plt.plot(x,y) ax=plt.gca() ax.set_yscale('log')
时间: 2024-04-22 19:27:52 浏览: 253
你的代码是正确的!首先,使用 `plt.plot(x, y)` 绘制了一条曲线。接下来,使用 `plt.gca()` 获取当前轴对象,并将其赋值给变量 `ax`。最后,使用 `ax.set_yscale('log')` 将纵向比例尺设置为对数比例尺。
这段代码的作用是在对数比例尺下绘制一条曲线。对数比例尺可用于显示数据范围很大的情况,可以更好地展示数据中的细节。
请确保在运行这段代码之前已经导入了 `matplotlib.pyplot` 模块,并且已经定义了变量 `x` 和 `y` 来存储曲线的横纵坐标数据。
如果需要显示图形,还需要调用 `plt.show()` 方法来显示绘制的图形。完整的代码示例如下:
```python
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [1, 10, 100, 1000]
plt.plot(x, y)
ax = plt.gca()
ax.set_yscale('log')
plt.show()
```
运行这段代码后,将会得到一条在对数比例尺下绘制的曲线。
相关问题
import numpy as np from matplotlib_inline import backend_inline from d2l import torch as d2l def f(x): return 3*x**2-4*x def numerical_lim(f,x,h): return(f(x+h)-f(x))/h def use_svg_display(): #@save backend_inline.set_matplotlib_formats('svg') def set_figsize(figsize=(3.5,2.5)): #@save use_svg_display() d2l.plt.rcParams['figure.figsize'] = figsize #@save def set_axes(axes,xlabel,ylabel,xlim,ylim,xscale,yscale,legend): axes.set_xlabel(xlabel) axes.set_ylabel(ylabel) axes.set_xscale(xscale) axes.set_yscale(yscale) axes.set_xlim(xlim) axes.set_ylim(ylim) if legend: axes.legend(legend) axes.grid() #@save def plot(X,Y=None,xlabel=None,ylabel=None,legend=None,xlim=None,ylim=None,xscale='linear',yscale='linear', fmts=('-','m--','g-.','r:'),figsize=(3.5,2.5),axes=None): if legend is None: legend = [] set_figsize(figsize) axes = axes if axes else d2l.plt.gca() def has_one_axis(X): return (hasattr(X,"ndim")and X.ndim == 1 or isinstance(X,list) and not hasattr(X[0],"__len__")) if has_one_axis(X): X = [X] if Y is None: X,Y = [[]]*len(X),X if has_one_axis(Y): Y = [Y] if len(X) != len(Y): X = X*len(Y) axes.cla() for x,y,fmt in zip(X,Y,fmts): if len(x): axes.plot(x,y,fmt) else: axes.plot(y,fmt) set_axes(axes,xlabel,ylabel,xlim ,ylim,xscale,yscale,legend) x = np.arange(0,3,0.1) plot(x,[f(x),2*x-3],'x','f(x)',legend=['f(x)','Tangent line(x=1)'])这段代码为什么在pycharm中运行不出来图形
这段代码在 PyCharm 中无法显示图形的原因可能是因为缺少了 `plt.show()` 的调用。在 PyCharm 中,需要手动调用 `plt.show()` 来显示图形窗口。你可以在代码的最后添加 `plt.show()` 来尝试解决该问题。修改后的代码如下所示:
```python
import numpy as np
from matplotlib_inline import backend_inline
from d2l import torch as d2l
def f(x):
return 3*x**2-4*x
def numerical_lim(f,x,h):
return(f(x+h)-f(x))/h
def use_svg_display(): #@save
backend_inline.set_matplotlib_formats('svg')
def set_figsize(figsize=(3.5,2.5)): #@save
use_svg_display()
d2l.plt.rcParams['figure.figsize'] = figsize
#@save
def set_axes(axes,xlabel,ylabel,xlim,ylim,xscale,yscale,legend):
axes.set_xlabel(xlabel)
axes.set_ylabel(ylabel)
axes.set_xscale(xscale)
axes.set_yscale(yscale)
axes.set_xlim(xlim)
axes.set_ylim(ylim)
if legend:
axes.legend(legend)
axes.grid()
#@save
def plot(X,Y=None,xlabel=None,ylabel=None,legend=None,xlim=None,ylim=None,xscale='linear',yscale='linear',
