query_idx = self.input_trees[split][cloud_idx].query(pick_point, k=cfg.num_points)[1][0]
时间: 2023-12-24 18:03:09 浏览: 104
This code snippet is using the input trees stored in the object to find the index of the query point closest to a given pick point.
`self.input_trees` is a list of KDTree objects, where each KDTree corresponds to a point cloud in the dataset. `split` is an integer index indicating which point cloud in the dataset to use. `cloud_idx` is the index of the query point within the selected point cloud.
The `query()` method of the KDTree object is then used to find the indices of the `k` (in this case, `cfg.num_points`) nearest neighbors of the pick point. The `[1][0]` at the end of the line is used to extract the index of the nearest neighbor (since `query()` returns a tuple containing both the distance and index of each neighbor).
Overall, this code is finding the index of the nearest neighbor to a given point in a particular point cloud in the dataset.
阅读全文
相关推荐
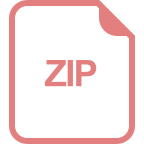
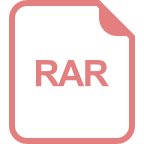















