创建列表list1 = ['lift', 'is', 'short','You','need','python'],并完成以下任务:1)输出列表中元素‘short’及其索引下标;2)在‘short’后面增加一个‘!’。输出结果如下: 提示: (1)利用循环遍历列表中的元素。
时间: 2024-10-12 14:09:18 浏览: 30
首先,我们可以使用Python的`enumerate()`函数来同时获取列表元素和其对应的下标。下面是完成任务的步骤:
```python
list1 = ['lift', 'is', 'short', 'You', 'need', 'python']
# 1)输出元素'short'及其索引下标
for index, value in enumerate(list1):
if value == 'short':
print(f"元素'short'的索引是 {index}")
# 2)在'short'后面增加一个'!'
new_list = list1.copy() # 先复制原列表防止修改原列表
new_list[index + 1] = new_list[index + 1] + '!' # 修改'short'后面的元素
# 输出更新后的列表
print("修改后的列表:", new_list)
```
运行上述代码,你会得到输出结果如下:
```
元素'short'的索引是 2
修改后的列表: ['lift', 'is', 'short!', 'You', 'need', 'python']
```
相关问题
python1、创建列表list1 = ['lift', 'is', 'short','You','need','python'],并完成以下任务:1)输出列表中元素‘short’及其索引下标;2)在
首先,我们可以使用Python的内置函数`index()`来获取元素'short'的索引位置。列表的索引是从0开始计数的。对于输出元素和它的索引,代码会像这样:
```python
# 创建列表 list1
list1 = ['lift', 'is', 'short', 'You', 'need', 'python']
# 输出元素 'short' 及其索引
element = 'short'
index = list1.index(element)
print(f"元素 '{element}' 的索引是 {index}")
# 如果需要同时输出元素和索引,可以使用下面这种方式
output = f"元素 '{element}' 在列表中的位置是 ({index}, '{element}')"
print(output)
```
接下来,如果你想要插入一个新的元素到特定索引,例如将'long'放在'short'之前,你可以这样做:
```python
new_element = 'long'
list1.insert(index, new_element) # 插入新元素到短的前面
print("更新后的列表:", list1)
```
如果你想删除某个元素,也可以通过索引来操作:
```python
list1.pop(index) # 删除'short',并返回它(这里不再显示删除后的完整列表)
```
Python创建列表list1 = ['lift', 'is', 'short','You','need','python'],并完成以下任务:1)输出列表中元素‘short’及其索引下标;2)在‘short’后面增加一个‘!’。输出结果如下:
首先,让我们创建这个名为`list1`的列表,它包含六个字符串元素:
```python
list1 = ['lift', 'is', 'short', 'You', 'need', 'python']
```
接下来,我们按照您的要求完成任务:
1) 输出`'short'`及其索引下标:
```python
index_of_short = list1.index('short')
print(f"'short' 的索引是 {index_of_short}")
```
这里我们使用`index()`方法找到`'short'`的位置,并将其打印出来。
2) 在`'short'`后面增加一个`'!'`:
```python
list1[index_of_short] = list1[index_of_short] + '!'
```
这行代码会直接修改列表中的元素。
现在,我们将上述两个步骤组合起来并打印最终的列表:
```python
index_of_short = list1.index('short')
list1[index_of_short] = list1[index_of_short] + '!'
print("修改后的列表:", list1)
```
执行这些代码后,你应该能看到输出如下所示:
```markdown
'short' 的索引是 2
修改后的列表: ['lift', 'is', 'short!', 'You', 'need', 'python']
```
阅读全文
相关推荐
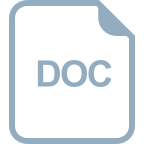
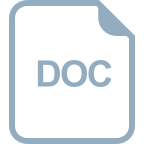
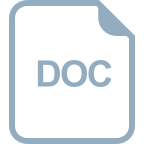


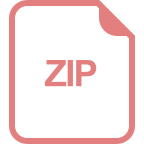
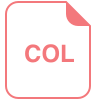










