Java 执行一个功能函数,通过另外一个线程计算时间实现超时退出 详细代码
时间: 2024-04-16 20:27:33 浏览: 14
当需要执行一个功能函数,并通过另外一个线程计算时间来实现超时退出时,可以使用Java的多线程机制和`Future`接口来实现。下面是一个简单的示例代码:
```java
import java.util.concurrent.*;
public class TimeoutExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(1);
Callable<String> task = new Callable<String>() {
public String call() throws InterruptedException {
// 执行功能函数的代码
// 这里仅作示例,假设需要执行一个耗时的任务
Thread.sleep(3000);
return "Task completed";
}
};
Future<String> future = executor.submit(task);
try {
// 设置超时时间为2秒
String result = future.get(2, TimeUnit.SECONDS);
System.out.println(result);
} catch (InterruptedException e) {
System.out.println("Task interrupted");
} catch (ExecutionException e) {
System.out.println("Task encountered an exception: " + e.getCause());
} catch (TimeoutException e) {
System.out.println("Task timed out");
}
// 关闭线程池
executor.shutdownNow();
}
}
```
在上面的示例中,创建了一个线程池`executor`,然后定义了一个`Callable`接口的实现任务`task`,在`call()`方法中编写了要执行的功能函数的代码。然后通过`executor.submit(task)`提交任务并返回一个`Future`对象。
接下来,通过调用`future.get(timeout, unit)`方法来获取结果,在指定的超时时间内等待任务完成。如果任务在超时时间内完成,将会返回结果,否则将抛出`TimeoutException`。
最后,记得关闭线程池,释放资源。
请注意,这只是一个简单的示例,实际情况下你可能需要根据具体需求对代码进行适当修改。
相关推荐
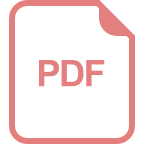
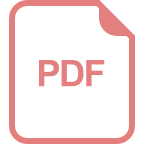














