C++设计一个基于图的邻接表的图的基本操作的演示程序。 【基本要求】 实现下列六种基本运算:(1)创建图;(2)深度优先遍历图;(3)广度优先遍历图;(4)转换图的存储结构 分别在图的邻接矩阵和邻接表的存储结构实现
时间: 2024-12-13 16:24:43 浏览: 35
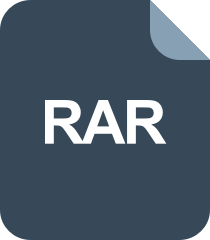
AdjGraph.rar_数据结构_Visual_C++_
在C++中,我们可以使用邻接表来表示图,因为邻接表对于稀疏图(边的数量远小于顶点数量的平方)更节省空间。首先,我们定义一个邻接表节点`Node`和一个`AdjacencyList`类。以下是针对基本操作的一个简单示例:
```cpp
#include <iostream>
#include <list>
// 邻接列表节点
struct Node {
int vertex; // 顶点ID
std::list<int> neighbors; // 存储邻居顶点的列表
};
// 图的邻接列表表示
class AdjacencyListGraph {
private:
std::vector<Node*> nodes; // 存储所有节点
public:
// 创建图
void createGraph(int numVertices) {
for (int i = 0; i < numVertices; ++i) {
nodes.push_back(new Node{i});
}
}
// 添加边
void addEdge(int src, int dest) {
nodes[src]->neighbors.push_back(dest);
}
// 深度优先搜索(DFS)
void dfs(int start) {
bool visited[numVertices] = {false};
dfsHelper(start, visited);
printPath(start);
}
// 深度优先搜索辅助函数
void dfsHelper(int vertex, bool visited[]) {
visited[vertex] = true;
std::cout << vertex << " ";
for (auto& neighbor : nodes[vertex].neighbors) {
if (!visited[neighbor]) {
dfsHelper(neighbor, visited);
}
}
}
// 广度优先搜索(BFS)
void bfs(int start) {
bool visited[numVertices] = {false};
std::queue<int> queue;
visited[start] = true;
queue.push(start);
while (!queue.empty()) {
int current = queue.front();
queue.pop();
std::cout << current << " ";
for (auto& neighbor : nodes[current].neighbors) {
if (!visited[neighbor]) {
visited[neighbor] = true;
queue.push(neighbor);
}
}
}
}
// 转换存储结构(假设从邻接矩阵转到邻接表)
void convertToAdjacencyList() {
for (auto& node : nodes) {
for (int i = 0; i < numVertices; ++i) {
if (node->neighbors.find(i) != node->neighbors.end()) {
addEdge(node->vertex, i);
}
}
}
}
// 打印路径(仅用于DFS示例)
void printPath(int start) {
std::cout << "DFS path from " << start << ": ";
}
~AdjacencyListGraph() {
for (auto* node : nodes) {
delete node;
}
}
};
int main() {
const int numVertices = 5;
AdjacencyListGraph graph;
graph.createGraph(numVertices); // 示例:graph.addEdge(0, 1); graph.addEdge(1, 2); ...;
graph.bfs(0); // 测试BFS
graph.dfs(0); // 测试DFS
return 0;
}
```
这个例子展示了如何创建、添加边、以及使用深度优先和广度优先遍历图。`convertToAdjacencyList`方法模拟了从邻接矩阵转换到邻接表的过程。
阅读全文