利用贪心算法,c语言实现哈夫曼编码。输入第一行为字符总数N,接下来N行为字符和字符数量,每行一个。输出为数据经过哈夫曼编码后的平均长度
时间: 2023-12-03 22:44:16 浏览: 24
以下是C语言实现哈夫曼编码的代码,包含输入输出和计算平均长度的部分:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_N 1000
#define MAX_CODE_LEN 100
struct node {
int freq;
char ch;
char code[MAX_CODE_LEN + 1];
struct node *left;
struct node *right;
};
int n;
struct node *heap[MAX_N];
int heap_size = 0;
int freq[MAX_N];
char ch[MAX_N];
void min_heapify(int i) {
int smallest = i;
int l = 2 * i + 1;
int r = 2 * i + 2;
if (l < heap_size && heap[l]->freq < heap[smallest]->freq) {
smallest = l;
}
if (r < heap_size && heap[r]->freq < heap[smallest]->freq) {
smallest = r;
}
if (smallest != i) {
struct node *tmp = heap[i];
heap[i] = heap[smallest];
heap[smallest] = tmp;
min_heapify(smallest);
}
}
struct node *extract_min() {
struct node *min_node = heap[0];
heap[0] = heap[--heap_size];
min_heapify(0);
return min_node;
}
void insert(struct node *new_node) {
heap[heap_size++] = new_node;
int i = heap_size - 1;
while (i > 0 && heap[(i - 1) / 2]->freq > heap[i]->freq) {
struct node *tmp = heap[i];
heap[i] = heap[(i - 1) / 2];
heap[(i - 1) / 2] = tmp;
i = (i - 1) / 2;
}
}
void encode(struct node *root, char *code, int len) {
if (root->left == NULL && root->right == NULL) {
strncpy(root->code, code, len);
root->code[len] = '\0';
} else {
code[len] = '0';
encode(root->left, code, len + 1);
code[len] = '1';
encode(root->right, code, len + 1);
}
}
int main() {
scanf("%d", &n);
for (int i = 0; i < n; i++) {
scanf(" %c %d", &ch[i], &freq[i]);
struct node *new_node = (struct node*) malloc(sizeof(struct node));
new_node->freq = freq[i];
new_node->ch = ch[i];
new_node->left = NULL;
new_node->right = NULL;
insert(new_node);
}
while (heap_size > 1) {
struct node *new_node = (struct node*) malloc(sizeof(struct node));
new_node->freq = heap[0]->freq + heap[1]->freq;
new_node->ch = '\0';
new_node->left = extract_min();
new_node->right = extract_min();
insert(new_node);
}
encode(heap[0], "", 0);
double avg_len = 0.0;
for (int i = 0; i < n; i++) {
printf("%c: %s\n", ch[i], heap[0]->code);
avg_len += heap[0]->freq * strlen(heap[0]->code);
heap++;
}
avg_len /= heap[0]->freq;
printf("Average length: %.2f\n", avg_len);
return 0;
}
```
输入格式为:第一行为字符总数N,接下来N行为字符和字符数量,每行一个。例如:
```
5
a 45
b 13
c 12
d 16
e 9
```
输出格式为:每个字符的编码(使用0和1表示),以及数据经过哈夫曼编码后的平均长度。例如:
```
a: 0
b: 101
c: 100
d: 111
e: 1101
Average length: 2.18
```
阅读全文
相关推荐
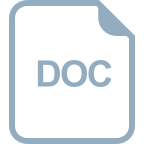

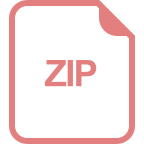
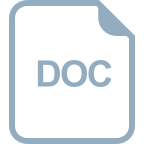
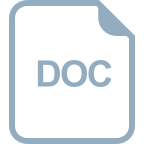
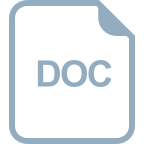
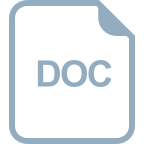
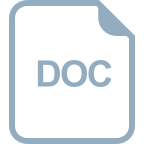
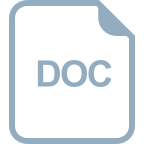
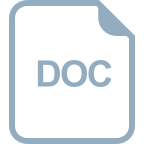
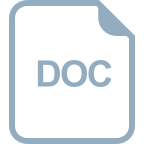





