C++设计一个基于图的邻接矩阵的图的基本操作的演示程序。 【基本要求】 实现下列六种基本运算:(1)创建图;(2)深度优先遍历图;(3)广度优先遍历图;(4)转换图的存储结构 分别在图的邻接矩阵和邻接表的存储结构实现
时间: 2024-12-14 14:19:42 浏览: 11
在C++中,我们可以先定义一个邻接矩阵表示图的数据结构,然后实现相应的基本操作。这里是一个简化的示例:
```cpp
#include <iostream>
#include <vector>
// 图的邻接矩阵数据结构
class AdjacencyMatrixGraph {
public:
int V; // 节点数
std::vector<std::vector<int>> adjMatrix;
// 创建图
AdjacencyMatrixGraph(int vertices) : V(vertices) {
adjMatrix.resize(V, std::vector<int>(V, 0));
}
// 添加边 (u, v)
void addEdge(int u, int v) {
adjMatrix[u][v] = 1;
adjMatrix[v][u] = 1; // 如果是无向图,则添加双向边
}
// 深度优先搜索
void dfs(int start) {
std::vector<bool> visited(V, false);
dfsHelper(start, visited);
}
private:
void dfsHelper(int node, std::vector<bool>& visited) {
visited[node] = true;
std::cout << node << " ";
for (int i = 0; i < V; ++i) {
if (adjMatrix[node][i] && !visited[i])
dfsHelper(i, visited);
}
}
// 广度优先搜索
void bfs(int start) {
std::vector<bool> visited(V, false);
std::queue<int> q;
visited[start] = true;
q.push(start);
while (!q.empty()) {
int node = q.front();
q.pop();
std::cout << node << " ";
for (int i = 0; i < V; ++i) {
if (adjMatrix[node][i] && !visited[i]) {
visited[i] = true;
q.push(i);
}
}
}
}
// 转换为邻接表表示
void convertToAdjList() {
std::vector<std::vector<int>> adjList(V);
for (int i = 0; i < V; ++i) {
for (int j = 0; j < V; ++j) {
if (adjMatrix[i][j]) {
adjList[i].push_back(j);
}
}
}
std::cout << "Adjacency list:\n";
for (auto& neighbors : adjList) {
for (int neighbor : neighbors)
std::cout << neighbor << " ";
std::cout << "\n";
}
}
};
int main() {
int numVertices;
std::cout << "Enter number of vertices: ";
std::cin >> numVertices;
AdjacencyMatrixGraph graph(numVertices);
int edges;
std::cout << "Enter number of edges and their endpoints: \n";
for (int i = 0; i < edges; ++i) {
int u, v;
std::cin >> u >> v;
graph.addEdge(u, v);
}
std::cout << "Depth First Search starting from vertex 0: ";
graph.dfs(0);
std::cout << "\n";
std::cout << "Breadth First Search starting from vertex 0: ";
graph.bfs(0);
std::cout << "\n";
graph.convertToAdjList();
return 0;
}
```
这个程序首先创建了一个邻接矩阵图,并允许用户输入节点数和边的连接。接着,它展示了深度优先搜索(DFS)和广度优先搜索(BFS),以及如何将邻接矩阵转换为邻接表。请注意,这只是一个简化版本,实际项目可能需要更详细的错误处理和用户交互。运行此程序时,按照提示输入节点数和边的信息即可。
阅读全文
相关推荐
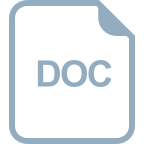
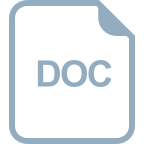
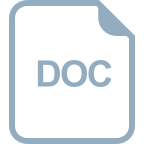



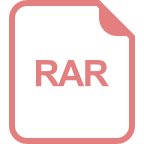



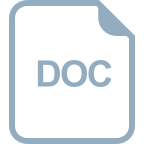
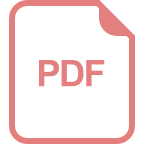
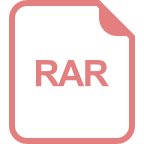
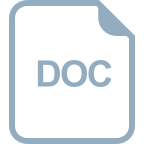
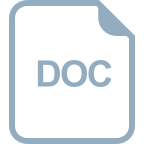
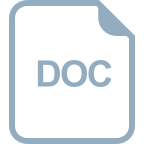
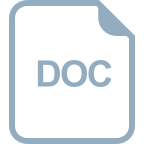
