C++数据结构带头链表实现
时间: 2024-09-18 09:17:03 浏览: 63
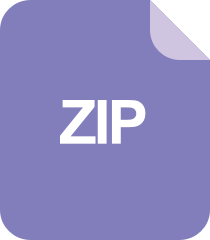
基于C++(QT)链表实现过程化(数据结构)【100011836】
在C++中,带头结点(也叫单链表头结点)是一种常见的数据结构,用于存储一系列动态大小的元素。这种结构通常包含两个部分:一个指向列表第一个元素的指针(称为头节点),以及每个普通节点的数据域和指向下一个节点的指针。
```cpp
// 定义链表节点结构体
struct Node {
int data; // 数据域
Node* next; // 指向下一个节点的指针
};
// 定义头结点
class LinkedList {
private:
Node* head; // 链表头节点
public:
LinkedList() { head = nullptr; } // 构造函数,初始化为空链表
// 插入新元素到链表头部
void push(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = head;
head = newNode;
}
// 删除指定值的第一个节点
void remove(int value) {
if (head && head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
}
else {
Node* current = head;
while (current && current->data != value) {
current = current->next;
}
if (current)
current->next = current->next->next;
}
}
// 遍历并打印链表
void printList() const {
Node* temp = head;
while (temp) {
std::cout << temp->data << " -> ";
temp = temp->next;
}
std::cout << "nullptr" << std::endl;
}
};
```
阅读全文
相关推荐
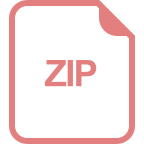
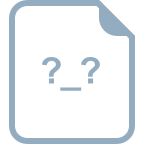
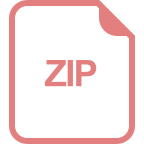
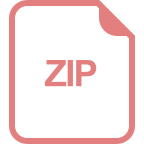
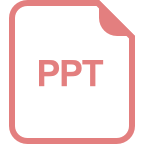
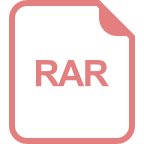
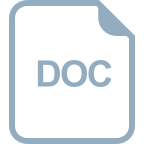
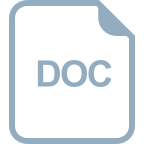
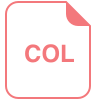

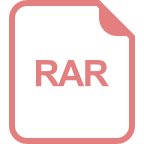
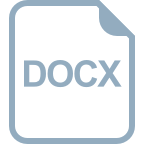
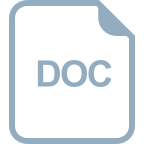



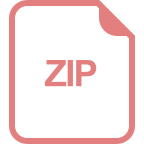