sklearn的多项式回归
时间: 2023-09-04 09:06:26 浏览: 111
多项式回归是一种线性回归的扩展,它通过引入多项式特征来拟合非线性数据。在sklearn库中,可以使用PolynomialFeatures类来实现多项式特征的生成,然后再将生成的特征传递给线性回归模型进行拟合。
下面是一个使用sklearn进行多项式回归的示例代码:
```python
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
import numpy as np
# 生成一些非线性数据
X = np.array([1, 2, 3, 4, 5]).reshape(-1, 1)
y = np.array([2, 3, 6, 9, 15])
# 创建多项式特征生成器,指定最高次数为2
poly = PolynomialFeatures(degree=2)
X_poly = poly.fit_transform(X)
# 创建线性回归模型并拟合数据
regressor = LinearRegression()
regressor.fit(X_poly, y)
# 预测新的数据样本
new_X = np.array([6]).reshape(-1, 1)
new_X_poly = poly.transform(new_X)
predicted_y = regressor.predict(new_X_poly)
print(predicted_y)
```
相关问题
sklearn 多项式回归
多项式回归是一种在线性回归的基础上引入多项式特征的方法。在sklearn中,可以使用PolynomialFeatures类来实现多项式特征的创建,然后使用线性回归模型进行拟合。
下面是一个使用sklearn进行多项式回归的示例代码:
```python
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
from sklearn.pipeline import make_pipeline
import numpy as np
# 创建一些带有噪声的训练数据
np.random.seed(0)
X = 2 * np.pi * np.random.rand(100, 1)
y = np.sin(X) + 0.2 * np.random.randn(100, 1)
# 创建多项式特征
degree = 3 # 设置多项式的阶数
poly_features = PolynomialFeatures(degree=degree, include_bias=False)
X_poly = poly_features.fit_transform(X)
# 使用线性回归模型进行拟合
model = make_pipeline(PolynomialFeatures(degree), LinearRegression())
model.fit(X, y)
# 预测新的数据
X_test = np.linspace(0, 2 * np.pi, 100).reshape(-1, 1)
y_pred = model.predict(X_test)
```
在上面的代码中,首先使用`PolynomialFeatures`类将原始输入特征`X`转换为包含多项式特征的`X_poly`。然后使用`make_pipeline`函数创建一个包含多项式特征转换和线性回归模型的管道。最后,使用管道进行拟合和预测。
希望对你有帮助!如果还有其他问题,请继续提问。
sklearn多项式回归
多项式回归是一种基于线性回归的扩展方法,它通过将输入变量的多项式作为特征来建立非线性关系模型。在sklearn库中,多项式回归可以通过PolynomialFeatures类和LinearRegression类来实现。
首先,你需要导入所需的库:
```python
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
```
接下来,你可以使用PolynomialFeatures类来转换输入特征为多项式形式:
```python
# 创建PolynomialFeatures对象,指定多项式的阶数
poly_features = PolynomialFeatures(degree=n)
# 将输入特征X转换为多项式形式
X_poly = poly_features.fit_transform(X)
```
其中,n是多项式的阶数。
然后,你可以使用LinearRegression类来拟合多项式回归模型:
```python
# 创建LinearRegression对象
model = LinearRegression()
# 拟合多项式回归模型
model.fit(X_poly, y)
```
其中,X_poly是转换后的多项式特征矩阵,y是目标变量。
最后,你可以使用拟合好的模型进行预测:
```python
# 预测新样本
y_pred = model.predict(poly_features.transform(new_X))
```
这样,你就可以使用sklearn库进行多项式回归建模和预测了。希望对你有所帮助!如果还有其他问题,请继续提问。
阅读全文
相关推荐






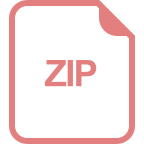




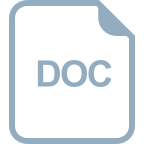
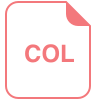

