能否提供一个用do-while语句实现计算任意正整数n阶乘的C/C++代码示例?
时间: 2024-12-16 16:19:42 浏览: 9
当然可以,虽然在C/C++中标准的编程语言规范并不推荐过多使用do-while语句来处理迭代计数,因为for循环更为直观,但在某些特定场景下,do-while确实能解决问题。这里有一个使用do-while的简单C++代码示例,用于计算任意正整数n的阶乘:
```cpp
#include <iostream>
int factorial(int n) {
int fact = 1;
do {
fact *= n;
n--;
} while(n > 0);
return fact;
}
int main() {
int n;
std::cout << "请输入一个正整数: ";
std::cin >> n;
if (n >= 0) {
int result = factorial(n);
std::cout << "该数字的阶乘是: " << result << std::endl;
} else {
std::cout << "输入错误,只能计算正整数的阶乘." << std::endl;
}
return 0;
}
```
在这个代码里,do-while循环会一直执行,直到`n`变为0为止。注意,由于do-while至少执行一次,所以需要在循环体开始前初始化变量`fact`为1。
相关问题
c++根据用户输入的n值, 用for 语句、do...while语句、while语句实现求n的阶乘。
在C++中,你可以使用三种循环结构(for、do-while 和 while)来计算给定数n的阶乘。这里分别给出示例:
1. **使用for循环**:
```cpp
#include <iostream>
int factorial(int n) {
int result = 1;
for (int i = 1; i <= n; ++i) {
result *= i;
}
return result;
}
int main() {
int n;
std::cout << "请输入一个正整数: ";
std::cin >> n;
int fact = factorial(n);
std::cout << "n的阶乘是: " << fact << std::endl;
return 0;
}
```
2. **使用do...while循环**:
```cpp
#include <iostream>
int factorial(int n) {
int result = 1;
do {
result *= ++i;
} while (i <= n);
return result;
}
int main() {
int n, i = 1;
std::cout << "请输入一个正整数: ";
std::cin >> n;
int fact = factorial(n);
std::cout << "n的阶乘是: " << fact << std::endl;
return 0;
}
```
注意,在do...while循环里,`i`变量在循环体内部被自增,所以需要先初始化。
3. **使用while循环**:
```cpp
#include <iostream>
int factorial(int n) {
int result = 1, i = 1;
while (i <= n) {
result *= i;
++i;
}
return result;
}
int main() {
int n;
std::cout << "请输入一个正整数: ";
std::cin >> n;
int fact = factorial(n);
std::cout << "n的阶乘是: " << fact << std::endl;
return 0;
}
```
编程语言(1)编程:用 while 语句编写,从键盘输入一个正整数 n,求 n!。 (2)编程:用 do-while 语句编写,从1加到 100,求和。
当然可以。
(1) 使用 `while` 语句编写计算阶乘的程序如下:
```python
n = int(input("请输入一个正整数 n: "))
factorial = 1
while n > 0:
factorial *= n
n -= 1
print(f"{n} 的阶乘是 {factorial}")
```
在这个例子中,我们首先获取用户输入的正整数 `n`,然后初始化阶乘为1。接着,在 `while` 循环中,每次迭代都将 `factorial` 乘以 `n`,然后将 `n` 减1,直到 `n` 变为0,循环结束。
(2) 使用 `do-while` 语句编写累加1到100的和的程序类似于这样:
```c++
#include <iostream>
int main() {
int sum = 0;
int num = 1;
do {
sum += num;
num++;
} while (num <= 100);
std::cout << "1 到 100 的和是 " << sum << std::endl;
return 0;
}
```
在这个do-while循环中,先执行一次累加操作(将`sum`加上`num`),然后判断`num`是否小于等于100,如果满足条件则继续循环,否则退出循环并输出结果。
阅读全文
相关推荐
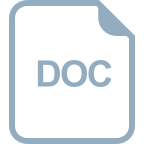
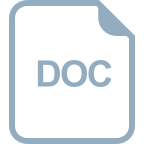
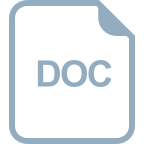







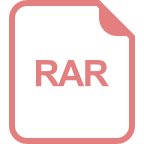
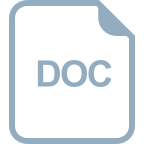

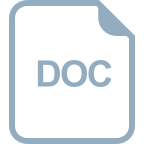
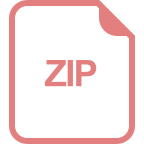
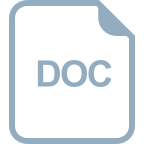
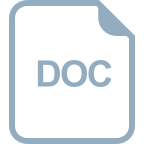
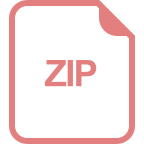