c++用类实现俄罗斯方块c++c++
时间: 2024-01-08 14:03:37 浏览: 120
以下是使用C++类实现俄罗斯方块的示例代码:
```c++
#include <iostream>
#include <ncurses.h>
#include <cstdlib>
#include <ctime>
using namespace std;
class Tetris {
private:
int x, y; // 方块的坐标
int shape; // 方块的形状
int color; // 方块的颜色
int board[20][10]; // 游戏面板
int score; // 得分
int speed; // 下落速度
public:
Tetris() {
x = 0;
y = 4;
shape = rand() % 7;
color = rand() % 7 + 1;
score = 0;
speed = 500;
for (int i = 0; i < 20; i++) {
for (int j = 0; j < 10; j++) {
board[i][j] = 0;
}
}
}
void draw() {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if ((1 << (i * 4 + j)) & shapes[shape]) {
attron(COLOR_PAIR(color));
mvprintw(x + i, y + j * 2, "[]");
attroff(COLOR_PAIR(color));
}
}
}
}
void erase() {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if ((1 << (i * 4 + j)) & shapes[shape]) {
mvprintw(x + i, y + j * 2, " ");
}
}
}
}
bool can_move(int dx, int dy, int dshape) {
int nx = x + dx;
int ny = y + dy;
int nshape = (shape + dshape) % 4;
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if ((1 << (i * 4 + j)) & shapes[nshape]) {
int tx = nx + i;
int ty = ny + j;
if (tx < 0 || tx >= 20 || ty < 0 || ty >= 10 || board[tx][ty]) {
return false;
}
}
}
}
return true;
}
void move(int dx, int dy, int dshape) {
erase();
x += dx;
y += dy;
shape = (shape + dshape) % 4;
draw();
}
void fix() {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if ((1 << (i * 4 + j)) & shapes[shape]) {
board[x + i][y + j] = color;
}
}
}
}
bool can_clear(int row) {
for (int j = 0; j < 10; j++) {
if (!board[row][j]) {
return false;
}
}
return true;
}
void clear(int row) {
for (int i = row; i > 0; i--) {
for (int j = 0; j < 10; j++) {
board[i][j] = board[i - 1][j];
}
}
for (int j = 0; j < 10; j++) {
board[0][j] = 0;
}
score += 10;
if (score % 100 == 0) {
speed -= 50;
}
}
bool game_over() {
for (int j = 0; j < 10; j++) {
if (board[0][j]) {
return true;
}
}
return false;
}
void play() {
initscr();
start_color();
init_pair(1, COLOR_RED, COLOR_BLACK);
init_pair(2, COLOR_GREEN, COLOR_BLACK);
init_pair(3, COLOR_YELLOW, COLOR_BLACK);
init_pair(4, COLOR_BLUE, COLOR_BLACK);
init_pair(5, COLOR_MAGENTA, COLOR_BLACK);
init_pair(6, COLOR_CYAN, COLOR_BLACK);
init_pair(7, COLOR_WHITE, COLOR_BLACK);
noecho();
curs_set(0);
keypad(stdscr, TRUE);
timeout(speed);
srand(time(NULL));
while (true) {
if (can_move(1, 0, 0)) {
move(1, 0, 0);
} else {
fix();
int cnt = 0;
for (int i = 0; i < 20; i++) {
if (can_clear(i)) {
clear(i);
cnt++;
}
}
if (cnt) {
timeout(speed - cnt * 10);
}
if (game_over()) {
break;
}
x = 0;
y = 4;
shape = rand() % 7;
color = rand() % 7 + 1;
draw();
}
int ch = getch();
switch (ch) {
case KEY_LEFT:
if (can_move(0, -2, 0)) {
move(0, -2, 0);
}
break;
case KEY_RIGHT:
if (can_move(0, 2, 0)) {
move(0, 2, 0);
}
break;
case KEY_UP:
if (can_move(0, 0, 1)) {
move(0, 0, 1);
}
break;
case KEY_DOWN:
if (can_move(1, 0, 0)) {
move(1, 0, 0);
}
break;
case 'q':
case 'Q':
endwin();
return;
}
}
endwin();
cout << "Game over! Your score is " << score << "." << endl;
}
static const int shapes[7];
};
const int Tetris::shapes[7] = {
0x0F00, 0x2222, 0x00F0, 0x4444,
0x04E0, 0x0E40, 0x4640
};
int main() {
Tetris tetris;
tetris.play();
return 0;
}
```
阅读全文
相关推荐
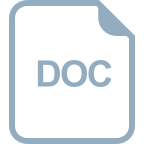
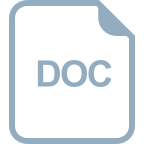
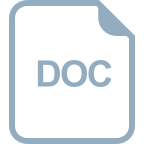
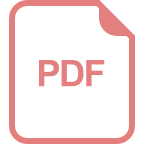
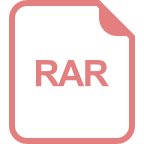


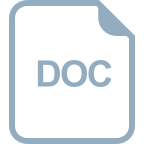
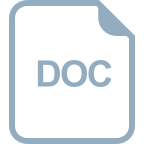
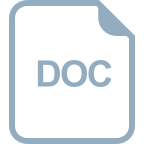


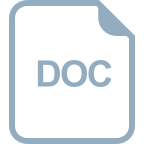
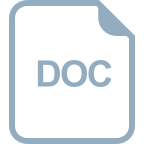
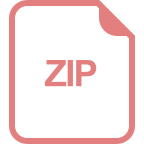