注:此题也可采用STL知识用next_permutation函数来做
时间: 2024-09-07 20:02:47 浏览: 15
在C++标准模板库(Standard Template Library, STL)中,`next_permutation`函数是一个非常实用的工具,它用于生成一个序列的所有可能的下一个排列。这个函数适用于可以进行排序操作的元素集合,比如整数、字符数组等。
`next_permutation`函数接收两个迭代器作为参数,分别指向待排列序列的第一个元素和最后一个元素之后的一个位置。它会找到当前排列后的下一个最大排列,如果找不到,则说明已经是最小排列,函数返回`false`;如果找到,就将序列调整到下一个排列,并返回`true`。
例如,对于一个已排序的升序数组,你可以先用`prev_permutation`得到当前的最大排列,然后立即调用`next_permutation`获取下一个小于当前排列的排列。这种方法常用于实现一些随机化算法或者需要所有排列的应用场景。
```cpp
#include <algorithm>
#include <vector>
std::vector<int> nums = {1, 2, 3};
if (std::next_permutation(nums.begin(), nums.end())) {
// 打印当前排列
for (int num : nums) {
std::cout << num << " ";
}
} else {
// 已经是最小排列,无需继续
}
```
相关问题
next_permutation函数
next_permutation函数是一个C++ STL函数,用于生成下一个排列。该函数的作用是在当前排列的基础上生成下一个字典序更大的排列。如果当前排列是最大的排列,则该函数会将排列重置为最小的排列。
下面是一个示例程序,演示了如何使用next_permutation函数来生成排列:
```
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main()
{
vector<int> v = {1, 2, 3, 4}; // 初始排列
do {
for (int i = 0; i < v.size(); i++) {
cout << v[i] << " ";
}
cout << endl;
} while (next_permutation(v.begin(), v.end()));
return 0;
}
```
在上面的程序中,我们首先定义了一个初始排列,然后使用do-while循环来迭代生成所有可能的排列。在每次迭代中,我们使用next_permutation函数来生成下一个排列,并输出该排列。当next_permutation函数返回false时,表示已经生成了所有排列,退出循环。
需要注意的是,next_permutation函数只能生成字典序更大的排列,如果需要生成字典序更小的排列,可以使用prev_permutation函数。
c++next_permutation函数
### 回答1:
C++中的next_permutation函数是一个STL算法,用于生成下一个排列。它接受两个迭代器作为参数,表示一个范围内的元素。函数会将这个范围内的元素重新排列,生成下一个排列,并返回true。如果已经是最后一个排列,则返回false。
### 回答2:
C++标准库中的next_permutation函数用于生成一个序列中下一个排列的序列。
该函数在一个非递减排列的序列中,找到下一个比当前排列更大的排列,并对原序列进行重排,如果不存在下一个更大的排列,则返回false。重排完成后,原序列将变为下一个更大的排列。
next_permutation函数接收两个迭代器,表示序列的开始和结束位置。使用该函数时,要求序列必须是允许重复元素的。函数会根据序列中的元素值进行重排。
函数的使用步骤如下:
1. 首先需要包含<algorithm>头文件。
2. 调用next_permutation函数并传入序列的开始和结束迭代器。
3. 函数会返回一个布尔值,表示是否存在下一个更大的排列。
4. 如果返回true,表示存在下一个更大的排列,可以通过迭代器遍历并打印出来。
5. 如果返回false,表示已经是最大排列,序列不再改变。
下面是一个示例代码段,展示了如何使用next_permutation函数:
```cpp
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3};
// 打印初始排列
for (int num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
// 获取下一个更大的排列
while (std::next_permutation(nums.begin(), nums.end())) {
// 打印下一个更大的排列
for (int num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
}
return 0;
}
```
运行以上代码,会输出以下结果:
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
可以看到,函数通过改变序列中元素的排列顺序,依次生成了下一个更大的排列。
### 回答3:
next_permutation函数是C++中的一个算法函数,用于获取给定排列的下一个排列。该函数接受两个迭代器作为参数,并在原地将排列重新排列为下一个排列。如果存在下一个排列,则该函数返回true,否则返回false。
next_permutation函数的算法原理是,从后往前找到第一个非递增的元素,记为i。然后再从后往前找到第一个大于i的元素,记为j。将i和j交换位置,并将i之后的元素按照升序重新排列,即得到下一个排列。
下面以一个例子来说明next_permutation函数的使用。假设有一个排列{1, 2, 3},我们使用next_permutation函数来获取所有的排列。
首先,初始排列是{1, 2, 3},然后调用next_permutation函数,它会返回true,并将排列修改为{1, 3, 2}。接着再次调用next_permutation函数,会得到{2, 1, 3},再次调用则得到{2, 3, 1},继续调用则得到{3, 1, 2},最后再次调用则得到{3, 2, 1},此时函数返回false,表示已经获取到了所有的排列。
总结来说,next_permutation函数是用于获取给定排列的下一个排列的函数,通过不断调用该函数,可以获取排列集合中的所有排列。
相关推荐
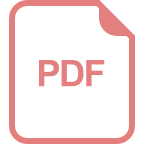
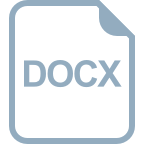
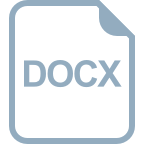
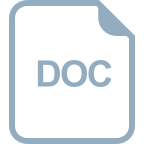
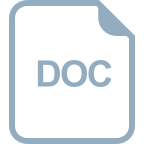
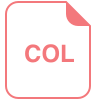
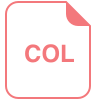

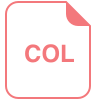
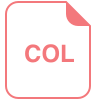





