evolution 函数拟合了这个分段连续线性函数,并输出了拟合结果。
阅读全文
相关推荐
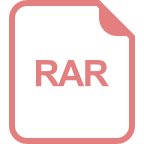
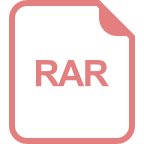
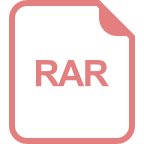
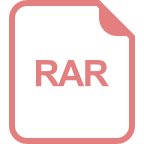
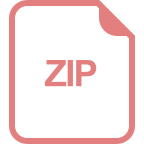
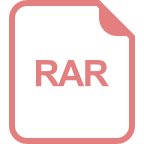
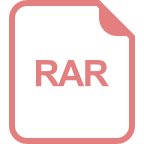
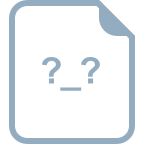
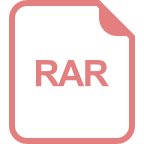
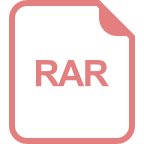
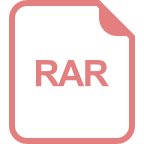
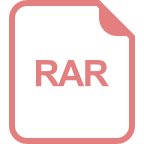
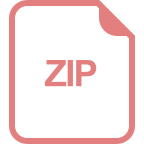
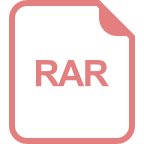
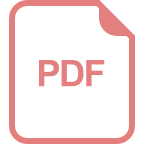
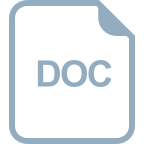
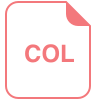