在Qt中,怎么在子线程中创建 QSerialPort?
时间: 2024-10-27 14:09:41 浏览: 26
在Qt中,要在子线程中创建QSerialPort实例,你需要遵守一些规则来确保UI线程和非UI线程之间的通信不会阻塞主线程。通常的做法是使用`QThread`和信号槽机制。以下是步骤:
1. 创建一个继承自`QObject`的子类,并将`QSerialPort`作为成员变量,以便在子线程中访问它。
```cpp
class SerialPortThread : public QObject
{
Q_OBJECT
private:
QSerialPort *serialPort;
public:
explicit SerialPortThread(QObject *parent = nullptr)
: QObject(parent), serialPort(new QSerialPort(this)) {}
// ...其他方法...
signals:
void portOpened();
void portError(QSerialPort::SerialPortError error);
};
```
2. 在子线程中初始化`QSerialPort`,然后发出信号通知主线程状态变化。
```cpp
void SerialPortThread::initializePort(const QString &portName)
{
if (!serialPort->open(QIODevice::ReadWrite)) {
emit portError(serialPort->error());
return;
}
emit portOpened(); // 发出信号,主线程可以接收到并处理
}
```
3. 在主线程中,通过连接信号到槽来响应子线程的状态变化。
```cpp
// 在主线程的UI设置里
SerialPortThread *thread = new SerialPortThread(this);
connect(thread, &SerialPortThread::portOpened, this, []() { qDebug() << "Serial port opened"; });
connect(thread, &SerialPortThread::portError, this, [this](QSerialPort::SerialPortError error) {
qWarning() << "Serial port error: " << error.toString();
});
thread->moveToThread(&thread); // 将线程放入单独的线程
thread->start(); // 启动子线程
```
阅读全文
相关推荐
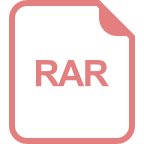
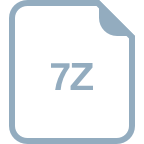
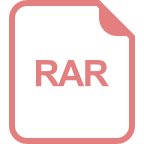


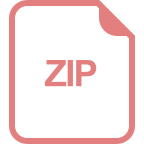
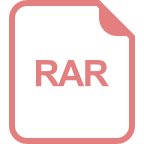
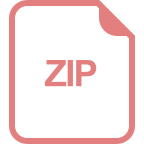
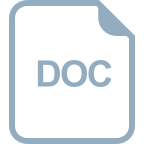
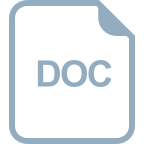
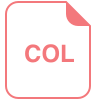
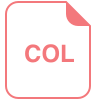
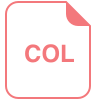
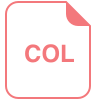