fmts=('-','m--','g-.','r:'),figsize=(3.5,2.5),axes=None):
if legend is None:
legend = []
set_figsize(figsize)
axes = axes if axes else d2l.plt.gca()
def has_one_axis(X):
return (hasattr(X,"ndim")and X.ndim == 1 or isinstance(X,list)
and not hasattr(X[0],"__len__"))
if has_one_axis(X):
X = [X]
if Y is None:
X,Y = [[]]*len(X),X
if has_one_axis(Y):
Y = [Y]
if len(X) != len(Y):
X = X*len(Y)
axes.cla()
for x,y,fmt in zip(X,Y,fmts):
if len(x):
axes.plot(x,y,fmt)
else:
axes.plot(y,fmt)
set_axes(axes,xlabel,ylabel,xlim
,ylim,xscale,yscale,legend)
x = np.arange(0,3,0.1)
plot(x,[f(x),2*x-3],'x','f(x)',legend=['f(x)','Tangent line(x=1)'])
d2l.plt.show()
```
请注意,`import matplotlib.pyplot as plt` 语句和 `plt.show()` 的调用被添加到了代码中。运行修改后的代码,你应该能够在 PyCharm 中看到图形窗口显示出来。
#@save def plot(X, Y=None, xlabel=None, ylabel=None, legend=None, xlim=None, ylim=None, xscale='linear', yscale='linear', fmts=('-', 'm--', 'g-.', 'r:'), figsize=(3.5, 2.5), axes=None): """绘制数据点""" if legend is None: legend = [] set_figsize(figsize) axes = axes if axes else d2l.plt.gca() # 如果X有一个轴,输出True def has_one_axis(X): return (hasattr(X, "ndim") and X.ndim == 1 or isinstance(X, list) and not hasattr(X[0], "__len__")) if has_one_axis(X): X = [X] if Y is None: X, Y = [[]] * len(X), X elif has_one_axis(Y): Y = [Y] if len(X) != len(Y): X = X * len(Y) axes.cla() for x, y, fmt in zip(X, Y, fmts): if len(x): axes.plot(x, y, fmt) else: axes.plot(y, fmt) set_axes(axes, xlabel, ylabel, xlim, ylim, xscale, yscale, legend)
这段代码定义了一个名为`plot()`的函数,用于绘制数据点的图像。
函数参数解释:
- `X`: x轴上的数据点,可以是一个列表或多个列表的列表。
- `Y`: y轴上的数据点,可以是一个列表或多个列表的列表。
- `xlabel`: x轴的标签。
- `ylabel`: y轴的标签。
- `legend`: 图例标签,是一个列表。
- `xlim`: x轴的取值范围,是一个包含两个元素的列表或元组。
- `ylim`: y轴的取值范围,是一个包含两个元素的列表或元组。
- `xscale`: x轴的缩放类型,可以是'linear'(线性缩放)或'log'(对数缩放)。
- `yscale`: y轴的缩放类型,可以是'linear'(线性缩放)或'log'(对数缩放)。
- `fmts`: 绘制线条的格式,是一个包含线条样式的字符串列表。
- `figsize`: 绘图的尺寸,是一个包含宽度和高度的元组。
- `axes`: matplotlib的轴对象,用于绘制图像。
函数主要步骤:
1. 如果`legend`为空,则将其设置为空列表。
2. 调用`set_figsize(figsize)`函数设置绘图的尺寸。
3. 如果`axes`存在,则使用`axes`作为绘图的轴对象,否则使用`d2l.plt.gca()`获取当前的轴对象。
4. 定义了一个名为`has_one_axis(X)`的内部函数,用于判断`X`是否只有一个轴。
5. 如果`X`只有一个轴,则将其包装为列表。
6. 如果`Y`为空,则将`X`设置为空列表,将`Y`设置为`X`。
7. 如果`Y`只有一个轴,则将其包装为列表。
8. 如果`X`和`Y`的长度不一致,则将`X`复制到与`Y`相同的长度。
9. 使用`axes.cla()`清除轴对象上的所有绘图。
10. 使用`zip(X, Y, fmts)`将`X`、`Y`和`fmts`打包成元组序列。
11. 遍历打包后的元组序列,对每个元组进行如下操作:
- 如果`x`的长度大于0,则使用`axes.plot(x, y, fmt)`绘制数据点的图像。
- 否则,使用`axes.plot(y, fmt)`绘制数据点的图像。
12. 调用`set_axes()`函数设置轴对象的属性,包括标签、取值范围、缩放类型和图例。
通过调用这个函数,可以方便地绘制数据点的图像,并设置轴的属性,包括标签、取值范围、缩放类型和图例。
阅读全文
相关推荐
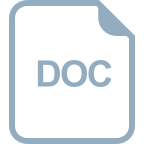
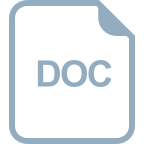
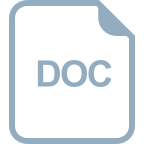



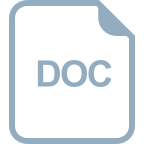
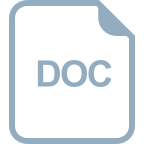
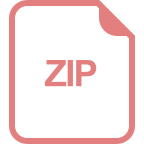
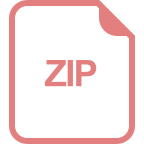