java道格拉斯普克算法 工具包
时间: 2023-12-22 18:28:58 浏览: 152
根据您提供的引用内容,道格拉斯-普克算法是一种将曲线近似表示为一系列点,并减少点的数量的算法。如果您想在Java中使用道格拉斯-普克算法,可以考虑使用以下工具包:
1. JTS Topology Suite:JTS是一个Java库,提供了一套用于处理地理空间数据的工具。它包含了道格拉斯-普克算法的实现,可以用于抽稀经纬度路径点。您可以通过以下代码示例来使用JTS Topology Suite进行抽稀:
```java
import org.locationtech.jts.geom.Coordinate;
import org.locationtech.jts.geom.GeometryFactory;
import org.locationtech.jts.simplify.DouglasPeuckerSimplifier;
public class DouglasPeuckerExample {
public static void main(String[] args) {
// 输入的经纬度路径点
Coordinate[] inputPoints = new Coordinate[] {
new Coordinate(0, 0),
new Coordinate(1, 1),
new Coordinate(2, 2),
new Coordinate(3, 3),
new Coordinate(4, 4)
};
// 创建GeometryFactory对象
GeometryFactory geometryFactory = new GeometryFactory();
// 创建LineString对象
org.locationtech.jts.geom.LineString lineString = geometryFactory.createLineString(inputPoints);
// 使用Douglas-Peucker算法进行抽稀
double tolerance = 0.5; // 抽稀的容差值
DouglasPeuckerSimplifier simplifier = new DouglasPeuckerSimplifier(lineString);
simplifier.setDistanceTolerance(tolerance);
org.locationtech.jts.geom.LineString simplifiedLineString = (org.locationtech.jts.geom.LineString) simplifier.getResultGeometry();
// 输出抽稀后的经纬度路径点
Coordinate[] simplifiedPoints = simplifiedLineString.getCoordinates();
for (Coordinate point : simplifiedPoints) {
System.out.println("Latitude: " + point.x + ", Longitude: " + point.y);
}
}
}
```
2. GeoTools:GeoTools是一个开源的Java库,用于处理地理空间数据。它提供了许多空间分析和地理信息系统功能,包括道格拉斯-普克算法的实现。您可以使用以下代码示例来使用GeoTools进行抽稀:
```java
import org.geotools.geometry.jts.JTSFactoryFinder;
import org.geotools.simplify.DouglasPeuckerSimplifier;
import org.locationtech.jts.geom.Coordinate;
import org.locationtech.jts.geom.Geometry;
import org.locationtech.jts.geom.GeometryFactory;
public class DouglasPeuckerExample {
public static void main(String[] args) {
// 输入的经纬度路径点
Coordinate[] inputPoints = new Coordinate[] {
new Coordinate(0, 0),
new Coordinate(1, 1),
new Coordinate(2, 2),
new Coordinate(3, 3),
new Coordinate(4, 4)
};
// 创建GeometryFactory对象
GeometryFactory geometryFactory = JTSFactoryFinder.getGeometryFactory();
// 创建LineString对象
Geometry lineString = geometryFactory.createLineString(inputPoints);
// 使用Douglas-Peucker算法进行抽稀
double tolerance = 0.5; // 抽稀的容差值
DouglasPeuckerSimplifier simplifier = new DouglasPeuckerSimplifier(lineString);
simplifier.setDistanceTolerance(tolerance);
Geometry simplifiedGeometry = simplifier.getResultGeometry();
// 输出抽稀后的经纬度路径点
Coordinate[] simplifiedPoints = simplifiedGeometry.getCoordinates();
for (Coordinate point : simplifiedPoints) {
System.out.println("Latitude: " + point.x + ", Longitude: " + point.y);
}
}
}
```
请注意,以上示例代码仅为演示如何使用Java工具包实现道格拉斯-普克算法进行抽稀。您可以根据自己的需求进行调整和扩展。
阅读全文
相关推荐
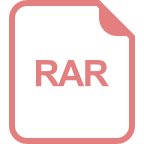
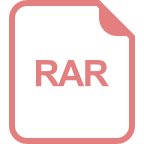
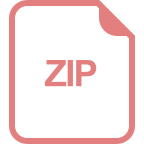
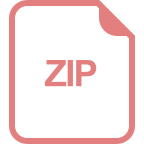
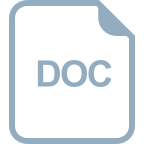
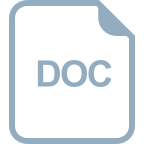
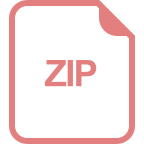
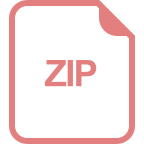
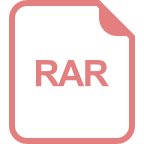
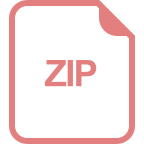
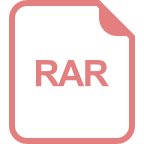
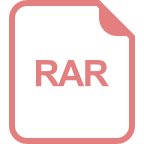
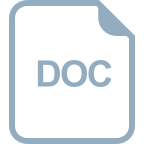
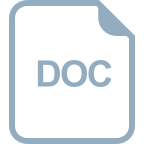
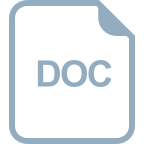