Java道格拉斯普克抽稀算法
时间: 2023-11-30 18:40:38 浏览: 126
以下是Java实现的道格拉斯-普克抽稀算法的示例代码:
```java
import java.util.ArrayList;
import java.util.List;
public class DouglasPeucker {
public static List<Point> douglasPeucker(List<Point> points, double epsilon) {
if (points == null || points.size() < 3) {
return points;
}
int maxIndex = 0;
double maxDistance = 0;
int endIndex = points.size() - 1;
for (int i = 1; i < endIndex; i++) {
double distance = perpendicularDistance(points.get(i), points.get(0), points.get(endIndex));
if (distance > maxDistance) {
maxIndex = i;
maxDistance = distance;
}
}
List<Point> resultList = new ArrayList<>();
if (maxDistance > epsilon) {
List<Point> leftList = points.subList(0, maxIndex + 1);
List<Point> rightList = points.subList(maxIndex, endIndex + 1);
List<Point> leftResultList = douglasPeucker(leftList, epsilon);
List<Point> rightResultList = douglasPeucker(rightList, epsilon);
resultList.addAll(leftResultList.subList(0, leftResultList.size() - 1));
resultList.addAll(rightResultList);
} else {
resultList.add(points.get(0));
resultList.add(points.get(endIndex));
}
return resultList;
}
private static double perpendicularDistance(Point point, Point start, Point end) {
double area = Math.abs(0.5 * (start.getX() * end.getY() + end.getX() * point.getY() + point.getX() * start.getY() - end.getX() * start.getY() - point.getX() * end.getY() - start.getX() * point.getY()));
double bottom = Math.sqrt(Math.pow(start.getX() - end.getX(),2) + Math.pow(start.getY() - end.getY(), 2));
double height = area / bottom * 2;
return height;
}
public static class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
}
}
```
上述代码中,`douglasPeucker`方法接收一个点集和一个误差值`epsilon`,返回一个抽稀后的点集。`perpendicularDistance`方法用于计算一个点到一条线段的垂直距离。`Point`类表示一个二维坐标点。
阅读全文
相关推荐

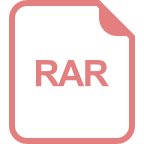
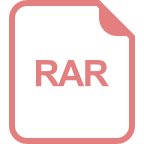
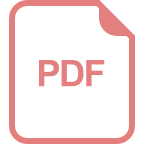
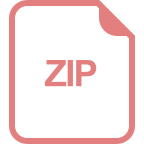
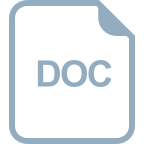


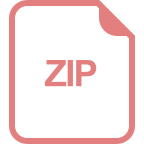
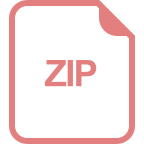
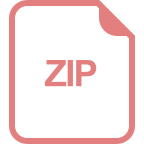
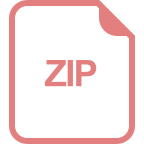
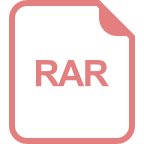