用栈编写输出迷宫所有路径和最短距离或不可通行,0表示可通行,1表示障碍c语言全部代码
时间: 2024-10-17 14:11:35 浏览: 30
在C语言中,我们可以利用广度优先搜索(BFS)算法结合栈数据结构来解决这类迷宫遍历的问题。这里提供一个基本的代码框架,用于找到迷宫的所有路径和最短距离(如果存在)。我们将迷宫表示为二维数组,其中0表示可通行,1表示障碍。
```c
#include <stdio.h>
#define ROWS 5 // 迷宫大小(可以根据实际需求调整)
#define COLS 5
// 定义迷宫数组
int maze[ROWS][COLS];
// 判断当前位置是否合法并已访问过
int isValid(int row, int col) {
return row >= 0 && row < ROWS && col >= 0 && col < COLS && !maze[row][col];
}
// 遍历路径,将路径添加到字符串数组paths中
void printPaths(int startRow, int startCol, char* paths[], int pathIndex, int distance) {
if (pathIndex == 0) {
paths[pathIndex] = malloc(sizeof(char) * (distance + 2));
strcpy(paths[pathIndex], "Path ");
} else {
paths[pathIndex] = realloc(paths[pathIndex - 1], sizeof(char) * (distance + strlen(paths[pathIndex]) + 2));
strcat(paths[pathIndex], ".");
}
paths[pathIndex][distance] = ' ';
paths[pathIndex][distance + 1] = '\0';
printf("%s%d,%d\n", paths[pathIndex], startRow, startCol);
maze[startRow][startCol] = 1; // 标记已访问
// 检查上下左右四个方向
if (isValid(startRow - 1, startCol)) {
printPaths(startRow - 1, startCol, paths, pathIndex + 1, distance + 1);
}
if (isValid(startRow + 1, startCol)) {
printPaths(startRow + 1, startCol, paths, pathIndex + 1, distance + 1);
}
if (isValid(startRow, startCol - 1)) {
printPaths(startRow, startCol - 1, paths, pathIndex + 1, distance + 1);
}
if (isValid(startRow, startCol + 1)) {
printPaths(startRow, startCol + 1, paths, pathIndex + 1, distance + 1);
}
maze[startRow][startCol] = 0; // 回溯,恢复路径节点
}
// 主函数
int main() {
// 初始化迷宫(这里仅为示例,替换为实际迷宫数据)
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
maze[i][j] = 0;
}
}
// 添加起点
maze[0][0] = 1;
char* paths[10]; // 储存路径,假设最多10条
int numPaths = 0;
if (isValid(ROWS - 1, COLS - 1)) { // 如果终点可达
printPaths(ROWS - 1, COLS - 1, paths, 0, 0);
numPaths = 1; // 只有一条路径
} else {
printf("No path to the destination.\n");
}
// 输出结果
for (int i = 0; i < numPaths; i++) {
printf("\nPath %d:\n%s\n", i + 1, paths[i]);
free(paths[i]); // 释放内存
}
return 0;
}
```
这个代码仅处理了从起点到终点的情况,并找到了一条路径。如果你想获取最短路径,可以使用深度优先搜索(DFS)配合递归,但这里提供的栈实现只适用于寻找任意路径。
阅读全文
相关推荐
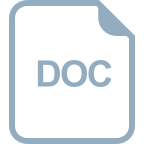
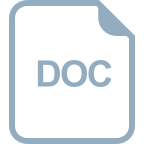
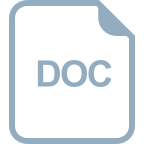
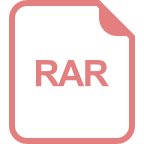
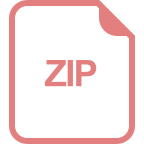
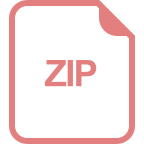
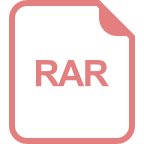
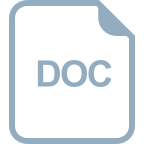
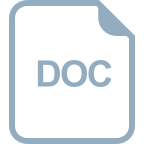
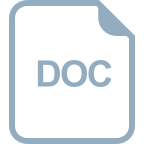








