请用C++简单实现哈希表,冲突采用链地址法
时间: 2024-10-11 20:12:05 浏览: 15
在C++中,我们可以使用`std::unordered_map`,它底层实际上是基于开放寻址法(如Chaining)实现的哈希表。如果你想手动实现,可以创建一个简单的结构体,包含键值对以及一个链表来解决哈希冲突。下面是一个简化的例子:
```cpp
#include <iostream>
#include <list>
// 定义节点结构体
struct HashNode {
int key;
int value;
HashNode* next; // 链接指针
HashNode(int k, int v) : key(k), value(v), next(nullptr) {}
};
// 哈希表模板
template <typename K, typename V>
class SimpleHashTable {
private:
static const int TABLE_SIZE = 10;
HashNode** table;
public:
SimpleHashTable() {
table = new HashNode*[TABLE_SIZE];
for (int i = 0; i < TABLE_SIZE; ++i)
table[i] = nullptr;
}
~SimpleHashTable() {
for (HashNode** node = table; node != nullptr; node = node->next)
delete node;
delete[] table;
}
void insert(K key, V value) {
int hash = std::hash<K>{}(key) % TABLE_SIZE;
HashNode* newNode = new HashNode(key, value);
if (!table[hash])
table[hash] = newNode;
else {
HashNode* current = table[hash];
while (current->next != nullptr && current->key != key) {
current = current->next;
}
if (current->key == key)
return; // 如果已经存在,不做插入
newNode->next = current->next;
current->next = newNode;
}
}
V get(K key) {
int hash = std::hash<K>{}(key) % TABLE_SIZE;
HashNode* current = table[hash];
while (current != nullptr && current->key != key)
current = current->next;
if (current != nullptr)
return current->value;
else
return nullptr; // 表示找不到对应的键
}
};
int main() {
SimpleHashTable<int, int> hashTable;
hashTable.insert(5, 15);
hashTable.insert(7, 21);
int result = hashTable.get(5); // 获取5的值,结果应为15
std::cout << "Value of key 5: " << (result ? result : "Not found") << "\n";
return 0;
}
```
这个简单的哈希表实现了基本的插入和查找功能,并使用了链地址法处理冲突。
阅读全文
相关推荐
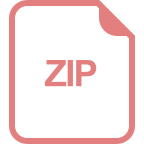
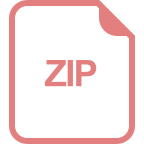
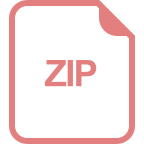














