可以不写std::string::吗,直接写find什么的
时间: 2024-01-11 14:02:05 浏览: 70
是的,可以通过使用命名空间来避免在代码中反复写`std::string::`。下面是一个示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello, World!";
string substr = "World";
size_t found = str.find(substr);
if (found != string::npos) {
cout << "Substring found at position: " << found << endl;
} else {
cout << "Substring not found." << endl;
}
return 0;
}
```
在上面的示例中,通过使用`using namespace std;`语句,我们可以直接使用`string`和`npos`,而不必写`std::string::`。这使得代码更加简洁和易读。
但是需要注意的是,使用命名空间时要小心避免发生名称冲突。如果在代码中有多个命名空间,并且这些命名空间中有相同名称的函数或变量,那么可能会导致编译错误或意外的行为。因此,在较大的项目中,最好明确指定命名空间或使用作用域限定符来避免潜在的问题。
相关问题
std::string find 大小写
在C++中,可以使用`std::transform`函数将字符串转换为小写或大写。下面是一个示例代码:
```cpp
#include <iostream>
#include <algorithm>
#include <string>
int main() {
std::string str = "Hello World";
std::transform(str.begin(), str.end(), str.begin(), ::tolower);
std::cout << str << std::endl; // 输出:hello world
std::transform(str.begin(), str.end(), str.begin(), ::toupper);
std::cout << str << std::endl; // 输出:HELLO WORLD
return 0;
}
```
在上面的代码中,我们使用`std::transform`函数将字符串`str`转换为小写字母,并将结果存储回原字符串。然后,我们再次使用`std::transform`函数将字符串转换为大写字母,并将结果存储回原字符串。最后,我们输出转换后的字符串。
std::string中find依赖什么库
`std::string` 是 C++ 标准库中的一个容器类,用于处理字符串。`std::string` 类提供了很多操作字符串的方法,其中 `find` 方法用于在字符串中查找子字符串或字符的位置。`std::string` 及其 `find` 方法是定义在 C++ 的标准模板库(Standard Template Library,简称 STL)中的。
为了使用 `std::string` 及其 `find` 方法,你需要包含 C++ 标准库中的 `<string>` 头文件。当你的程序包含了这个头文件之后,你就可以使用 `std::string` 类型和它的成员函数了。
示例代码:
```cpp
#include <string>
int main() {
std::string str = "Hello, World!";
size_t pos = str.find("World");
if (pos != std::string::npos) {
// 找到了子字符串 "World"
}
return 0;
}
```
在这个例子中,`std::string::find` 方法用于查找子字符串 "World" 在字符串 `str` 中的位置。
阅读全文
相关推荐
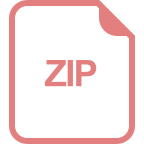
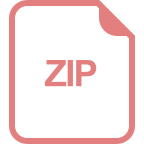
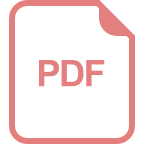













