ground_points = (cloud.select_by_index(ground_idx)) return ground_points
时间: 2024-04-06 22:32:00 浏览: 15
这段代码的作用是从给定的点云数据 `cloud` 中,根据索引数组 `ground_idx` 提取出地面点,然后返回这些地面点的数据。
其中,`cloud` 是一个点云对象,可以包含多个点,每个点包含 x、y、z 等属性。`ground_idx` 是一个索引数组,表示在 `cloud` 点云数据中,哪些点属于地面点。这个索引数组可以通过一些算法或手动标注得到。
代码中,`select_by_index()` 是 Earth Engine API 提供的函数,用于根据给定的索引数组从一个 ImageCollection 或 FeatureCollection 中选择数据。在这里,`cloud` 是一个 FeatureCollection,`select_by_index(ground_idx)` 的作用是根据 `ground_idx` 数组从中选择对应的数据,即地面点数据,返回一个新的 FeatureCollection 对象 `ground_points`。
相关问题
cloud = o3d.io.read_point_cloud("Model.pcd") Delta = 0.01 dPlatform = 0.3 point_cloud = np.asarray(cloud.points) _, _, z_min = np.amin(point_cloud, axis=0) idx = [] for i in range(len(point_cloud)): index = np.floor((point_cloud[i][2] - z_min) / dPlatform) sliceMin = z_min + index * dPlatform if sliceMin <= point_cloud[i][2] < sliceMin + Delta: idx.append(i) slicing_cloud = (cloud.select_by_index(idx))这段代码获取了几个点云切片,如何改可以单独获得每个切片
可以尝试将获取切片的部分放在一个循环内,每次循环获取一个切片,将其存储到一个列表中。具体代码如下:
```
cloud = o3d.io.read_point_cloud("Model.pcd")
Delta = 0.01
dPlatform = 0.3
point_cloud = np.asarray(cloud.points)
_, _, z_min = np.amin(point_cloud, axis=0)
slicing_clouds = [] # 存储每个切片的列表
for index in range(int((np.max(point_cloud[:,2]) - z_min) / dPlatform)): # 遍历每个切片
idx = []
sliceMin = z_min + index * dPlatform # 当前切片的最小高度
for i in range(len(point_cloud)): # 获取当前切片中的点
if sliceMin <= point_cloud[i][2] < sliceMin + Delta:
idx.append(i)
slicing_clouds.append(cloud.select_by_index(idx)) # 将当前切片存储到列表中
```
这样,`slicing_clouds` 列表中的每个元素就是一个单独的切片点云了。可以通过 `slicing_clouds[i]` 获取第 i 个切片的点云。
优化以下SQL使其查询速度更快SELECT P.LOT_NO UNIT_ID, '0' AS UNIT_TYPE, '宗地' AS UNIT_TYPE_DES, NVL(BD.PARCEL_CODE_TMP, P.LOT_NO || P.SITE) UNIT_CODE, P.PARCEL_NO, P.LOC_CANTON, BD.REAL_UNIT_NO AS UNIT_CODE_GB, P.LU_LOCATION, P.SITE_KEY, P.CHG_TYPE, P.LU_AREA AS UNIT_AREA, P.PARCEL_CODE FROM V_LD_PARCEL P LEFT JOIN V_BD_PARCEL BD ON BD.LOT_NO = P.LOT_NO where 2 > 1 and not exists (select 1 from v_bk_reg_idx idx where idx.reg_unit_code = p.lot_no and idx.reg_unit_type = '0' and idx.buss_reg_type = '1101' and (idx.status_book = '1' or idx.status_book = '9')) and not exists(select 1 from v_bk_reg_idx idx where (idx.reg_unit_code, idx.reg_unit_type) in (select rise_id, '1' from bd_rise br, bd_parcel bp where br.par_lot_no = bp.lot_no and bp.lot_no = p.lot_no) and idx.buss_reg_type = '1200') and p.parcel_no = ?
首先,可以看到这个SQL语句中有两个子查询。这些子查询可能会导致性能问题,因此可以尝试优化它们。
以下是可能的优化措施:
1. 将子查询转换为JOIN语句
将第一个子查询转换为JOIN语句,可以避免使用NOT EXISTS,从而提高性能。例如:
```
SELECT P.LOT_NO UNIT_ID,
'0' AS UNIT_TYPE,
'宗地' AS UNIT_TYPE_DES,
NVL(BD.PARCEL_CODE_TMP, P.LOT_NO || P.SITE) UNIT_CODE,
P.PARCEL_NO,
P.LOC_CANTON,
BD.REAL_UNIT_NO AS UNIT_CODE_GB,
P.LU_LOCATION,
P.SITE_KEY,
P.CHG_TYPE,
P.LU_AREA AS UNIT_AREA,
P.PARCEL_CODE
FROM V_LD_PARCEL P
LEFT JOIN V_BD_PARCEL BD ON BD.LOT_NO = P.LOT_NO
LEFT JOIN v_bk_reg_idx idx ON idx.reg_unit_code = p.lot_no
AND idx.reg_unit_type = '0'
AND idx.buss_reg_type = '1101'
AND (idx.status_book = '1' OR idx.status_book = '9')
WHERE 2 > 1
AND idx.reg_unit_code IS NULL
AND P.PARCEL_NO = ?
```
同样,将第二个子查询转换为JOIN语句也可以提高性能:
```
SELECT P.LOT_NO UNIT_ID,
'0' AS UNIT_TYPE,
'宗地' AS UNIT_TYPE_DES,
NVL(BD.PARCEL_CODE_TMP, P.LOT_NO || P.SITE) UNIT_CODE,
P.PARCEL_NO,
P.LOC_CANTON,
BD.REAL_UNIT_NO AS UNIT_CODE_GB,
P.LU_LOCATION,
P.SITE_KEY,
P.CHG_TYPE,
P.LU_AREA AS UNIT_AREA,
P.PARCEL_CODE
FROM V_LD_PARCEL P
LEFT JOIN V_BD_PARCEL BD ON BD.LOT_NO = P.LOT_NO
LEFT JOIN v_bk_reg_idx idx ON idx.reg_unit_code = p.lot_no
AND idx.reg_unit_type = '0'
AND idx.buss_reg_type = '1101'
AND (idx.status_book = '1' OR idx.status_book = '9')
LEFT JOIN v_bk_reg_idx idx2 ON idx2.reg_unit_code = (SELECT rise_id FROM bd_rise br, bd_parcel bp WHERE br.par_lot_no = bp.lot_no AND bp.lot_no = p.lot_no)
AND idx2.reg_unit_type = '1'
AND idx2.buss_reg_type = '1200'
WHERE 2 > 1
AND idx.reg_unit_code IS NULL
AND idx2.reg_unit_code IS NULL
AND P.PARCEL_NO = ?
```
2. 添加索引
为V_LD_PARCEL和V_BD_PARCEL表添加适当的索引,可以加速JOIN操作。例如:
```
CREATE INDEX idx_v_ld_parcel_parcel_no ON V_LD_PARCEL (PARCEL_NO);
CREATE INDEX idx_v_bd_parcel_lot_no ON V_BD_PARCEL (LOT_NO);
```
3. 减少查询返回的行数
如果查询返回的行数非常大,可以考虑分页或者限制返回的行数。这可以减少查询的执行时间。例如,可以添加以下子句来限制返回的行数:
```
WHERE ROWNUM <= 100
```
相关推荐
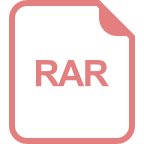
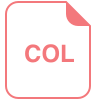












