编写程序实现任意斜率的Bresenham 直线生成算法,要求:基于鼠标实现橡皮筋 动态绘制直线效果,即在屏幕上单击鼠标左键开始绘制(直线的第一个端点),按住鼠标 左键的同时拖动鼠标,跟踪鼠标位置(直线的第二个端点),动态绘制直线。直到释放鼠 标左键,绘制最终直线。
时间: 2024-10-27 17:13:46 浏览: 21
为了实现这个功能,我们需要结合一些基本图形库,如Python的Pygame库,用于处理窗口和用户输入,以及Bresenham算法来计算屏幕上的像素点。以下是使用Python和Pygame的一个简单步骤:
1. 首先安装Pygame库,如果尚未安装,可以使用pip安装:
```
pip install pygame
```
2. 导入必要的模块:
```python
import pygame
from math import sqrt
```
3. 定义函数来检查鼠标是否按下并移动:
```python
def bresenham_line(screen, start_pos, end_pos):
# Bresenham算法
dx = abs(end_pos[0] - start_pos[0])
dy = abs(end_pos[1] - start_pos[1])
is_steep = dy > dx
if is_steep:
x_start, y_start = start_pos
x_end, y_end = end_pos
swap = lambda x, y: (y, x) if is_steep else (x, y)
start_pos, end_pos = swap(start_pos)
dx, dy = swap(dx, dy)
x_axis_step = 1 if start_pos[0] < end_pos[0] else -1
y_axis_step = 1 if start_pos[1] < end_pos[1] else -1
for i in range(dx + 1):
if is_steep:
screen.set_at((start_pos[0], y_start + i * y_axis_step), (0, 0, 0)) # 空白画布
else:
screen.set_at((x_start + i * x_axis_step, start_pos[1]), (0, 0, 0))
for i in range(dx):
if is_steep:
y = start_pos[1] + i * y_axis_step
x = int(start_pos[0] + ((i + 0.5) / dx) * (end_pos[0] - start_pos[0]))
screen.set_at((x, y), (255, 255, 255)) # 绘制白色像素
else:
x = start_pos[0] + i * x_axis_step
y = int(start_pos[1] + ((i + 0.5) / dy) * (end_pos[1] - start_pos[1]))
screen.set_at((x, y), (255, 255, 255))
# 主游戏循环
def main():
# 初始化Pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN and event.button == 1:
click_pos = pygame.mouse.get_pos()
dragging = True
elif event.type == pygame.MOUSEBUTTONUP and event.button == 1:
dragging = False
line_end_pos = pygame.mouse.get_pos()
bresenham_line(screen, click_pos, line_end_pos)
pygame.display.update()
if dragging:
line_end_pos = pygame.mouse.get_pos()
bresenham_line(screen, click_pos, line_end_pos)
pygame.quit()
```
4. 运行`main()`函数开始游戏。
阅读全文
相关推荐
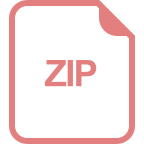
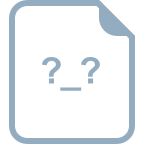


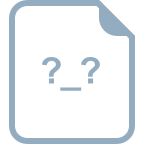
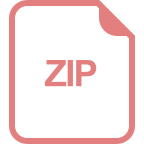
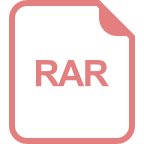
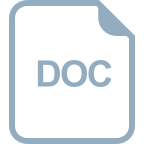
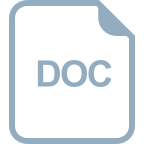
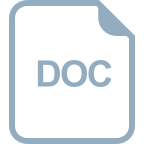
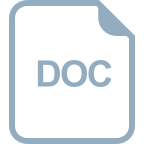
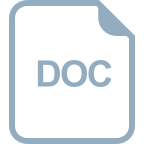
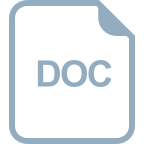
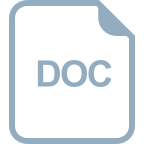




