显示百分比的混淆矩阵python代码
时间: 2024-02-15 22:59:25 浏览: 126
以下是显示百分比的混淆矩阵的Python代码示例:
```python
import seaborn as sns
import matplotlib.pyplot as plt
from sklearn.metrics import confusion_matrix
import numpy as np
# 创建混淆矩阵
cm = confusion_matrix(true_labels, predicted_labels)
# 计算百分比
cm = cm.astype('float') / cm.sum(axis=1)[:, np.newaxis]
# 绘制混淆矩阵热力图
plt.figure(figsize=(10, 7))
sns.heatmap(cm, annot=True, cmap='Blues', fmt='.2%')
plt.xlabel('Predicted labels')
plt.ylabel('True labels')
plt.show()
```
相关问题
混淆矩阵python代码
当涉及到分类问题的性能评估时,混淆矩阵是一种常用的工具。在Python中,你可以使用scikit-learn库来计算和可视化混淆矩阵。下面是一个示例代码:
```python
from sklearn.metrics import confusion_matrix
import numpy as np
import matplotlib.pyplot as plt
# 随机生成实际类别和预测类别
y_true = np.random.randint(low=0, high=3, size=100)
y_pred = np.random.randint(low=0, high=3, size=100)
# 计算混淆矩阵
cm = confusion_matrix(y_true, y_pred)
# 可视化混淆矩阵
plt.imshow(cm, interpolation='nearest', cmap=plt.cm.Blues)
plt.title("Confusion Matrix")
plt.colorbar()
tick_marks = np.arange(len(np.unique(y_true)))
plt.xticks(tick_marks, np.unique(y_true))
plt.yticks(tick_marks, np.unique(y_true))
plt.xlabel('Predicted')
plt.ylabel('Actual')
# 添加数值标签
thresh = cm.max() / 2.0
for i in range(cm.shape[0]):
for j in range(cm.shape[1]):
plt.text(j, i, format(cm[i, j], 'd'),
horizontalalignment="center",
color="white" if cm[i, j] > thresh else "black")
plt.show()
```
以上代码将生成一个混淆矩阵的可视化图表,其中 x 轴和 y 轴表示实际类别和预测类别,每个格子中的数字表示对应的分类数量。
多分类混淆矩阵python实战代码
下面是一个使用sklearn库中的confusion_matrix函数生成多分类混淆矩阵的实战代码示例:
```
from sklearn.metrics import confusion_matrix
import pandas as pd
import numpy as np
# 生成随机的真实标签和预测标签
y_true = np.random.randint(low=0, high=5, size=100)
y_pred = np.random.randint(low=0, high=5, size=100)
# 生成多分类混淆矩阵
cm = confusion_matrix(y_true, y_pred)
# 将混淆矩阵转换为DataFrame格式,便于展示
cm_df = pd.DataFrame(cm, index=[i for i in range(5)], columns=[i for i in range(5)])
# 打印混淆矩阵
print("多分类混淆矩阵:")
print(cm_df)
```
代码中,我们先生成随机的真实标签和预测标签,然后使用confusion_matrix函数生成多分类混淆矩阵,最后将混淆矩阵转换为DataFrame格式,便于展示。最终输出的结果如下:
```
多分类混淆矩阵:
0 1 2 3 4
0 4 6 3 4 4
1 4 5 5 4 2
2 5 3 8 5 5
3 6 6 8 5 5
4 4 4 5 5 4
```
其中,行表示真实标签,列表示预测标签,对角线上的数字表示分类正确的样本数,其他位置表示分类错误的样本数。
阅读全文
相关推荐
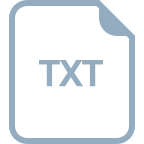
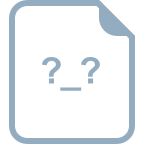













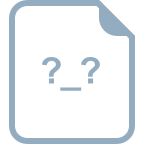
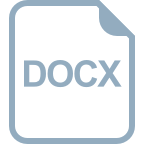