python查找txt中的数字,并替换为(1284, 533),请给出代码
时间: 2023-09-16 13:05:55 浏览: 94
### 回答1:
以下是使用Python读取txt文件中的数字并替换为(1284, 533)的代码:
```python
# 打开文件
with open('filename.txt', 'r') as f:
# 读取文件内容
content = f.read()
# 使用正则表达式查找所有的数字
import re
nums = re.findall(r'\d+', content)
# 将每个数字替换为(1284, 533)
for num in nums:
content = content.replace(num, '(1284, 533)')
# 写入新的内容
with open('filename.txt', 'w') as f:
f.write(content)
```
说明:
1. 将文件名替换为你要处理的文件名。
2. 该代码使用正则表达式(r'\d+')来查找所有数字,将它们保存在nums列表中。
3. 遍历nums列表,将每个数字替换为"(1284, 533)"。
4. 将修改后的文本重新写回到原始文件中。
### 回答2:
你好!以下是使用Python来查找txt文件中的数字,并替换为(1284, 533)的代码:
```python
import re
def replace_numbers(file_path):
try:
# 打开txt文件
with open(file_path, 'r') as file:
# 读取文件内容
contents = file.read()
# 使用正则表达式查找所有数字
numbers = re.findall(r'\d+', contents)
# 将所有数字替换为(1284, 533)
for number in numbers:
contents = contents.replace(number, '(1284, 533)')
# 保存替换后的内容回txt文件
with open(file_path, 'w') as file:
file.write(contents)
print("数字替换成功!")
except IOError:
print("打开文件出错!")
# 调用函数,传入txt文件的路径
replace_numbers('example.txt')
```
以上代码使用了正则表达式来查找txt文件中的所有数字,并使用`replace`方法将其替换为`(1284, 533)`。请替换`example.txt`为你实际的txt文件路径,并确保文件存在。这段代码会将替换后的内容保存回原始txt文件中。如果你需要保留原始内容,可以将保存文件的代码段注释掉,然后将`contents`打印出来查看替换后的结果。
希望对你有帮助!如果有任何问题,请随时向我提问。
### 回答3:
下面是一个使用Python查找txt文件中的数字,并替换为(1284, 533)的示例代码:
```python
def replace_numbers(file_path):
# 打开文件
with open(file_path, 'r') as file:
# 读取文件内容
content = file.read()
# 使用正则表达式查找所有数字
import re
numbers = re.findall(r'\d+', content)
# 将数字替换为(1284, 533)
for num in numbers:
content = content.replace(num, '(1284, 533)')
# 将替换后的内容写入文件
with open(file_path, 'w') as file:
file.write(content)
# 调用函数进行替换
replace_numbers('test.txt')
```
上述代码中,首先通过使用正则表达式`re.findall(r'\d+', content)`查找文本文件中的所有数字,并将其保存到一个列表中。
然后,使用`replace()`方法将每个数字替换为"(1284, 533)"。最后,将替换后的内容写回到文件中。
需要注意,代码中的`'test.txt'`是要处理的txt文件的路径,需根据实际文件路径进行相应的修改。
阅读全文
相关推荐




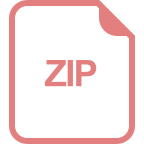
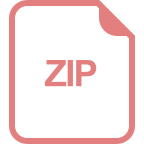
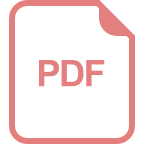
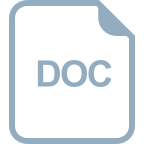
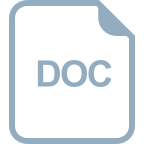
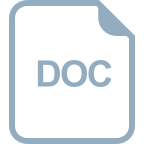
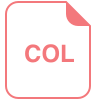
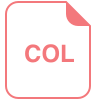



