memcpy(buff_.data() + writer_, data, len)
时间: 2024-09-09 11:15:15 浏览: 63
`memcpy` 是 C++ 中的一个函数,用于将一段内存内容复制到另一段内存。具体来说,`memcpy` 函数的原型定义在头文件 `<cstring>` 中,如下所示:
```cpp
void* memcpy(void* dest, const void* src, size_t count);
```
- `dest`:目标内存区域的指针,要将数据复制到这个位置。
- `src`:源内存区域的指针,要从这个位置复制数据。
- `count`:要复制的字节数。
在你给出的代码 `memcpy(buff_.data() + writer_, data, len)` 中,执行的操作是将 `data` 指向的内存区域中的 `len` 个字节复制到 `buff_` 指向的内存区域中,复制的起始位置是从 `buff_` 的当前写入位置 `writer_` 开始的偏移位置。
这里 `buff_.data()` 返回 `buff_` 所管理的内存的指针(通常是一个 `std::vector` 或类似容器),而 `+ writer_` 是对这个指针进行偏移操作,确保数据从正确的位置开始被复制。
这是一个常见的操作,用于在不创建新对象的情况下,将数据复制到容器中某个特定位置,比如用于实现快速的数据插入或更新。
相关问题
static int aciga_action_in_cmd_common(unsigned short cmd,uint8_t msgid, uint32_t runid,uint8_t *para,int para_len,uint8_t *out,int *out_len) { // ACIGA_LOGD("%s",__func__); int msg_len=0; out[msg_len++]=(cmd>>8)&0xff; out[msg_len++]=(cmd)&0xff; out[msg_len++] = msgid; //msg_id out[msg_len++] = (runid>>24)&0xff; out[msg_len++] = (runid>>16)&0xff; out[msg_len++] = (runid>>8)&0xff; out[msg_len++]= (runid)&0xff; if(para_len) { memcpy(&out[msg_len],para,para_len); msg_len =msg_len+para_len; } *out_len = msg_len; return 0; }
这段代码是一个静态函数,函数名为`aciga_action_in_cmd_common`,其功能为将输入的参数打包成一段二进制数据,用于发送给某个外部设备或进行网络传输。具体而言,该函数接受以下参数:
- `cmd`:unsigned short类型的命令码,用于标识需要执行的命令。
- `msgid`:uint8_t类型的消息ID,用于标识消息。
- `runid`:uint32_t类型的运行ID,用于标识运行的ID。
- `para`:uint8_t类型的参数数组,用于存储需要发送的参数。
- `para_len`:int类型的参数长度,表示参数数组的长度。
- `out`:uint8_t类型的输出数组,用于存储打包后的二进制数据。
- `out_len`:int类型的输出长度指针,表示打包后的数据长度。
函数首先将命令码、消息ID和运行ID打包到输出数组中,接着将参数数组拷贝到输出数组的后面,最后将输出数组的长度赋值给输出长度指针,并返回0表示执行成功。
osal_memcpy(serial_data.data0,tempvalue,4);
`osal_memcpy` 函数是操作系统抽象层(Operating System Abstraction Layer,OSAL)中的一个内存复制函数,它用于将一块内存中的内容复制到另一块内存中。函数原型通常如下:
```c
void osal_memcpy(void *dest, const void *src, size_t n);
```
这个函数接受三个参数:
1. `dest`:目标内存地址,是一个指向 void 类型的指针,即可以指向任何类型的数据。
2. `src`:源内存地址,也是一个指向 void 类型的指针,表示数据来源。
3. `n`:要复制的字节数,指定从源地址复制多少字节到目标地址。
在你给出的示例代码 `osal_memcpy(serial_data.data0,tempvalue,4);` 中,它的含义是将 `tempvalue` 指向的内存中的4个字节复制到 `serial_data.data0` 指向的内存地址开始的位置。
需要注意的是,使用 `osal_memcpy` 或者类似的内存复制函数时,必须确保目标内存区域足够大,以便能够容纳即将复制过来的数据,否则可能会导致内存越界,从而引发不可预知的错误。
相关推荐
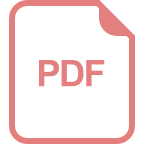
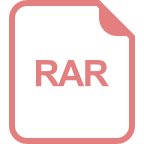
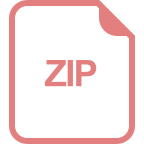












