pytest框架中的assert()
时间: 2024-09-11 09:02:39 浏览: 46
pytest是一个非常流行的Python测试框架,它提供了一种简洁的断言方式。在pytest中,`assert`关键字用于验证代码中期望的条件是否满足。当`assert`后的条件为`True`时,测试通过;如果条件为`False`,则测试失败,并显示失败的原因。
使用pytest的`assert`断言有以下特点:
1. 易用性:可以像在Python标准的`assert`语句中那样直接使用,不需要引入任何额外的断言库。
2. 可配置:pytest提供了丰富的配置选项,可以控制测试运行时的行为,比如自动收集测试、显示详细的失败信息等。
3. 插件支持:pytest有丰富的插件生态,可以扩展其功能,比如使用`pytest-assume`等第三方插件来增强断言的灵活性。
以下是一个简单的例子来展示如何在pytest中使用`assert`:
```python
def test_example():
assert 2 + 2 == 4 # 这是一个简单的断言,如果条件为False则测试失败
```
在上面的例子中,如果`2 + 2 == 4`条件为`True`,那么测试会通过;如果条件为`False`,则测试失败,并且会报告哪个断言失败,失败的具体情况。
相关问题
pytest框架中的attrib函数
pytest框架中的`@pytest.mark`装饰器配合`attr`函数(注意不是attrib,可能是您记错了),用于为测试用例添加标记(marker)。这些标记可以用于识别测试用例的特定属性,也可以用于在执行测试时通过命令行选择性地运行或跳过标记的测试。
使用`@pytest.mark.attr`的示例代码如下:
```python
import pytest
# 使用@pytest.mark.attr来标记测试函数
@pytest.mark.attr("core")
def test_core_functionality():
assert some_core_function() == "expected result"
# 使用@pytest.mark.attr来标记另一个测试函数
@pytest.mark.attr("extended")
def test_extended_functionality():
assert some_extended_function() == "expected result"
```
在上面的例子中,`test_core_functionality`函数被标记为“core”,`test_extended_functionality`函数被标记为“extended”。这意味着,如果我们想运行所有标记为“core”的测试,可以在命令行中使用如下命令:
```
pytest -v -m "attr=='core'"
```
其中`-v`代表详细模式,`-m "attr=='core'"`是使用标记来筛选测试用例。
pytest框架中,如何使用各类装饰器
在Pytest框架中,我们可以使用各种装饰器来扩展和自定义测试行为。下面是一些常用的装饰器及其用法示例:
1. `@pytest.fixture`:用于创建测试夹具(fixtures),它们可以提供测试数据或设置测试环境。
```python
import pytest
@pytest.fixture
def setup():
# 设置测试环境
print("Setup")
def test_example(setup):
# 使用setup夹具的测试代码
print("Running test")
```
2. `@pytest.mark.parametrize`:用于参数化测试,允许在多个输入值上运行同一个测试函数。
```python
import pytest
@pytest.mark.parametrize("input, expected", [(1, 2), (3, 6), (4, 8)])
def test_multiply(input, expected):
result = multiply_by_two(input)
assert result == expected
```
3. `@pytest.mark.skip`:用于跳过某个测试函数。
```python
import pytest
@pytest.mark.skip(reason="Test is not ready yet")
def test_function():
# 测试代码
assert 1 + 1 == 2
```
4. `@pytest.mark.xfail`:用于标记预期失败的测试用例。
```python
import pytest
@pytest.mark.xfail
def test_divide():
result = divide(10, 0)
assert result == 5
```
5. `@pytest.mark.timeout`:用于设置测试运行的最大时间限制。
```python
import pytest
import time
@pytest.mark.timeout(5) # 5秒超时
def test_function():
time.sleep(10)
assert 1 + 1 == 2
```
这些只是一些常用的装饰器,Pytest框架还提供了许多其他装饰器和功能,可以根据具体需求进行查阅和使用。
阅读全文
相关推荐
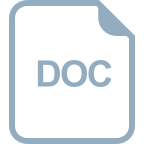
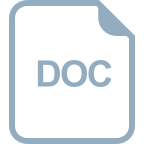
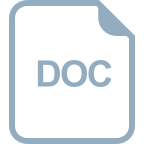
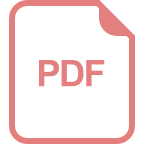












