用C语言编程实现: 已知线性表LA和LB
时间: 2024-09-10 17:18:44 浏览: 73
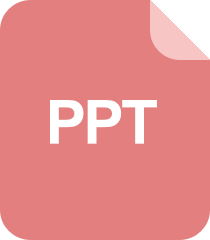
数据结构-C语言版:DS02-线性表.ppt
在C语言编程中,如果你想要操作线性表LA和LB,通常我们会使用数组或者结构体来表示线性表。这里我们假设线性表是通过动态内存分配的单链表来实现的,因为链表可以方便地处理元素插入和删除。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义线性表节点结构
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建一个新的节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 在线性表LA的末尾添加元素
void appendToList(Node** LA, int value) {
if (*LA == NULL) { // 如果列表为空
*LA = createNode(value);
} else {
Node* temp = *LA;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = createNode(value);
}
}
// 合并两个线性表LA和LB
void mergeLists(Node** LA, Node** LB) {
if (*LA == NULL) { // 如果LA为空,则直接将LB连接到LA
*LA = *LB;
} else if (*LB == NULL) { // 如果LB为空,则不做任何事情,LA已经足够了
} else if (*LA->data <= *LB->data) { // 如果LA的第一个元素小于等于LB的第一个元素
*LA->next = mergeLists(&(*LA)->next, LB); // 递归合并LA的剩余部分
} else {
*LB->next = mergeLists(LA, &(*LB)->next); // 否则,将LB的剩余部分连接到LA之后
}
return;
}
// 打印线性表
void printList(Node* node) {
while (node != NULL) {
printf("%d -> ", node->data);
node = node->next;
}
printf("NULL\n");
}
int main() {
Node* LA = NULL;
Node* LB = NULL;
// 初始化和填充LA和LB...
appendToList(&LA, 5);
appendToList(&LA, 10);
appendToList(&LB, 3);
appendToList(&LB, 8);
mergeLists(&LA, &LB);
printf("Merged List: ");
printList(LA);
return 0;
}
```
在这个例子中,我们首先创建了两个空的链表LA和LB,然后对它们进行了初始化和填充。接着,我们实现了合并功能,最后打印出合并后的线性表。
阅读全文
相关推荐
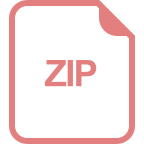
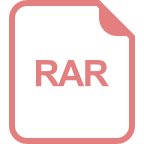






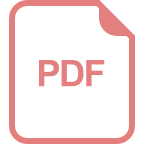
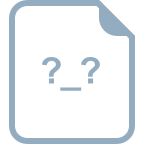
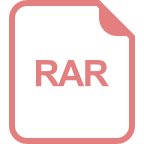

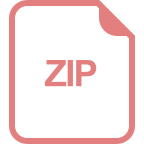
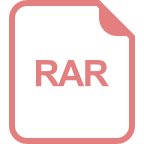